Question
MIPS Programming Exercise Write a recursive function using the real-world linkage convention using a stack frame to implement the Fibonacci function, with all parameters passed
MIPS Programming Exercise
Write a recursive function using the "real-world linkage convention" using a stack frame to implement the Fibonacci function, with all parameters passed on the stack, not via registers. Allocate all variables (even for main's variables) on the stack, not in the data segment. For this exercise, the Fibonacci series is 0, 1, 1, 2, 3, 5, 8 ... and the elements are numbered from 0, so fib(6) = 8. Your pseudocode for this function will be like this. The parameter n must be held on the stack, not in a register: The variables x and y must also be local variables allocated on the stack. int Fib( int n )
{
if( n <= 0 )
return 0
endif
if( n == 1 )
return 1
endif
x = Fib( n-1 )
y = Fib( n-2 )
return x + y
}
The return value will be in $v0. You might want to start this by first writing it using a register for the argument, not the stack, and then change it to use the stack. Test up to Fib(10).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
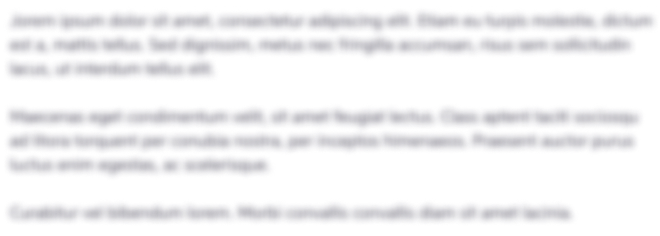
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started