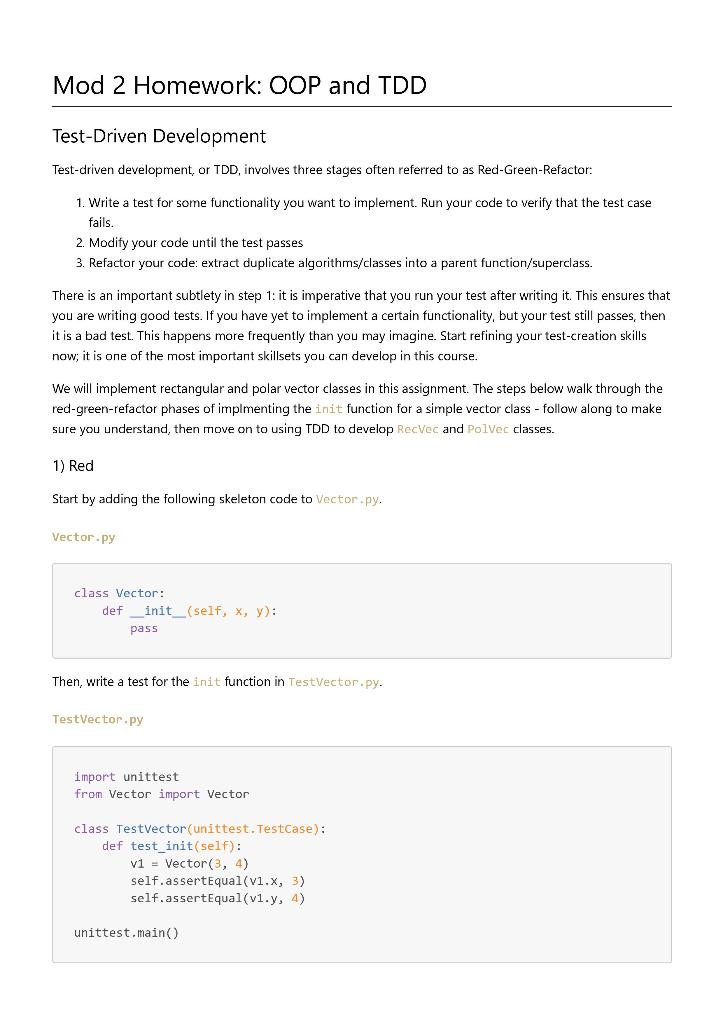
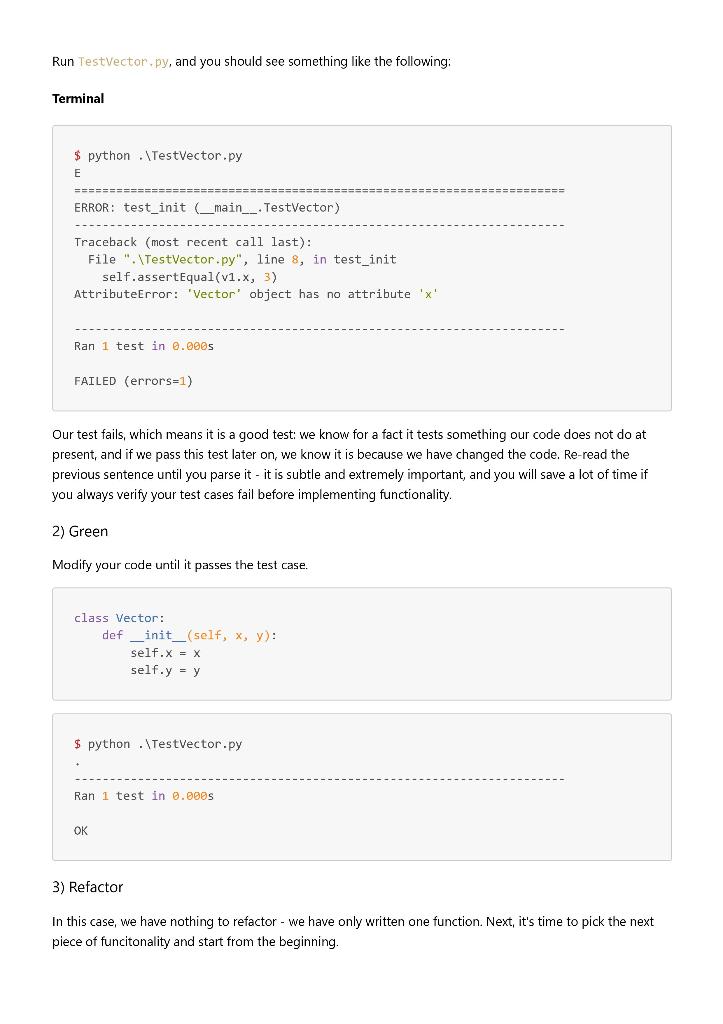
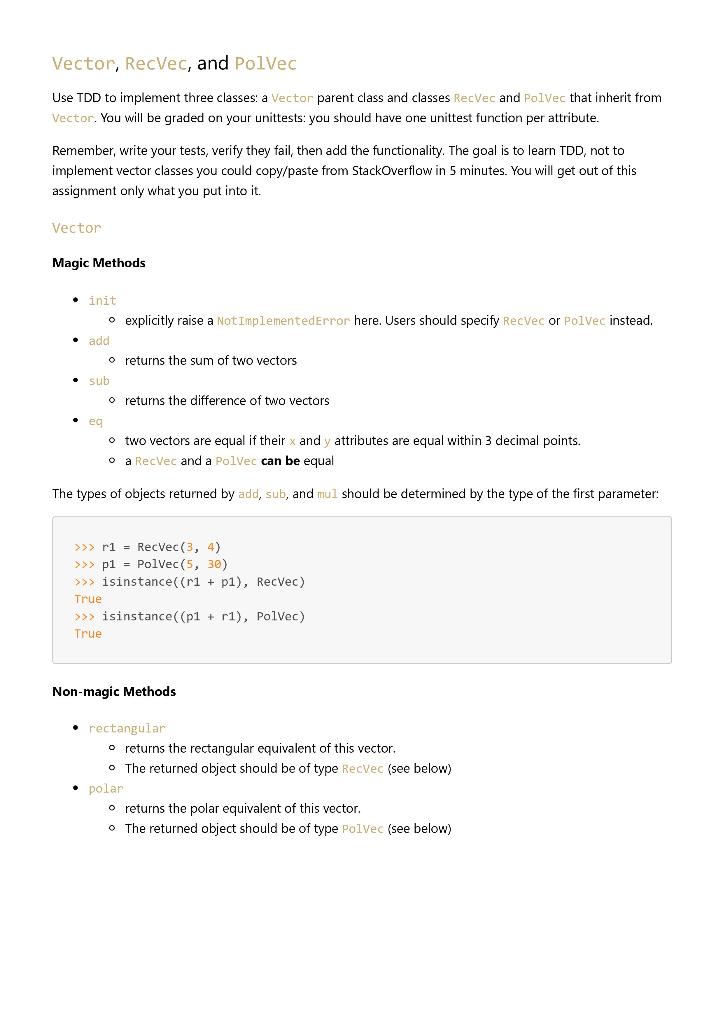
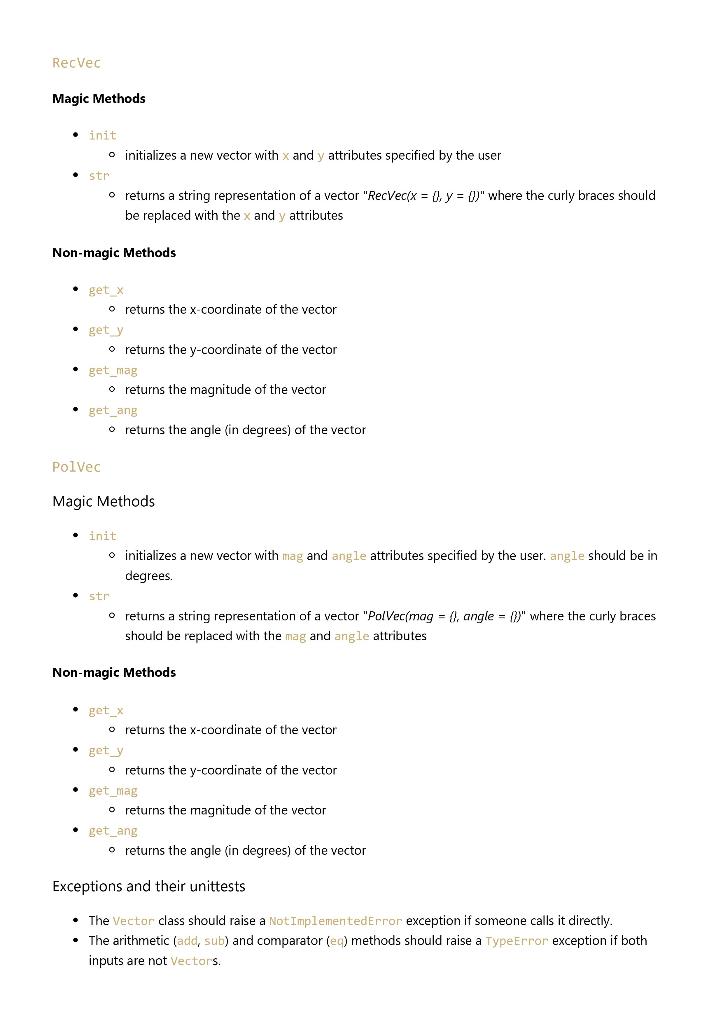
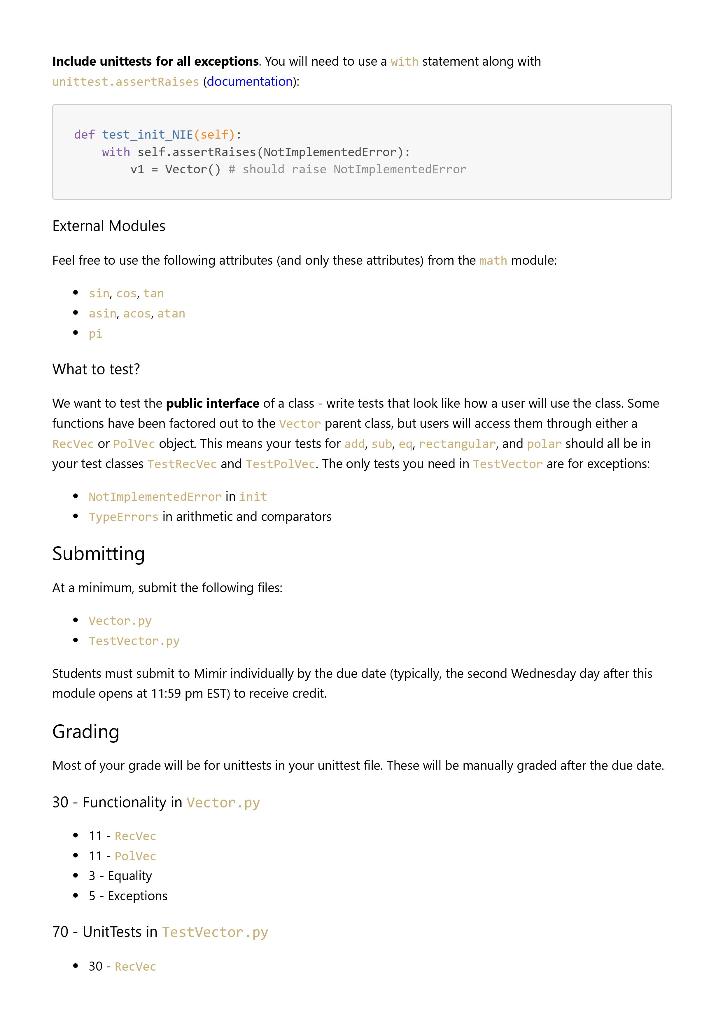
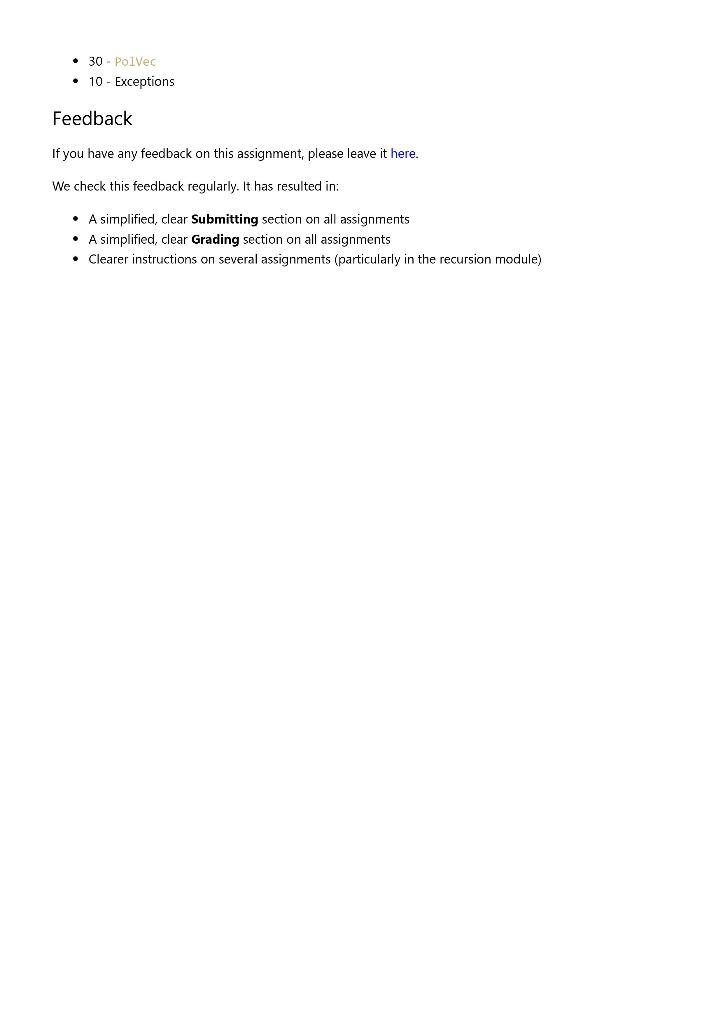
Mod 2 Homework: OOP and TDD Test-Driven Development Test-driven development, or TDD, involves three stages often referred to as Red-Green-Refactor: 1. Write a test for some functionality you want to implement. Run your code to verify that the test case fails. 2. Modify your code until the test passes 3. Refactor your code: extract duplicate algorithms/classes into a parent function/superclass. There is an important subtlety in step 1: it is imperative that you run your test after writing it. This ensures that you are writing good tests. If you have yet to implement a certain functionality, but your test still passes, then it is a bad test. This happens more frequently than you may imagine. Start refining your test-creation skills now, it is one of the most important skillsets you can develop in this course. We will implement rectangular and polar vector classes in this assignment. The steps below walk through the red-green-refactor phases of implmenting the init function for a simple vector class - follow along to make sure you understand, then move on to using TDD to develop Recvec and Polvec classes. 1) Red Start by adding the following skeleton code to Vector.py. Vector.py class Vector: def _init__(self, x, y): pass Then, write a test for the init function in TestVector.py. Test Vector.py import unittest from Vector import Vector class Testvector(unittest. Testcase): def test_init(self): v1 = Vector(3, 4) self.assertEqual(v1.X, 3) self.assertEqual(v1.y, 4) unittest.main() Run TestVector.py, and you should see something like the following: Terminal $ python . \TestVector.py E ERROR: test_init (_main__.TestVector) Traceback (most recent call last): File ". \TestVector.py", line 8, in test_init self.assertEqual(v1.x, 3) AttributeError: 'Vector' object has no attribute 'x' Ran 1 test in @.0005 FAILED (errors=1) Our test fails, which means it is a good test: we know for a fact it tests something our code does not do at present, and if we pass this test later on, we know it is because we have changed the code. Re-read the previous sentence until you parse it - it is subtle and extremely important, and you will save a lot of time if you always verify your test cases fail before implementing functionality. 2) Green Modify your code until it passes the test case. class Vector: def _init__(self, x, y): self.x = x self.y = y $ python . \TestVector.py Ran 1 test in 0.0005 OK 3) Refactor In this case, we have nothing to refactor - we have only written one function. Next, it's time to pick the next piece of funcitonality and start from the beginning. Vector, RecVec, and Polvec Use TDD to implement three classes: a Vector parent class and classes Recvec and Polved that inherit from Vector. You will be graded on your unittests: you should have one unittest function per attribute. Remember, write your tests, verify they fail, then add the functionality. The goal is to learn TDD, not to implement vector classes you could copy/paste from Stackoverflow in 5 minutes. You will get out of this assignment only what you put into it Vector Magic Methods init explicitly raise a Not ImplementedError here. Users should specify Recvec or Polved instead. add o returns the sum of two vectors sub o returns the difference of two vectors . eg o two vectors are equal if their x and y attributes are equal within 3 decimal points. o a Recvec and a PolVec can be equal The types of objects returned by add, sub, and mul should be determined by the type of the first parameter: >>> r1 = Recvec(3, 4) >>> pl = Polvec (5, 30) >>> isinstance((r1 + p1), Recvec) True >>> isinstance((p1 + r1), PolVec) True Non-magic Methods rectangular o returns the rectangular equivalent of this vector. The returned object should be of type Recvec (see below) polar o returns the polar equivalent of this vector, The returned object should be of type Polvec (see below) Recvec Magic Methods init o initializes a new vector with x and y attributes specified by the user Str o returns a string representation of a vector "Recvec(x = , y = 1))"where the curly braces should be replaced with the x and y attributes Non-magic Methods get o returns the x-coordinate of the vector gety returns the y-coordinate of the vector get_mag o returns the magnitude of the vector get_ang o returns the angle (in degrees) of the vector PolVec Magic Methods init initializes a new vector with mag and angle attributes specified by the user angle should be in degrees st o returns a string representation of a vector "PolVec(mag = 1), angle = [])" where the curly braces should be replaced with the mag and angle attributes Non-magic Methods get_ o returns the x-coordinate of the vector gety o returns the y-coordinate of the vector get_mag o returns the magnitude of the vector get_ang o returns the angle in degrees) of the vector Exceptions and their unittests The Vector class should raise a NotImplementedError exception if someone calls it directly. The arithmetic (add, sub) and comparator (e) methods should raise a TypeError exception if both inputs are not Vectors. Include unittests for all exceptions. You will need to use a with statement along with unittest.assertRaises (documentation): def test_init_NIE(self): with self.assertRaises (Not ImplementedError): v1 = Vector() # should raise NotImplementedError External Modules Feel free to use the following attributes (and only these attributes) from the math module: sin, cos, tan asin, acos, atan pi What to test? We want to test the public interface of a class write tests that look like how a user will use the class. Some functions have been factored out to the vector parent class, but users will access them through either a Recvec or Polvec object. This means your tests for add, sub, egr rectangular, and polar should all be in your test classes TestRecvec and Test Polvec. The only tests you need in TestVector are for exceptions: Not implementedError in init TypeErrors in arithmetic and comparators Submitting At a minimum, submit the following files: Vector.py TestVector.py Students must submit to Mimir individually by the due date (typically, the second Wednesday day after this module opens at 11:59 pm EST) to receive credit. Grading Most of your grade will be for unittests in your unittest file. These will be manually graded after the due date. 30 - Functionality in Vector.py . 11 - Recvec 11 - PolVec 3 - Equality 5 - Exceptions 70 - Unit Tests in TestVector.py 30 - Recvec 30. PolVec 10 - Exceptions Feedback If you have any feedback on this assignment, please leave it here. We check this feedback regularly. It has resulted in: A simplified, clear Submitting section on all assignments A simplified, clear Grading section on all assignments Clearer instructions on several assignments (particularly in the recursion module) Mod 2 Homework: OOP and TDD Test-Driven Development Test-driven development, or TDD, involves three stages often referred to as Red-Green-Refactor: 1. Write a test for some functionality you want to implement. Run your code to verify that the test case fails. 2. Modify your code until the test passes 3. Refactor your code: extract duplicate algorithms/classes into a parent function/superclass. There is an important subtlety in step 1: it is imperative that you run your test after writing it. This ensures that you are writing good tests. If you have yet to implement a certain functionality, but your test still passes, then it is a bad test. This happens more frequently than you may imagine. Start refining your test-creation skills now, it is one of the most important skillsets you can develop in this course. We will implement rectangular and polar vector classes in this assignment. The steps below walk through the red-green-refactor phases of implmenting the init function for a simple vector class - follow along to make sure you understand, then move on to using TDD to develop Recvec and Polvec classes. 1) Red Start by adding the following skeleton code to Vector.py. Vector.py class Vector: def _init__(self, x, y): pass Then, write a test for the init function in TestVector.py. Test Vector.py import unittest from Vector import Vector class Testvector(unittest. Testcase): def test_init(self): v1 = Vector(3, 4) self.assertEqual(v1.X, 3) self.assertEqual(v1.y, 4) unittest.main() Run TestVector.py, and you should see something like the following: Terminal $ python . \TestVector.py E ERROR: test_init (_main__.TestVector) Traceback (most recent call last): File ". \TestVector.py", line 8, in test_init self.assertEqual(v1.x, 3) AttributeError: 'Vector' object has no attribute 'x' Ran 1 test in @.0005 FAILED (errors=1) Our test fails, which means it is a good test: we know for a fact it tests something our code does not do at present, and if we pass this test later on, we know it is because we have changed the code. Re-read the previous sentence until you parse it - it is subtle and extremely important, and you will save a lot of time if you always verify your test cases fail before implementing functionality. 2) Green Modify your code until it passes the test case. class Vector: def _init__(self, x, y): self.x = x self.y = y $ python . \TestVector.py Ran 1 test in 0.0005 OK 3) Refactor In this case, we have nothing to refactor - we have only written one function. Next, it's time to pick the next piece of funcitonality and start from the beginning. Vector, RecVec, and Polvec Use TDD to implement three classes: a Vector parent class and classes Recvec and Polved that inherit from Vector. You will be graded on your unittests: you should have one unittest function per attribute. Remember, write your tests, verify they fail, then add the functionality. The goal is to learn TDD, not to implement vector classes you could copy/paste from Stackoverflow in 5 minutes. You will get out of this assignment only what you put into it Vector Magic Methods init explicitly raise a Not ImplementedError here. Users should specify Recvec or Polved instead. add o returns the sum of two vectors sub o returns the difference of two vectors . eg o two vectors are equal if their x and y attributes are equal within 3 decimal points. o a Recvec and a PolVec can be equal The types of objects returned by add, sub, and mul should be determined by the type of the first parameter: >>> r1 = Recvec(3, 4) >>> pl = Polvec (5, 30) >>> isinstance((r1 + p1), Recvec) True >>> isinstance((p1 + r1), PolVec) True Non-magic Methods rectangular o returns the rectangular equivalent of this vector. The returned object should be of type Recvec (see below) polar o returns the polar equivalent of this vector, The returned object should be of type Polvec (see below) Recvec Magic Methods init o initializes a new vector with x and y attributes specified by the user Str o returns a string representation of a vector "Recvec(x = , y = 1))"where the curly braces should be replaced with the x and y attributes Non-magic Methods get o returns the x-coordinate of the vector gety returns the y-coordinate of the vector get_mag o returns the magnitude of the vector get_ang o returns the angle (in degrees) of the vector PolVec Magic Methods init initializes a new vector with mag and angle attributes specified by the user angle should be in degrees st o returns a string representation of a vector "PolVec(mag = 1), angle = [])" where the curly braces should be replaced with the mag and angle attributes Non-magic Methods get_ o returns the x-coordinate of the vector gety o returns the y-coordinate of the vector get_mag o returns the magnitude of the vector get_ang o returns the angle in degrees) of the vector Exceptions and their unittests The Vector class should raise a NotImplementedError exception if someone calls it directly. The arithmetic (add, sub) and comparator (e) methods should raise a TypeError exception if both inputs are not Vectors. Include unittests for all exceptions. You will need to use a with statement along with unittest.assertRaises (documentation): def test_init_NIE(self): with self.assertRaises (Not ImplementedError): v1 = Vector() # should raise NotImplementedError External Modules Feel free to use the following attributes (and only these attributes) from the math module: sin, cos, tan asin, acos, atan pi What to test? We want to test the public interface of a class write tests that look like how a user will use the class. Some functions have been factored out to the vector parent class, but users will access them through either a Recvec or Polvec object. This means your tests for add, sub, egr rectangular, and polar should all be in your test classes TestRecvec and Test Polvec. The only tests you need in TestVector are for exceptions: Not implementedError in init TypeErrors in arithmetic and comparators Submitting At a minimum, submit the following files: Vector.py TestVector.py Students must submit to Mimir individually by the due date (typically, the second Wednesday day after this module opens at 11:59 pm EST) to receive credit. Grading Most of your grade will be for unittests in your unittest file. These will be manually graded after the due date. 30 - Functionality in Vector.py . 11 - Recvec 11 - PolVec 3 - Equality 5 - Exceptions 70 - Unit Tests in TestVector.py 30 - Recvec 30. PolVec 10 - Exceptions Feedback If you have any feedback on this assignment, please leave it here. We check this feedback regularly. It has resulted in: A simplified, clear Submitting section on all assignments A simplified, clear Grading section on all assignments Clearer instructions on several assignments (particularly in the recursion module)