Question
Modify following program to create N*L threads, each computing ith row multiplied by jth column. #include #include #include #define N 4 //Number of threads //Global
Modify following program to create N*L threads, each computing ith row multiplied by jth column.
#include
#include
#include
#define N 4 //Number of threads
//Global matrices A, B, C
double **matrixA, **matrixB, **matrixC;
int M, L;
//Thread function for multiplying the ith row of matrixA by matrixB
void *multiplyRow(void *arg) {
int i = (int)(size_t)arg;
for (int j = 0; j < L; j++) {
matrixC[i][j] = 0;
for (int k = 0; k < M; k++) {
matrixC[i][j] += matrixA[i][k] * matrixB[k][j];
}
}
return NULL;
}
//Initialize matrix with random values
void initializeMatrix(int r, int c, double **matrix) {
for (int i = 0; i < r; i++) {
for (int j = 0; j < c; j++) {
matrix[i][j] = (double)rand() / RAND_MAX;
}
}
}
//Print matrix
void printMatrix(int r, int c, double **matrix) {
for (int i = 0; i < r; i++) {
for (int j = 0; j < c; j++) {
printf("%f ", matrix[i][j]);
}
printf(" ");
}
}
int main(int argc, char *argv[]) {
if (argc < 3) {
printf("Usage: %s M L ", argv[0]);
return 1;
}
M = atoi(argv[1]);
L = atoi(argv[2]);
//Allocate memory for matrices A, B, and C
matrixA = (double**)malloc(N * sizeof(double*));
matrixB = (double**)malloc(M * sizeof(double*));
matrixC = (double**)malloc(N * sizeof(double*));
for (int i = 0; i < N; i++) {
matrixA[i] = (double*)malloc(M * sizeof(double));
matrixC[i] = (double*)malloc(L * sizeof(double));
}
for (int i = 0; i < M; i++) {
matrixB[i] = (double*)malloc(L * sizeof(double));
}
//Initialize matrices A and B with random values
initializeMatrix(N, M, matrixA);
initializeMatrix(M, L, matrixB);
//Create N threads, each multiplying ith row of matrixA by matrixB
for (int i = 0; i < N; i++) {
pthread_create(&threads[i], NULL, multiplyRow, (void*)(size_t)i);
}
//Wait for all threads to finish
for (int i = 0; i < N; i++) {
pthread_join(threads[i], NULL);
}
//Print matrices A, B, and C
printf("Matrix A: ");
printMatrix(N, M, matrixA);
printf("Matrix B: ");
printMatrix(M, L, matrixB);
printf("Matrix C: ");
printMatrix(N, L, matrixC);
//Free memory
for (int i = 0; i < N; i++) {
free(matrixA[i]);
free(matrixC[i]);
}
for (int i = 0; i < M; i++) {
free(matrixB[i]);
}
free(matrixA);
free(matrixB);
free(matrixC);
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
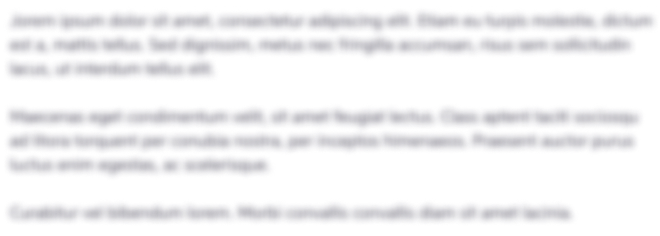
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started