Question
Modify project #4 by using modular programming principle: Divide the main function written for the project #4 into nine functions which are explained below. The
Modify project #4 by using modular programming principle: Divide the main function written for the project #4 into nine functions which are explained below. The number of students (n) will be declared global. Also, use global constants for the size of arrays. The numeric arrays and non-numeric array must be passed through parameters/arguments. Note that arrays are passed by reference only. The main function will be placed first. Therefore, you will need to declare prototypes of functions. Use your own students data and names of arrays. The description of functions is given below: Function main: It will include proper declarations. It will read the value of n (the number of students) from the key board and validate using a while loop (The value of n must be at least 3). It will call other functions as needed. Function inputData: This function will read the data from the input file into proper arrays and then call ValidateData to validate. Functions validateData: These will be two overloaded functions with same name. One function will validate non-numeric data and the other will validate numeric data. Function NumGrade: This function will compute and store into proper array elements the numerical grades. Function LetGrade: This function will compute and store into proper array elements the letter grades. Function Comments: This function will determine and write commend/warning comments. Function Report: After all computations are completed. Arrays will contain all the information. Pass these arrays to this function which will write to the output file the reports of all students.
here is my code for project 5
#include #include #include
using namespace std; //Define Arrays string nonNumeric[3][9]; int numeric[3][2]; double numeric2[3][3][6]; char letterGrade[3][3]; int students; //Define Floating point variables. const double A_Score = 90; const double B_Score = 80; const double C_Score = 70; const double D_Score = 60; //open files. ifstream file; ofstream fout; int inputData(); void outputData(); void validateData(string); void validateData(int); void letGrade(double); void writetoFile();
void letGrade(double numeric2[3][3][6]) { for(int j = 0; j < 3; j++) { //loop for courses for(int k = 0; k < 3; k++) { getline(file, nonNumeric[j][6+k]); if(nonNumeric[j][6].length() > 11) { fout << "Course number was too long, skipping to next class!" << endl; continue; } numeric2[j][k][5] = 0; double percent = .1; //loop fr tests for(int l = 0; l < 5; l++) { file >> numeric2[2][k][l]; if(numeric2[j][k][l] < 1.00 || numeric2[j][k][l] > 100.00) { fout << "Test score was not in valid range" << endl; break; } file.ignore(); //calculate grade numeric2[j][k][5] = numeric2[j][k][5] + (numeric2[j][k][l] * percent); percent += .05; } if(numeric2[j][k][5] >= A_Score) { letterGrade[j][k] = 'A'; } else if(numeric2[j][k][5] >= B_Score) { letterGrade[j][k] = 'B'; } else if(numeric2[j][k][5] >= C_Score) { letterGrade[j][k] = 'C'; } else if(numeric2[j][k][5] >= D_Score) { letterGrade[j][k] = 'D'; } else { letterGrade[j][k] = 'F'; } } } }
void validateData(string nonNumeric[3][9]) { for(int j = 0; j < 3; j++) { nonNumeric[j][0] = "Students Grade Sheet, Department of Computer Science, Texas State University"; getline(file, nonNumeric[j][1]); //checking whether the students name is within a reasonable range. if(nonNumeric[j][1].length() > 256) { fout << "Student Name was too long, skipping to next student!" << endl; continue; }
getline(file, nonNumeric[j][2]); //checking whether the students address is in reasonable range. if(nonNumeric[j][2].length() > 256) { fout << "Student address was too long, skipping to next student!" << endl; continue; }
getline(file, nonNumeric[j][3]); if(nonNumeric[j][3].length() > 10) { fout << "Student ID was not in the valid range" << endl; break; } getline(file, nonNumeric[j][4]); //used 16 to allow different country telephone numbers //checking whether the telephone number is within a reasonable range. if(nonNumeric[j][4].length() > 16) { fout << "Student telephone number was too long, skipping to next student!" << endl; continue; } getline(file, nonNumeric[j][5]); //11 is the max social number. //checking whether the social is within a reasonable range. if(nonNumeric[j][5].length() > 11) { fout << "Student social was too long, skipping to next student!" << endl; continue; }
} }
void validateData(int numberic[3][2]) {
for(int j = 0; j < 3; j++) { file >> numeric[j][0]; //checking whether age is between 1 and 90 if(numeric[j][0] > 90 || numeric[j][0] < 1) { fout << "Age was not in the valid range" << endl; break; }
file >> numeric[j][1]; //checking whether number of years is between 1 and 90 if(numeric[j][1] > 90 || numeric[j][1] < 1) { fout << "Number of years at Texas state was not in the valid range" << endl; break; }
} }
int inputData() {
file.open("Project5_input.txt"); if(!file) { cout << "Error in opening file: Project4_input.txt" << endl; return -1; } return 0; }
void outputData() { fout.open("Project5_Output.txt"); }
void writetoFile() { for(int i = 0; i <3; i++) { fout << setw(32) << nonNumeric[i][0] << endl; fout << setw(32) << "Name of Student:" << "\t" << nonNumeric[i][1] << endl; fout << setw(32) << "Student ID:" << "\t" << nonNumeric[i][3] << endl; fout << setw(32) << "Age:" << "\t" << numeric[i][0] << endl; fout << setw(32) << "Address:" << "\t" << nonNumeric[i][2] << endl; fout << setw(32) << "Number of years at Texas State:" << "\t" << numeric[i][1] << endl; fout << setw(32) << "Telephone Number:" << "\t" << nonNumeric[i][4] << endl; fout << setw(32) << "Student Social Security #:" << "\t" << nonNumeric[i][5] << endl << endl; for(int k = 0; k < 3; k++) { fout << setw(32) << "Course#:" << "\t" << nonNumeric[i][6+k] << endl; for(int j = 0; j < 5; j++) { fout << setw(30) << "Test#" << j + 1 << ":" << "\t" << numeric2[i][k][j] << endl; } fout << fixed << setw(32) << "Numerical Grade:" << "\t" << numeric2[i][k][5] << endl; fout << setw(32) << "Letter Grade:" << "\t" << letterGrade[i][k] << endl;
if(numeric2[i][k][5] >= A_Score) { fout << "Comment Note Congratulations! You have an A"; } else if(numeric2[i][k][5] < D_Score) { fout << "Warning Note: You Should Study! You are below a 70 grade average!"; } fout << endl << endl;
} } file.close(); fout.close(); }
int main() {
//get number of students cout << "Enter the number of Students: "; cin >> students; //loop for student information while(students >= 6 || students <= 0) { cout << "Incorrect range of students, please enter a number between 1 - 5" << endl; cin >> students; } inputData(); outputData(); validateData(nonNumeric); validateData(numeric); letGrade(numeric2); writetoFile(); return 0; }
when using the input file, it does not read from the input file correctly and does not pass the values into their correct slots when the file is outputed into a new txt file. Im not sure what is wrong with my code any advice will help thanks.
the format for the input for is like so
Mikr Fuchs
21
211 Teal Dr
3
A0134934
(713)-489-8950
492-35-5984
2302
60.5
67.5
92.6
78.6
82.6
1428
69.5
89.4
92.6
74.6
82.6
3339
97.4
67.5
92.6
74.6
82.6
The format is the same for the other two students
name
age
address
year at school
id
phone number
social security
course
Five grades
course
Five Grades
course
Five Grades
Step by Step Solution
There are 3 Steps involved in it
Step: 1
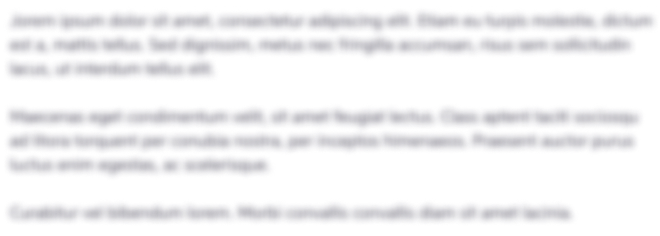
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started