Question
Modify Project #5 by replacing all arrays by one array of structures. You will use nested structures. Array should be passed to functions through Parameters/arguments.
Modify Project #5 by replacing all arrays by one array of structures. You will use nested structures. Array should be passed to functions through Parameters/arguments.
Project 5 code
#include
void letGrade(double numeric2[3][3][6]) { for(int j = 0; j < 3; j++) { //loop for courses for(int k = 0; k < 3; k++) { getline(file, nonNumeric[j][6+k]); if(nonNumeric[j][6].length() > 11) { fout << "Course number was too long, skipping to next class!" << endl; continue; } numeric2[j][k][5] = 0; double percent = .1; //loop five tests for(int l = 0; l < 5; l++) { file >> numeric2[j][k][l]; if(numeric2[j][k][l] < 1.00 || numeric2[j][k][l] > 100.00) { fout << "Test score was not in valid range" << endl; break; } file.ignore(); //calculate grade numeric2[j][k][5] = numeric2[j][k][5] + (numeric2[j][k][l] * percent); percent += .05; } if(numeric2[j][k][5] >= A_Score) { letterGrade[j][k] = 'A'; } else if(numeric2[j][k][5] >= B_Score) { letterGrade[j][k] = 'B'; } else if(numeric2[j][k][5] >= C_Score) { letterGrade[j][k] = 'C'; } else if(numeric2[j][k][5] >= D_Score) { letterGrade[j][k] = 'D'; } else { letterGrade[j][k] = 'F'; } } } }
void validateData(string nonNumeric[3][9]) { for(int j = 0; j < 3; j++) { //Heading for the Output File. nonNumeric[j][0] = "Students Grade Sheet, Department of Computer Science, Texas State University"; getline(file, nonNumeric[j][1]); //checking whether the students name is within a reasonable range. if(nonNumeric[j][1].length() > 256) { fout << "Student Name was too long, skipping to next student!" << endl; continue; }
getline(file, nonNumeric[j][2]); //checking whether the students address is in reasonable range. if(nonNumeric[j][2].length() > 256) { fout << "Student address was too long, skipping to next student!" << endl; continue; }
getline(file, nonNumeric[j][3]); if(nonNumeric[j][3].length() > 10) { fout << "Student ID was not in the valid range" << endl; break; } getline(file, nonNumeric[j][4]); //used 16 to allow different country telephone numbers. //checking whether the telephone number is within a reasonable range. if(nonNumeric[j][4].length() > 16) { fout << "Student telephone number was too long, skipping to next student!" << endl; continue; } getline(file, nonNumeric[j][5]); //11 is the max social number. //checking whether the social is within a reasonable range. if(nonNumeric[j][5].length() > 11) { fout << "Student social was too long, skipping to next student!" << endl; continue; }
} }
void validateData(int numberic[3][2]) {
for(int j = 0; j < 3; j++) { file >> numeric[j][0]; //checking whether age is between 1 and 90 if(numeric[j][0] > 90 || numeric[j][0] < 1) { fout << "Age was not in the valid range" << endl; break; }
file >> numeric[j][1]; //checking whether number of years is between 1 and 90 if(numeric[j][1] > 90 || numeric[j][1] < 1) { fout << "Number of years at Texas state was not in the valid range" << endl; break; }
} }
int inputData() { //reading from the input file. file.open("Project5_input.txt"); if(!file) { cout << "Error in opening file: Project5_input.txt" << endl; return -1; }
return 0; }
void outputData() { fout.open("Project5_output.txt"); //Printing to the output file }
void writetoFile() { for(int i = 0; i <3; i++) { fout << setw(32) << nonNumeric[i][0] << endl; fout << setw(32) << "Name of Student:" << "\t" << nonNumeric[i][1] << endl; fout << setw(32) << "Student ID:" << "\t" << nonNumeric[i][3] << endl; fout << setw(32) << "Age:" << "\t" << numeric[i][0] << endl; fout << setw(32) << "Address:" << "\t" << nonNumeric[i][2] << endl; fout << setw(32) << "Number of years at Texas State:" << "\t" << numeric[i][1] << endl; fout << setw(32) << "Telephone Number:" << "\t" << nonNumeric[i][4] << endl; fout << setw(32) << "Student Social Security #:" << "\t" << nonNumeric[i][5] << endl << endl; for(int k = 0; k < 3; k++) { fout << setw(32) << "Course#:" << " " << nonNumeric[i][6+k] << endl; for(int j = 0; j < 5; j++) { fout << setw(30) << "Test#" << j + 1 << ":" << "\t" << numeric2[i][k][j] << endl; } fout << fixed << setw(32) << "Numerical Grade:" << "\t" << numeric2[i][k][5] << endl; fout << setw(32) << "Letter Grade:" << "\t" << letterGrade[i][k] << endl; if(numeric2[i][k][5] >= A_Score) { fout << setw(32) << "Commend Note:" << "\t" << "Congratulations! You have an A!" << endl; } else if(numeric2[i][k][5] < D_Score) { fout << setw(32) << "Warning Note:" << "\t" << "You Should Study! You are below a 70 grade average!" << endl; } fout << endl << endl; } } file.close(); fout.close(); }
int main() {
//get number of students cout << "Enter the number of Students: "; cin >> students; //loop for student information while(students >= 6 || students <= 0) { cout << "Incorrect range of students, please enter a number between 1 - 5" << endl; cin >> students; } inputData(); outputData(); validateData(nonNumeric); validateData(numeric); letGrade(numeric2); writetoFile(); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
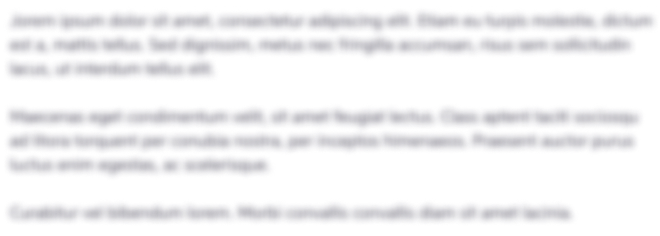
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started