Question
Modify Restart.action() to start the the system by reading events from a text file. Use Scanner and an appropriate regular expression. /////////////////////GreenhouseControls////////////////////////////////////////// public class GreenhouseControls
Modify Restart.action() to start the the system by reading events from a text file. Use Scanner and an appropriate regular expression.
/////////////////////GreenhouseControls//////////////////////////////////////////
public class GreenhouseControls extends Controller { private boolean light = false; private boolean water = false; private String thermostat = "Day"; private String eventsFile = "examples1.txt";
public class LightOn extends Event { public LightOn(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here to // physically turn on the light. light = true; } public String toString() { return "Light is on"; } } public class LightOff extends Event { public LightOff(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here to // physically turn off the light. light = false; } public String toString() { return "Light is off"; } } public class WaterOn extends Event { public WaterOn(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. water = true; } public String toString() { return "Greenhouse water is on"; } } public class WaterOff extends Event { public WaterOff(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. water = false; } public String toString() { return "Greenhouse water is off"; } } public class ThermostatNight extends Event { public ThermostatNight(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. thermostat = "Night"; } public String toString() { return "Thermostat on night setting"; } } public class ThermostatDay extends Event { public ThermostatDay(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. thermostat = "Day"; } public String toString() { return "Thermostat on day setting"; } } // An example of an action() that inserts a // new one of itself into the event list: public class Bell extends Event { public Bell(long delayTime) { super(delayTime); } public void action() { // nothing to do } public String toString() { return "Bing!"; } }
public class Restart extends Event { public Restart(long delayTime, String filename) { super(delayTime); eventsFile = filename; }
public void action() { addEvent(new ThermostatNight(0)); addEvent(new LightOn(2000)); addEvent(new WaterOff(8000)); addEvent(new ThermostatDay(10000)); addEvent(new Bell(9000)); addEvent(new WaterOn(6000)); addEvent(new LightOff(4000)); addEvent(new Terminate(12000)); }
public String toString() { return "Restarting system"; } }
public class Terminate extends Event { public Terminate(long delayTime) { super(delayTime); } public void action() { System.exit(0); } public String toString() { return "Terminating"; } }
public static void printUsage() { System.out.println("Correct format: "); System.out.println(" java GreenhouseControls -f
//--------------------------------------------------------- public static void main(String[] args) { try { String option = args[0]; String filename = args[1];
if ( !(option.equals("-f")) && !(option.equals("-d")) ) { System.out.println("Invalid option"); printUsage(); }
GreenhouseControls gc = new GreenhouseControls();
if (option.equals("-f")) { gc.addEvent(gc.new Restart(0,filename)); }
gc.run(); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Invalid number of parameters"); printUsage(); } }
} ///:~
///////Controler.java//////////////////////////////////////////
public class Controller { // A class from java.util to hold Event objects: private List
public void run() { while(eventList.size() > 0) // Make a copy so you're not modifying the list // while you're selecting the elements in it: for(Event e : new ArrayList
/////////////Event.java//////////////////////
package tme3;
import java.io.*;
public abstract class Event { private long eventTime; protected final long delayTime; public Event(long delayTime) { this.delayTime = delayTime; start(); } public void start() { // Allows restarting eventTime = System.currentTimeMillis() + delayTime; } public boolean ready() { return System.currentTimeMillis() >= eventTime; } public abstract void action();
/////////////////////GreenhouseControls//////////////////////////////////////////
public class GreenhouseControls extends Controller { private boolean light = false; private boolean water = false; private String thermostat = "Day"; private String eventsFile = "examples1.txt";
public class LightOn extends Event { public LightOn(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here to // physically turn on the light. light = true; } public String toString() { return "Light is on"; } } public class LightOff extends Event { public LightOff(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here to // physically turn off the light. light = false; } public String toString() { return "Light is off"; } } public class WaterOn extends Event { public WaterOn(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. water = true; } public String toString() { return "Greenhouse water is on"; } } public class WaterOff extends Event { public WaterOff(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. water = false; } public String toString() { return "Greenhouse water is off"; } } public class ThermostatNight extends Event { public ThermostatNight(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. thermostat = "Night"; } public String toString() { return "Thermostat on night setting"; } } public class ThermostatDay extends Event { public ThermostatDay(long delayTime) { super(delayTime); } public void action() { // Put hardware control code here. thermostat = "Day"; } public String toString() { return "Thermostat on day setting"; } } // An example of an action() that inserts a // new one of itself into the event list: public class Bell extends Event { public Bell(long delayTime) { super(delayTime); } public void action() { // nothing to do } public String toString() { return "Bing!"; } }
public class Restart extends Event { public Restart(long delayTime, String filename) { super(delayTime); eventsFile = filename; }
public void action() { addEvent(new ThermostatNight(0)); addEvent(new LightOn(2000)); addEvent(new WaterOff(8000)); addEvent(new ThermostatDay(10000)); addEvent(new Bell(9000)); addEvent(new WaterOn(6000)); addEvent(new LightOff(4000)); addEvent(new Terminate(12000)); }
public String toString() { return "Restarting system"; } }
public class Terminate extends Event { public Terminate(long delayTime) { super(delayTime); } public void action() { System.exit(0); } public String toString() { return "Terminating"; } }
public static void printUsage() { System.out.println("Correct format: "); System.out.println(" java GreenhouseControls -f
//--------------------------------------------------------- public static void main(String[] args) { try { String option = args[0]; String filename = args[1];
if ( !(option.equals("-f")) && !(option.equals("-d")) ) { System.out.println("Invalid option"); printUsage(); }
GreenhouseControls gc = new GreenhouseControls();
if (option.equals("-f")) { gc.addEvent(gc.new Restart(0,filename)); }
gc.run(); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Invalid number of parameters"); printUsage(); } }
} ///:~
///////Controler.java//////////////////////////////////////////
public class Controller { // A class from java.util to hold Event objects: private List
public void run() { while(eventList.size() > 0) // Make a copy so you're not modifying the list // while you're selecting the elements in it: for(Event e : new ArrayList
/////////////Event.java//////////////////////
package tme3;
import java.io.*;
public abstract class Event { private long eventTime; protected final long delayTime; public Event(long delayTime) { this.delayTime = delayTime; start(); } public void start() { // Allows restarting eventTime = System.currentTimeMillis() + delayTime; } public boolean ready() { return System.currentTimeMillis() >= eventTime; } public abstract void action();
//////////////DataFile.txt//////////////////////
Event=ThermostatNight,time=0 Event=LightOn,time=2000 Event=WaterOff,time=8000 Event=ThermostatDay,time=10000 Event=Bell,time=9000 Event=WaterOn,time=6000 Event=LightOff,time=4000 Event=Terminate,time=12000
Scanner scan= new Scanner(eventsFile); while(scan.hasNextLine()){ String line= scan.nextLine();
Step by Step Solution
There are 3 Steps involved in it
Step: 1
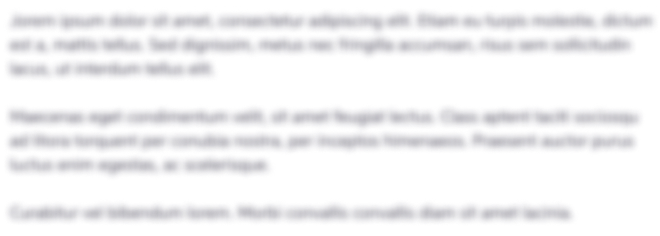
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started