Question
Modify the ArrayList implementation so that all methods can be safely accessed from multiple threads using the given code. ArrayList.java public class ArrayList { private
Modify the ArrayList implementation so that all methods can be safely accessed from multiple threads using the given code.
ArrayList.java
public class ArrayList
{
private Object[] buffer;
private int currentSize;
private boolean lock;
/**
Constructs an empty array list.
*/
public ArrayList()
{
final int INITIAL_SIZE = 10;
buffer = new Object[INITIAL_SIZE];
currentSize = 0;
lock = false;
}
/**
Gets the size of this array list.
@return the size
*/
public int size()
{
//complete this method
}
/**
Throws an IndexOutOfBoundsException if the checked index is out of bounds
@param n the index to check
*/
private void checkBounds(int n)
{
if (n < 0 || n >= currentSize)
{
throw new IndexOutOfBoundsException();
}
}
/**
Gets the element at a given position.
@param pos the position
@return the element at pos
*/
public Object get(int pos)
{
//complete this method
}
/**
Sets the element at a given position.
@param pos the position
@param element the new value
*/
public void set(int pos, Object element)
{
//complete this method
}
/**
Removes the element at a given position.
@param pos the position
@return the removed element
*/
public Object remove(int pos)
{
//complete this method
}
/**
Adds an element after a given position.
@param pos the position
@param newElement the element to add
@return true
*/
public boolean add(int pos, Object newElement)
{
//complete this method
}
/**
Adds an element after the end of the array list
@param newElement the element to add
@return true
*/
public boolean addLast(Object newElement)
{
//complete this method
}
/**
Grows the buffer if the current size equals the buffer's capacity.
*/
private void growBufferIfNecessary()
{
if (currentSize == buffer.length)
{
Object[] newBuffer = new Object[2 * buffer.length];
for (int i = 0; i < buffer.length; i++)
{
newBuffer[i] = buffer[i];
}
buffer = newBuffer;
}
}
}
ArrayListDemo
import java.util.Random;
/** This demo for demonstrating a thread safe array list. */ public class ArrayListThreadDemo implements Runnable { private static ArrayList list; private static Random randomizer;
public void run() { int choice = list.size(); if (list.size() > 0) { choice = randomizer.nextInt(list.size() + 1); } if (choice == list.size()) { String token = randomizer.nextInt(1000) + ""; list.addLast(token); System.out.println("Added: " + token); } else { String token = (String) list.remove(choice); System.out.println("Removed: " + token); } if (list.size() > 0) { run(); } }
public static void main(String[] args) { randomizer = new Random(); list = new ArrayList(); Thread thread1 = new Thread(new ArrayListThreadDemo()); thread1.start(); Thread thread2 = new Thread(new ArrayListThreadDemo()); thread2.start(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
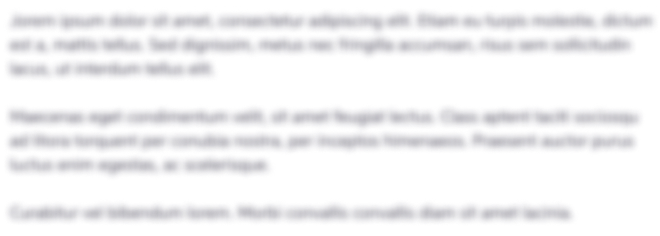
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started