Question
Modify the C program in Fig.7.24 (provided on Canvas) so that the card-dealing function deals a five-card poker hand. Then write the following additional functions:
Modify the C program in Fig.7.24 (provided on Canvas) so that the card-dealing function deals a five-card poker hand. Then write the following additional functions:
a)Determine whether the hand contains two pairs
b) Determine whether the hand contains a full house (i.e.,three of a kind with pair).
c) Determine whether the hand contains a straight flush (i.e., five cards of consecutive face values).
d) Determine whether the hand contains a flush (i.e., five of the same suit)
Here is my code ......
// Fig. 7.24: fig07_24.c // Card shuffling and dealing. #include
#define SUITS 4 #define FACES 13 #define CARDS 52
// prototypes void shuffle(unsigned int wDeck[][FACES]); // shuffling modifies wDeck void deal(unsigned int wDeck[][FACES], const char *wFace[], const char *wSuit[],unsigned int *handptr); // dealing doesn't modify the arrays
int main(void) { // initialize deck array unsigned int deck[SUITS][FACES] = { 0 };
srand(time(NULL)); // seed random-number generator
shuffle(deck); // shuffle the deck
// initialize suit array const char *suit[SUITS] = {"Hearts", "Diamonds", "Clubs", "Spades"}; // initialize face array const char *face[FACES] = {"Ace", "Deuce", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King"}; unsigned int hand[5];
deal(deck, face, suit, hand); // deal the deck }
// shuffle cards in deck void shuffle(unsigned int wDeck[][FACES]) { // for each of the cards, choose slot of deck randomly for (size_t card = 1; card <= CARDS; ++card) { size_t row; // row number size_t column; // column number
// choose new random location until unoccupied slot found do { row = rand() % SUITS; column = rand() % FACES; } while(wDeck[row][column] != 0); // end do...while
// place card number in chosen slot of deck wDeck[row][column] = card; } }
// deal cards in deck void deal(unsigned int wDeck[][FACES], const char *wFace[], const char *wSuit[], unsigned int *handptr) { // deal each of the cards for (size_t card = 1; card <= 5; ++card) { // loop through rows of wDeck for (size_t row = 0; row < SUITS; ++row) { // loop through columns of wDeck for current row for (size_t column = 0; column < FACES; ++column) {
// if slot contains current card, display card if (wDeck[row][column] == card) { handptr[card - 1] = wDeck[row][column]; printf("%5s of %-8s (%zd, %zd) %c", wFace[column], wSuit[row], row, column, card % 2 == 0 ? ' ' : '\t'); // 2-column format } } } } printf(" "); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
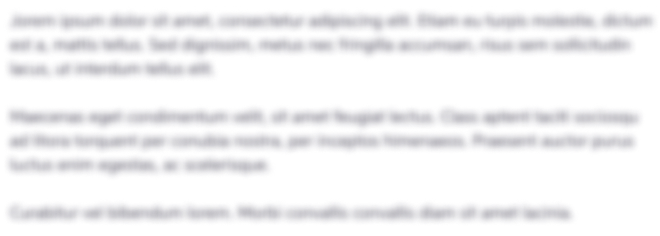
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started