Question
Modify the class below (SlotMachine)so that it simulates a slot machine. There are three spinners that display digits. When the button is clicked, the spinners
Modify the class below (SlotMachine)so that it simulates a slot machine. There are three spinners that display digits. When the button is clicked, the spinners should all start "spinning" (that is, they continuously increment the digit displayed, wrapping around to zero when necessary). Each subsequent time the button is clicked, one spinner should stop. After all three spinners stop, the game should display a message indicating whether the player won (all three digits are the same). If the user clicks the button again, the spinners all start spinning and the game continues.
___________________________________________________________________________________
package slotmachine;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class SlotMachine extends JFrame implements ActionListener {
public static void main(String[] args) {
new SlotMachine();
}
private JLabel spinner1; // Left digit display
private JLabel spinner2; // Middle digit display
private JLabel spinner3; // Right digit display
private int[] values; // Digit values
private JButton startStop; // Button to start or stop spinner(s)
// Constructor
public SlotMachine() {
super("Slots!");
values = new int[3];
makeFrame();
}
// Makes all three digits start spinning.
public void startSpinning() {
// WRITE ME!!!
}
// Makes one digit stop spinning.
// If all digits stop, displays a message if all three digits are
the same.
public void stopSpinning() {
// WRITE ME TOO!!!
}
// This method is called when Start/Stop button is clicked.
public void actionPerformed(ActionEvent e) {
if(startStop.getText().equals("START")) {
startStop.setText("STOP");
startSpinning();
} else {
stopSpinning();
}
}
// Builds the window and makes it appear!
private void makeFrame() {
setLayout(new BorderLayout(5, 5));
JPanel spinnerPanel = new JPanel(new GridLayout(1, 3, 5,
5));
spinner1 = new JLabel("0", JLabel.CENTER);
spinner1.setFont(new Font(null, Font.BOLD, 40));
spinnerPanel.add(spinner1);
spinner2 = new JLabel("0", JLabel.CENTER);
spinner2.setFont(new Font(null, Font.BOLD, 40));
spinnerPanel.add(spinner2);
spinner3 = new JLabel("0", JLabel.CENTER);
spinner3.setFont(new Font(null, Font.BOLD, 40));
spinnerPanel.add(spinner3);
add(spinnerPanel, BorderLayout.CENTER);
startStop = new JButton("START");
startStop.setFont(new Font(null, Font.ITALIC, 20));
startStop.addActionListener(this);
add(startStop, BorderLayout.SOUTH);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(200, 150);
setLocationRelativeTo(null);
setVisible(true);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
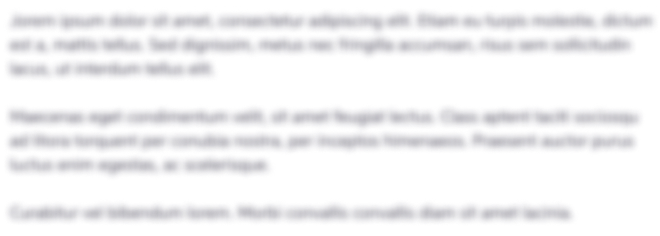
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started