Question
modify the code to get the expected output(shown at the bottom) using constructions provided: change the instance variables representing the number of students and
modify the code to get the expected output(shown at the bottom) using constructions provided:
change the instance variables representing the number of students and the Student array in the aggregator object to private static variables. change the addStudent method in the aggregator object from an instance method to a static method Remove all initialization/instantiation operations from the aggregator object's default constructor; the constructor can simple be an empty method { } provide a static initializer block in the aggregator object which does the following: o initializes the number of students to 0 o instantiates the student array o adds a single student named "Test Student" to the array using the addStudent method This is the most important part of this assignment, the static initializer block must be included in your submission. The remaining methods (including the main method) in the aggregator object should remain the same. The aggregated Student and Instructor classes should remain the same. Include all three classes in a single Java source code file, do not set the Student or Instuctor class visibility to public, this will keep the compiler happy.
Sample Output (notice the initial student created by the static initializer block):
Instructor = Sally Number of students = 4 Students: [Test Student, Sam, Rajiv, Jennifer, noname, noname, noname, noname, noname, noname]
*/
import java.util.Arrays;
public class InitializerDemo { public static final int MAX_STUDENTS = 10; private Student[] students; private Instructor instructor; private int numStudents = 0; // default constructor public InitializerDemo() { this.students = new Student[MAX_STUDENTS]; for (int i = 0; i < MAX_STUDENTS; i++) this.students[i] = new Student(); } // instructor mutator public void setInstructor(Instructor instructor) { this.instructor = instructor; } // add student and increment the count public void addStudent(Student s) { this.students[numStudents++] = s; } // toString() method which returns the String representation of the current // state of the aggregator object, including the instructor name, number of // students, and names of students. public String toString() { String s = "Instructor = " + instructor + " " + "Number of students = " + numStudents + " " + "Students = " + Arrays.toString(students) + " "; return s; } // main method which does the following: // o instantiates an instance of the aggregator class // o Sets the instructor (including their name) // o Adds three (or more, but no more than ten) new students using the addStudent method // o Prints the current state of the object public static void main(String[] args) { // create our aggregator object InitializerDemo id = new InitializerDemo(); // set the instructor id.setInstructor(new Instructor("Sally")); // add the students id.addStudent(new Student("Sam")); id.addStudent(new Student("Rajiv")); id.addStudent(new Student("Jennifer")); // output System.out.println(id); } }
class Student { private String name; // instance intializer block { name = "noname"; } // default constructor public Student() { //System.out.println("in Student default constructor."); } // overloaded constructor public Student(String name) { //System.out.println("in Student overload constructor."); this.name = name; } public String toString() { return name; } }
class Instructor { private String name; // instance intializer block { name = "noname"; } // default constructor public Instructor() { //System.out.println("in Instructor default constructor."); } // overloaded constructor public Instructor(String name) { //System.out.println("in Instructor overload constructor."); this.name = name; } public String toString() { return name; } }
Step by Step Solution
3.34 Rating (160 Votes )
There are 3 Steps involved in it
Step: 1
Below is the modified code based on the provided instructions import javautilArrays public class InitializerDemo private static final int MAXSTUDENTS ...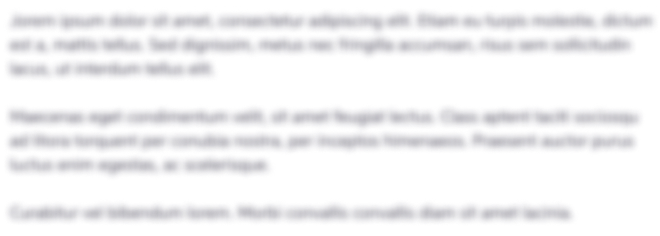
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started