Modify the code to show each step that was taken in order to sort the array or search it. class SelectionSort { void sort(int arr[])
Modify the code to show each step that was taken in order to sort the array or search it.
class SelectionSort { void sort(int arr[]) { int n = arr.length;
// One by one move boundary of unsorted subarray for (int i = 0; i < n-1; i++) { // Find the minimum element in unsorted array int min_idx = i; for (int j = i+1; j < n; j++) if (arr[j] < arr[min_idx]) min_idx = j; // Swap the found minimum element with the first // element int temp = arr[min_idx]; arr[min_idx] = arr[i]; arr[i] = temp; } }
// Prints the array void printArray(int arr[]) { int n = arr.length; for (int i=0; i // Driver code to test above public static void main(String args[]) { SelectionSort ob = new SelectionSort(); int arr[] = {64,25,12,22,11}; ob.sort(arr); System.out.println("Sorted array"); ob.printArray(arr); } } ----------------------------------------------------------------------------------- public class BinarySearch { // Returns index of x if it is present in arr[l.. // r], else return -1 int binarySearch(int arr[], int l, int r, int x) { if (r >= l) { int mid = l + (r - l) / 2; // If the element is present at the // middle itself if (arr[mid] == x) return mid; // If element is smaller than mid, then // it can only be present in left subarray if (arr[mid] > x) return binarySearch(arr, l, mid - 1, x); // Else the element can only be present // in right subarray return binarySearch(arr, mid + 1, r, x); } // We reach here when element is not present // in array return -1; } // Driver method to test above public static void main(String args[]) { BinarySearch ob = new BinarySearch(); int arr[] = { 2, 3, 4, 10, 40 }; int n = arr.length; int x = 40; int result = ob.binarySearch(arr, 0, n - 1, x); if (result == -1) System.out.println("Element not present"); else System.out.println("Element found at index " + result); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
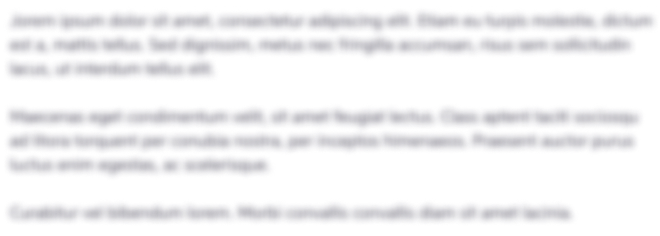
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started