Question
Modify the example such that the parent creates 8 child processes. After creating 8 child processes, the parent will send something to the children processes.
Modify the example such that the parent creates 8 child processes. After creating 8 child processes, the parent will send something to the children processes. The children, after receiving the message, will reply to their parent with their respective message.
#include
#include
char string0[] = "Hello, this is the parent process";
char string1[] = "Hi, this is the child process 1";
char string2[] = "Hi, this is the child process 2";
char string3[] = "Hi, this is the child process 3";
char string4[] = "Hi, this is the child process 4";
char string5[] = "Hi, this is the child process 5";
char string6[] = "Hi, this is the child process 6";
char string7[] = "Hi, this is the child process 7";
char string8[] = "Hi, this is the child process 8";
main(){
char buf[1024];
int i, fds0[2], fds1[2], fds2[2],fds3[2],fds4[2],fds5[2],fds6[2],fds7[2],fds8[2];
pipe(fds0); //pipe used by the parent process--zs
pipe(fds1); //pipe used by the child 1 process--zs
pipe(fds2); //pipe used by the child 2 process--zs
pipe(fds3); //pipe used by the child 3 process--zs
pipe(fds4); //pipe used by the child 4 process--zs
pipe(fds5); //pipe used by the child 5 process--zs
pipe(fds6); //pipe used by the child 6 process--zs
pipe(fds7); //pipe used by the child 7 process--zs
pipe(fds8); //pipe used by the child 8 process--zs
//The first child process is created.--zs
if(fork()==0)
{
close(fds0[1]);
//read from the parent --zs
read(fds0[0], buf, sizeof(string0));
printf("child 1 reads: %s ", buf);
//write child message to parent via its pipe--zs
close(fds1[0]);
write(fds1[1], string1, sizeof(string1));
exit(0);
}
//The second child process--zs
else if(fork()==0)
{
sleep(1);
close(fds0[1]);
//Get something from the parent process--zs
read(fds0[0], buf, sizeof(string0));
printf("child 2 reads: %s ", buf);
//write child message into fds2
close(fds2[0]);
write(fds2[1], string2, sizeof(string2));
exit(0);
}
//3rd
else if(fork()==0)
{
sleep(1);
close(fds0[1]);
//Get something from the parent process--zs
read(fds0[0], buf, sizeof(string0));
printf("child 3 reads: %s ", buf);
//write child message into fds2
close(fds3[0]);
write(fds3[1], string3, sizeof(string3));
exit(0);
}
//4th
else if(fork()==0) {
sleep(3);
close(fds0[1]);
//Get something from the parent process--zs
read(fds0[0], buf, sizeof(string0));
printf("child 4 reads: %s ", buf);
//write child message into fds2
close(fds4[0]);
write(fds4[1], string4, sizeof(string4));
exit(0);
}
//5th
else if(fork()==0) {
sleep(4);
close(fds0[1]);
//Get something from the parent process--zs
read(fds0[0], buf, sizeof(string0));
printf("child 5 reads: %s ", buf);
//write child message into fds2
close(fds5[0]);
write(fds5[1], string5, sizeof(string5));
exit(0);
}
//6th
else if(fork()==0) {
sleep(5);
close(fds0[1]);
//Get something from the parent process--zs
read(fds0[0], buf, sizeof(string0));
printf("child 6 reads: %s ", buf);
//write child message into fds2
close(fds6[0]);
write(fds6[1], string6, sizeof(string6));
exit(0);
}
//7th
else if(fork()==0) {
sleep(6);
close(fds0[1]);
//Get something from the parent process--zs
read(fds0[0], buf, sizeof(string0));
printf("child 7 reads: %s ", buf);
//write child message into fds2
close(fds7[0]);
write(fds7[1], string7, sizeof(string7));
exit(0);
}
//8th
else if(fork()==0) {
sleep(7);
close(fds0[1]);
//Get something from the parent process--zs
read(fds0[0], buf, sizeof(string0));
printf("child 8 reads: %s ", buf);
//write child message into fds2
close(fds8[0]);
write(fds8[1], string8, sizeof(string8));
exit(0);
}
else {//Parent process starts--zs
//write parent message into fds0
close(fds0[0]);
write(fds0[1], string0, sizeof(string0));
//read child 1 message from its associated pipe--zs
close(fds1[1]);
read(fds1[0],buf,sizeof(string1));
printf("parent reads from Child 1: %s ", buf);
//write something into fds0 again to child 2--zs
close(fds0[0]);
write(fds0[1], string0, sizeof(string0));
//read child 2 message from its associated pipe--zs
close(fds2[1]);
read(fds2[0],buf,sizeof(string2));
printf("parent reads from Child 2: %s ", buf);
exit(0);
close(fds0[0]);
write(fds0[1], string0, sizeof(string0));
//read child 3 message from its associated pipe--zs
close(fds3[1]);
read(fds3[0],buf,sizeof(string3));
printf("parent reads from Child 3: %s ", buf);
exit(0);
close(fds0[0]);
write(fds0[1], string0, sizeof(string0));
//read child 4 message from its associated pipe--zs
close(fds4[1]);
read(fds4[0],buf,sizeof(string4));
printf("parent reads from Child 4: %s ", buf);
exit(0);
close(fds0[0]);
write(fds0[1], string0, sizeof(string0));
//read child 5 message from its associated pipe--zs
close(fds5[1]);
read(fds5[0],buf,sizeof(string5));
printf("parent reads from Child 5: %s ", buf);
exit(0);
close(fds0[0]);
write(fds0[1], string0, sizeof(string0));
//read child 6 message from its associated pipe--zs
close(fds6[1]);
read(fds6[0],buf,sizeof(string6));
printf("parent reads from Child 6: %s ", buf);
exit(0);
close(fds0[0]);
write(fds0[1], string0, sizeof(string0));
//read child 7 message from its associated pipe--zs
close(fds7[1]);
read(fds7[0],buf,sizeof(string7));
printf("parent reads from Child 7: %s ", buf);
exit(0);
close(fds0[0]);
write(fds0[1], string0, sizeof(string0));
//read child 8 message from its associated pipe--zs
close(fds8[1]);
read(fds8[0],buf,sizeof(string8));
printf("parent reads from Child 8: %s ", buf);
exit(0);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
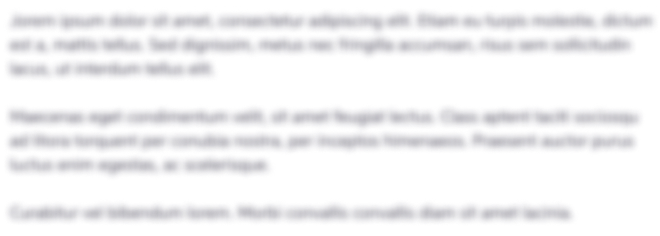
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started