Question
Modify the given code as follows: Check the command line arguments. A file is specified as -f textfile. If a file has not been specified,
Modify the given code as follows:
Check the command line arguments. A file is specified as "-f textfile". If a file has not been specified, exit the program with a meaningful error message.
Open the input file specified by argv[2] and enter into an endless loop that reads and processes the text lines. Save each text line in a cache (e.g., vector
Add a second endless loop that prompts the user for words to search for. Print all line numbers and the corresponding text for each successfull search. Print nothing for an unsuccessfull search. Use the vector of line numbers returned by hash_table::find() to do this. Look up the text in the above mentioned data cache for each line number. See output examples below.
Make no assumptions about the number of lines in the input file. Make no assumptions about the number of characters or words on each line. The test files may be pure ascii text, html code, or even a C++ program. Your code should work regardless of the type of ascii data stored in the file.
#include <...>
using namespace std;
typedef unsigned int uint;
template
class hash_table {
public:
hash_table(int N=9999);
void insert(const Tkey &);
private:
int hash(const Tkey &);
vector
};
template
hash_table
table.assign(N, Tkey());
}
template
void hash_table
int index = hash(key);
cout << setw(20) << left << key
<< "index "
<< setw(3) << right << index;
if (table[index] == key) {
cout << " -- key repeat (" << table[index] << ")";
} else if (table[index] == Tkey()) {
table[index] = key;
} else {
cout << " -- collision (" << table[index] << ")";
}
cout << " ";
}
Hint: Keys that hash to the index produces collisions. Above we detect and report them. Next handout provides
work arounds.
template<>
int hash_table
return (uint)key % table.size();
}
template<>
int hash_table
return *(uint *)&key % table.size();
}
template<>
int hash_table
uint index = 0;
const char *p = key.c_str();
while (*p)
index = ((index << 5) | (index >> 27)) + *p++;
return index % table.size();
}
int main(int argc, char *argv[]) {
hash_table
string key;
while (cin >> key)
H.insert(key);
}
Hint: The odd-looking type casting for hashing of floats serves to ensure full use of the underlying 32-bit data.
Hint: The binary logic used when hashing strings serves to implement cyclic shifting to prevent loss of bits as
more and more chars are added to the index. The 5 MSB bits become LSB bits. The remaining 27 bits are shifted
left to become the new MSB bits.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
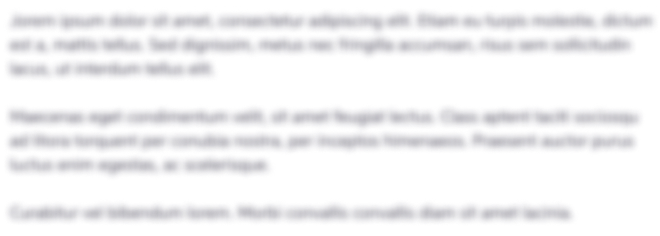
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started