Question
Modify the implementation of DrawVisitor.java to display a tree. The algorithm is listed below in the picture. DrawVisitor.class public class DrawVisitor extends ASTVisitor { private
Modify the implementation of DrawVisitor.java to display a tree. The algorithm is listed below in the picture.
DrawVisitor.class
public class DrawVisitor extends ASTVisitor {
private final int nodew = 100; private final int nodeh = 30; private final int vertSep = 50; private final int horizSep = 10;
private int width; private int height;
private int [] nCount; private int [] progress; private int depth = 0; private BufferedImage bimg; private Graphics2D g2;
public DrawVisitor(int [] nCount) { this.nCount = nCount; progress = new int [nCount.length];
width = max(nCount)*(nodew + horizSep); height = nCount.length*(nodeh + vertSep);
g2 = createGraphics2D(width,height); }
private int max(int [] array) { int max = array[0]; for(int i = 1; i
public void draw(String s, AST t) { int hstep = nodew + horizSep; int vstep = nodeh + vertSep;
int x = width/2 + progress[depth]*hstep - nCount[depth]*hstep/2; int y = depth*vstep;
g2.setColor(Color.black); g2.drawOval(x, y, nodew, nodeh); g2.setColor(Color.BLACK); g2.drawString(s, x+10, y+2*nodeh/3);
int startx = x + nodew/2; int starty = y + nodeh; int endx; int endy; g2.setColor(Color.black); for(int i = 0; i
progress[depth]++; depth++; visitKids(t); depth--; }
private Graphics2D createGraphics2D(int w, int h) { Graphics2D g2; if (bimg == null || bimg.getWidth() != w || bimg.getHeight() != h) { bimg = new BufferedImage(w, h,BufferedImage.TYPE_INT_RGB); } g2 = bimg.createGraphics(); g2.setBackground(Color.WHITE); g2.setRenderingHint(RenderingHints.KEY_RENDERING, RenderingHints.VALUE_RENDER_QUALITY); g2.clearRect(0, 0, w, h); return g2; }
@Override public Object visitProgramTree(AST t) { draw("Program",t); return null; } @Override public Object visitBlockTree(AST t) { draw("Block",t); return null; } @Override public Object visitFunctionDeclTree(AST t) { draw("FunctionDecl",t); return null; } @Override public Object visitCallTree(AST t) { draw("Call",t); return null; } @Override public Object visitDeclTree(AST t) { draw("Decl",t); return null; } @Override public Object visitIntTypeTree(AST t) { draw("IntType",t); return null; } @Override public Object visitBoolTypeTree(AST t) { draw("BoolType",t); return null; } @Override public Object visitFormalsTree(AST t) { draw("Formals",t); return null; } @Override public Object visitActualArgsTree(AST t) { draw("ActualArgs",t); return null; } @Override public Object visitIfTree(AST t) { draw("If",t); return null; } @Override public Object visitWhileTree(AST t) { draw("While",t); return null; } @Override public Object visitReturnTree(AST t) { draw("Return",t); return null; } @Override public Object visitAssignTree(AST t) { draw("Assign",t); return null; } @Override public Object visitIntTree(AST t) { draw("Int: "+((IntTree)t).getSymbol().toString(),t); return null; } @Override public Object visitIdTree(AST t) { draw("Id: "+((IdTree)t).getSymbol().toString(),t); return null; } @Override public Object visitRelOpTree(AST t) { draw("RelOp: "+((RelOpTree)t).getSymbol().toString(),t); return null; } public Object visitAddOpTree(AST t) { draw("AddOp: "+((AddOpTree)t).getSymbol().toString(),t); return null; } public Object visitMultOpTree(AST t) { draw("MultOp: "+((MultOpTree)t).getSymbol().toString(),t); return null; } //public Object visitUnaryOpTree (AST t) { draw("UnaryOp: "+((UnaryOpTree)t).getSymbol().toString(),t); return null; } /ew methods here public Object visitFloatTree(AST t) { draw("Float: "+((FloatTree)t).getSymbol().toString(),t); return null; } public Object visitFloatTypeTree(AST t) { draw("FloatType",t); return null; } public Object visitRepeatTree(AST t) { draw("Repeat",t); return null; }
@Override public Object visitCharTypeTree(AST t) { draw("CharTypeTree: ",t); return null;}
@Override public Object visitCharTree(AST t) { draw("Char: "+((CharTree)t).getSymbol().toString(),t); return null; }
@Override public Object visitScientificNTree(AST t) { draw("ScientificN: "+((ScientificNTree)t).getSymbol().toString(),t); return null; }
@Override public Object visitDoTree(AST t) { draw("Do",t); return null; }
@Override public Object visitScientificNTypeTree(AST t) { draw("ScientificNType",t); return null; }
public Object visiVOIDTree(AST t) { draw("Void: "+((VoidTree)t).getSymbol().toString(),t); return null; } }
ASTVisitor class
public abstract class ASTVisitor {
public void visitKids(AST t) { for (AST kid : t.getKids()) { kid.accept(this); } return; }
public abstract Object visitProgramTree(AST t); public abstract Object visitBlockTree(AST t); public abstract Object visitFunctionDeclTree(AST t); public abstract Object visitCallTree(AST t); public abstract Object visitDeclTree(AST t); public abstract Object visitIntTypeTree(AST t); public abstract Object visitVOIDTypeTree(AST t); public abstract Object visitBoolTypeTree(AST t); public abstract Object visitFormalsTree(AST t); public abstract Object visitActualArgsTree(AST t); public abstract Object visitIfTree(AST t); public abstract Object visitWhileTree(AST t); public abstract Object visitReturnTree(AST t); public abstract Object visitAssignTree(AST t); public abstract Object visitIntTree(AST t); public abstract Object visitIdTree(AST t); public abstract Object visitRelOpTree(AST t); public abstract Object visitAddOpTree(AST t); public abstract Object visitMultOpTree(AST t); public abstract Object visitCharTree(AST t); public abstract Object visitCharTypeTree(AST t); public abstract Object visitFloatTree(AST t); public abstract Object visitFloatTypeTree(AST t); public abstract Object visitDoTree(AST t); public abstract Object visitScientificNTree(AST t); public abstract Object visitScientificNTypeTree(AST t); }
CountVisitor.java
public class CountVisitor extends ASTVisitor { private int [] nCount = new int[100]; private int depth = 0; private int maxDepth = 0; private void count(AST t) { nCount[depth]++; if(depth > maxDepth) {maxDepth = depth;} depth++; visitKids(t); depth--; } public int[] getCount() { int [] count = new int[maxDepth + 1]; for(int i = 0; i
public Object visitProgramTree(AST t) {count(t); return null;} public Object visitBlockTree(AST t) {count(t); return null;} public Object visitFunctionDeclTree(AST t) {count(t); return null; } public Object visitCallTree(AST t) {count(t); return null; } public Object visitDeclTree(AST t) {count(t); return null; } public Object visitIntTypeTree(AST t) {count(t); return null; } public Object visitBoolTypeTree(AST t) {count(t); return null; } public Object visitFormalsTree(AST t) {count(t); return null; } public Object visitActualArgsTree(AST t) {count(t); return null; } public Object visitIfTree(AST t) {count(t); return null; } public Object visitWhileTree(AST t) {count(t); return null; } public Object visitReturnTree(AST t) {count(t); return null; } public Object visitAssignTree(AST t) {count(t); return null; } public Object visitIntTree(AST t) {count(t); return null; } public Object visitIdTree(AST t) {count(t); return null; } public Object visitRelOpTree(AST t) {count(t); return null; } public Object visitAddOpTree(AST t) {count(t); return null; } public Object visitMultOpTree(AST t) {count(t); return null; } public Object visitUnaryOpTree (AST t) {count(t); return null; } /ew methods here public Object visitFloatTree(AST t) {count(t); return null; } public Object visitFloatTypeTree(AST t) {count(t); return null; } public Object visitRepeatTree(AST t) {count(t); return null; }
@Override public Object visitCharTypeTree(AST t) {count(t); return null;}
@Override public Object visitCharTree(AST t) {count(t); return null;}
@Override public Object visitScientificNTree(AST t) {count(t); return null;}
@Override public Object visitDoTree(AST t) {count(t); return null;}
@Override public Object visitScientificNTypeTree(AST t) {count(t); return null;}
@Override public Object visitVOIDTypeTree(AST t) { count(t); return null; } }
PrintVisitor.java
public class PrintVisitor extends ASTVisitor { private int indent = 0;
private void printSpaces(int num) { String s = ""; for (int i = 0; i
/** * Print the tree * @param s is the String for the root of t * @param t is the tree to print - print the information * in the node at the root (e.g. decoration) and its kids * indented appropriately */ public void print(String s,AST t) { // assume less than 1000 nodes; no problem for csc 413 int num = t.getNodeNum(); AST decoration = t.getDecoration(); int decNum = (decoration == null)? -1 : decoration.getNodeNum(); String spaces = ""; if (num 0 ) { s += " Label: "+t.getLabel(); } if (t.getClass() == IdTree.class) { int offset = ((IdTree)t).getFrameOffset(); if (offset >= 0) { s += " Addr: " + offset; } } System.out.println(s); indent += 2; visitKids(t); indent -= 2; }
public Object visitProgramTree(AST t) { print("Program",t); return null; } public Object visitBlockTree(AST t) { print("Block",t); return null; } public Object visitFunctionDeclTree(AST t) { print("FunctionDecl",t); return null; } public Object visitCallTree(AST t) { print("Call",t); return null; } public Object visitDeclTree(AST t) { print("Decl",t); return null; } public Object visitIntTypeTree(AST t) { print("IntType",t); return null; } public Object visitBoolTypeTree(AST t) { print("BoolType",t); return null; } public Object visitFormalsTree(AST t) { print("Formals",t); return null; } public Object visitActualArgsTree(AST t) { print("ActualArgs",t); return null; } public Object visitIfTree(AST t) { print("If",t); return null; } public Object visitWhileTree(AST t) { print("While",t); return null; } public Object visitReturnTree(AST t) { print("Return",t); return null; } public Object visitAssignTree(AST t) { print("Assign",t); return null; } public Object visitIntTree(AST t) { print("Int: "+((IntTree)t).getSymbol().toString(),t); return null; } public Object visitIdTree(AST t) { print("Id: "+((IdTree)t).getSymbol().toString(),t); return null; } public Object visitRelOpTree(AST t) { print("RelOp: "+((RelOpTree)t).getSymbol().toString(),t); return null; } public Object visitAddOpTree(AST t) { print("AddOp: "+((AddOpTree)t).getSymbol().toString(),t); return null; } public Object visitMultOpTree(AST t) { print("MultOp: "+((MultOpTree)t).getSymbol().toString(),t); return null; } public Object visitCharTree(AST t) { print("Char: "+((CharTree)t).getSymbol().toString(),t); return null; } public Object visitCharTypeTree(AST t) { print("CharType",t); return null; } public Object visitDoTree(AST t) {print("Do",t); return null;}; public Object visitFloatTypeTree(AST t) { print("FloatType",t); return null; } public Object visitScientificNTypeTree(AST t) {print("ScientificNType",t); return null; } public Object visitFloatTree(AST t) {print("Float: "+((FloatTree)t).getSymbol().toString(),t); return null;} public Object visitScientificNTree(AST t) {print("ScientificN: "+((ScientificNTree)t).getSymbol().toString(),t); return null;} // public Object visitUnaryOpTree(AST t) { print("UnaryOp: "+((UnaryOpTree)t).getSymbol().toString(),t); return null; }
@Override public Object visitVOIDTypeTree(AST t) { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
}
(this is effectively done already order (this is effectively done already with the visit at 0 next available X offset for the current depth, starting b. Base case: if the node has no children, then record that node's X offset to be the next available offset for the current depth, update the offset, and return c. After visiting all children, calculate the X offset for the parent rightmost child's X offset, plus left most child's X offset), quantity divided by 2. 1) If the calculated offset is less than the next available offset for the current depth, we must recursively shift all children by next available offset for the current depth calculated X offset) 2) Set the current node's offset 3) Update the next available offset for the current depth (this is effectively done already order (this is effectively done already with the visit at 0 next available X offset for the current depth, starting b. Base case: if the node has no children, then record that node's X offset to be the next available offset for the current depth, update the offset, and return c. After visiting all children, calculate the X offset for the parent rightmost child's X offset, plus left most child's X offset), quantity divided by 2. 1) If the calculated offset is less than the next available offset for the current depth, we must recursively shift all children by next available offset for the current depth calculated X offset) 2) Set the current node's offset 3) Update the next available offset for the current depth
Step by Step Solution
There are 3 Steps involved in it
Step: 1
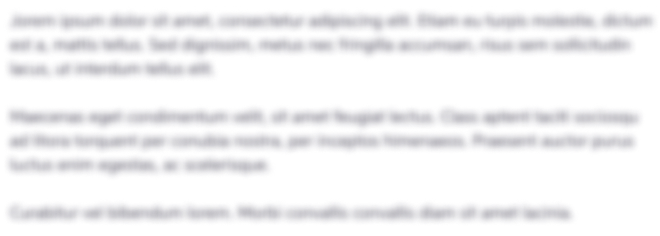
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started