Question
Modify the program below (Please do modify THE PROGRAM BELOW) to do vector operations on 3D vectors instead of scalars or real numbers. Each 3D
Modify the program below (Please do modify THE PROGRAM BELOW) to do vector operations on 3D vectors instead of scalars or real numbers. Each 3D vector is specified as three numbers bounded by [ and ], and separated by a blank space. Assume that there are no errors ever in input. Assume that * indicates dot product. Replace / with x and use that to indicate cross product. Also, include an operation m to indicate magnitude of a vector, u to indicate the unit vector of a given vector, a to indicate the angle in degrees between two given vectors, and s to indicate scalar multiplication.
To run the program below you must make a "inputfile.txt" & "outputfile.txt" files in the root of the project folder. Once you copied the code below you must go to the Project menu and click on properties for example "cheggprog98 properties". In the dialog box select Debugging under Configuration Properties and set the Command Arguments as "inputfile.txt outputfile.txt". Just as an example to show the program is working as designed you may enter the following in the inputfile.txt and then check the outputfile.txt to see that it has done the operations corrrectly:
+ 7 8
- 12 5
+ 2.1 4.6
- 5 6
q
This is the exact sample data the modified program will need to calculate from inputfile.txt:
+ [ 1.1 2.2 3.3 ] [ 4.4 5.5 6.6]
- [ 1.1 2.2 3.3 ] [ 4.4 5.5 6.6]
* [ 1.1 2.2 3.3 ] [ 4.4 5.5 6.6]
x [ 1.1 2.2 3.3 ] [ 4.4 5.5 6.6]
a [ 1.1 2.2 3.3 ] [ 4.4 5.5 6.6]
m [ 1.1 2.2 3.3 ]
u [ 1.1 2.2 3.3 ]
s 2.5 [ 1.1 2.2 3.3 ]
CODE:
#include
using namespace std;
#include
#include
// void main() {
int main(int argc, char *argv[]) {
char c;
float x, y, z;
if (argc != 3) {
cout << endl << "Usage: " << argv[0] << "input-file output-file" << endl;
exit(1);
}
ifstream fin(argv[1]);
ofstream fout(argv[2]);
if (!fin) {
cerr << "cannot open " << argv[1] << endl;
exit(1);
}
if (!fout) {
cerr << "cannot open " << argv[2] << endl;
exit(1);
}
fout << "Enter operation + or - or * or / or q to quit. ";
fin >> c;
while (c != 'q') {
if (!((c == '+') || (c == '-') || (c == '*') || (c == '/')))
{
fout << "Illegal Operation";
return 0;
}
fout << "Enter x. ";
fin >> x;
fout << "Enter y . ";
fin >> y;
switch (c) {
case '+': z = x + y;
break;
case '-': z = x - y;
break;
case '*': z = x * y;
break;
case '/': z = x / y;
break;
default:
fout << "Illegal op ";
}
fout << "Answer: ";
fout << z << " ";
fout << "Enter operation + or - or * or / or q to quit. ";
fin >> c;
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
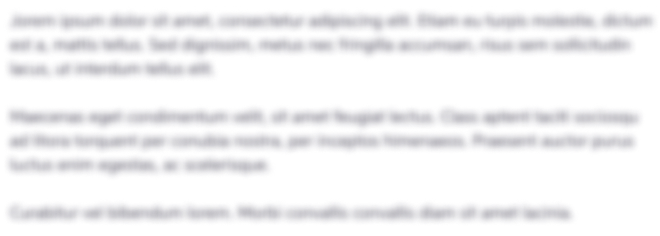
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started