Question
Modify the program below to have these additional features: Detect when a user clicks in the area on the screen where the third segment of
Modify the program below to have these additional features:
Detect when a user clicks in the area on the screen where the third segment of the flag would go, and draw that segment of the flag (the original program only supports the first two segments). In the original program, a section of the flag can be red, white, blue, or green. Add yellow. Whenever a user of the program creates an existing design, use messaging to indicate which country that flag represents. In all other cases, indicate to the user that the design is available. The program needs to be completed with the following six requirements:
1 - The functionality to detect a click in the third segment is present and working correctly.
2 - Yellow is present as a possible color for a section of flag, and the color cycling functionality works correctly.
3 - The six countries represented by specific flags designs are identified correctly in the messaging:
FRANCE: Blue, White, Red
ITALY: Green, White, Red
PERU: Red, White, Red
NIGERIA: Green, White, Green
ROMANIA: Blue, Yellow, Red
MALI: Green, Yellow, Red
4 - Flag designs not representative of the six countries above are displayed with messaging that reads, "That design is available."
5 - The onscreen messaging about the flag design is always clear and legible, correct, and font is consistently 20 pixel Arial. (You don't have to change the font size of the initial messaging that says, "Click anywhere to begin designing a flag.") In order to erase the current message before writing the new one, it will be necessary to complete the erase_message() function.
6 - The Python solution is easy to understand and does not contain unnecessary code. Remove the #TODO tags once they are complete, and add comments of your own to help explain what your code is doing.
import turtle width = 800 height = 500 turtle.setup(width, height) wn = turtle.Screen() wn.bgcolor("lightgray") draw_color = "gray" color_1, color_2, color_3 = draw_color, draw_color, draw_color section = turtle.Turtle() section.color(draw_color, "lightgray") section.shapesize(2) section.speed(0) section.penup() message = turtle.Turtle() message.hideturtle() message.penup() message.goto(-100, -150) message.color("gray") message.speed(0) message.write("Click anywhere to begin designing a flag.", font = ("Arial", 12)) def draw_section(): section.begin_fill() section.pendown() for i in range(2): section.forward(100) section.right(90) section.forward(200) section.right(90) section.end_fill() section.penup() section.goto(-250, 0) def draw_first(): section.goto(-150,100) draw_section() def draw_second(): section.goto(-50,100) draw_section() def draw_third(): # TODO: remove 'pass,' and draw the third section of the flag ... pass def cycle_color(current_color): if current_color == "green" or current_color == "gray" : new_color = "white" elif current_color == "white": new_color = "blue" elif current_color == "blue": new_color = "red" elif current_color == "red": new_color = "green" # TODO: add yellow to the color cycle else: # (note: we shouldn't ever get here...) print("There seems to be a mistake with the color cycling.") new_color = "gray" return new_color def color_flag(x, y): # Whenever we try to change a variable defined outside the function, # we need to use the global keyword to let Python know it's on purpose. global draw_color, color_1, color_2, color_3 # This print statment will tell us where on the screen the user clicked. print("x: ", x, "y: ", y) if (-150 < x < -50) and (100 > y > -100): draw_first() color_1 = draw_color elif (-50 < x < 50) and (100 > y > -100): draw_second() color_2 = draw_color # TODO: if user clicks where the third segment goes, call draw_third(), # and set the color_3 variable to store the current value of draw_color # The third segment should be the same size as the other two, and to the right else: draw_color = cycle_color(draw_color) section.color(draw_color) check_flag(color_1, color_2, color_3) # function to clear the previous message by drawing a box over it def erase_message(): message.fillcolor("lightgray") message.begin_fill() message.goto(-300, -120) #TODO: draw a 600 wide by 40 pixel high box here message.end_fill() message.goto(-100, -150) def check_flag(one, two, three): print("left: " + one + ", center: " + two + ", right: " + three) erase_message() # TODO: # Use if, elif, else (ie: selection or branching) statements # to display a message to the user: "National Flag of," # when a particular flag design is repersentative of one of these countries... # FRANCE: Blue, White, Red # ITALY: Green, White, Red # PERU: Red, White, Red # NIGERIA: Green, White, Green # ROMANIA: Blue, Yellow, Red # MALI: Green, Yellow, Red # ...otherwise, display a message that the design is available. # if any of the three sections are gray, prompt the user to color them if (one == "gray" or two == "gray" or three == "gray"): message.write("Color all three sections.", font = ("Arial", 20)) draw_first() draw_second() draw_third() wn.onclick(color_flag)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
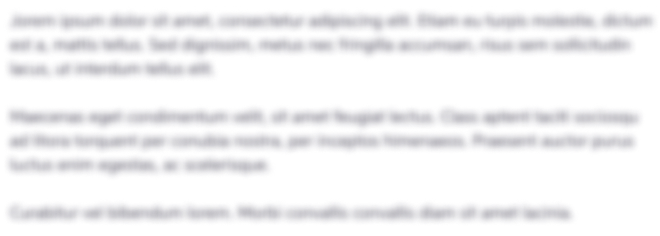
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started