Question
Modify the program so that, rather than printing data directly to the screen, it will read the data into an array of car structs and
Modify the program so that, rather than printing data directly to the screen, it will read the data into an array of car structs and then print out the contents of the array.
#include "car.h" #include#include #include #include
Car GetCar(ifstream& dataIn);
// Pre: File dataIn has been opened.
// Post: The fields of car are read from file dataIn.
void WriteCar(ofstream& dataOut, Car car);
// Pre: File dataOut has been opened.
// Post: The fields of car are written on file dataOut,
// appropriately labeled.
int main ()
{
Car car;
ifstream dataIn;
ofstream dataOut;
dataIn.open("cars.dat");
dataOut.open("cars.out");
cout << fixed << showpoint;
car = GetCar(dataIn);
while (dataIn)
{
car.price = car.price * 1.10f;
WriteCar(dataOut, car);
car = GetCar(dataIn);
}
return 0;
}
//*****************************************************
Car GetCar(ifstream& dataIn)
{
Car car;
dataIn >> car.customer;
dataIn >> car.price >> car.purchased.day
>> car.purchased.month >> car.purchased.year;
dataIn.ignore(2, ' ');
return car;
}
//*****************************************************
void WriteCar(ofstream& dataOut, Car car)
{
dataOut << "Customer: " << car.customer << endl
<< "Price: " << car.price << endl
<< "Purchased:" << car.purchased.day << "/"
<< car.purchased.month << "/"
<< car.purchased.year << endl;
}
.h file
#includestruct PersonType { std::string firstname; std::string lastname; }; struct Date { int month; int day; int year; }; struct Car { float price; Date purchased; PersonType customer; };
Step by Step Solution
There are 3 Steps involved in it
Step: 1
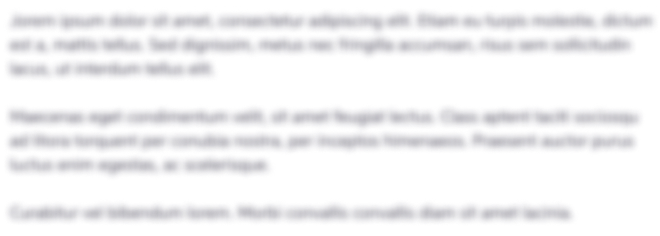
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started