Question
Modify the radix sort to receive an array of Strings of various lengths. Do not modify the Strings. DO NOT add any additional data structures
Modify the radix sort to receive an array of Strings of various lengths. Do not modify the Strings. DO NOT add any additional data structures to the method (this includes additional arrays).
public static void radixSortSameLength(String[] arr, int maxLength)
{
//number of buckets (256 in standard character set)
int bucketCount = 256;
//buckets can't be counters, need to be lists
List
//create all buckets needed
for(int i = 0; i < buckets.length; i++)
{
buckets[i] = new LinkedList<>();
}
//loop from end of string to beginning to properly sort
for(int currChar = maxLength-1;currChar >= 0; currChar--)
{
//loop through each string
for(int i = 0; i < arr.length; i++)
{
//add to appropriate bucket
buckets[arr[i].charAt(currChar)].add(arr[i]);
//arr[i].charAt(currChar) converts to the ASCII number automatically
}
//loop through buckets
int pos = 0;
for(int i = 0; i < buckets.length; i++)
{
//put each item from the bucket into original array
for(String item : buckets[i])
{
arr[pos] = item;
pos++;
}
//clear bucket
buckets[i].clear();
}
//System.out.println(Arrays.toString(arr));
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
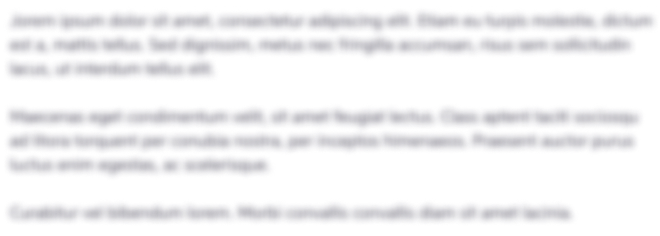
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started