Question
Modify the Tree class from hw6D to implement the Comparable interface from the Java library. Do not write your own Comparable interface. If you need
Modify the Tree class from hw6D to implement the Comparable interface from the Java library. Do not write your own Comparable interface.
If you need a working version of the Tree class, you may use this solution.
Trees are ordered first by height with the smallest coming first. If two Trees have the same height, the Trees are ordered alphabetically by type in lexicographical order. If this Tree should come before the other tree , compareTo returns a negative integer. If this Tree should come after the other Tree, it returns a positive integer. Otherwise, it returns 0.
Remember about using Double.compare to compare doubles.
Note: The compareTo method takes an Object as the parameter, not a Tree. (That means you will have to cast it.)
The header for compareTo is public int compareTo(Object otherObject).
Note: You will get a compiler warning about "unsafe or unchecked operations" when you compile TreeRunner. You can safely ignore it for now.
public class Tree { private double height; private String type; /** * Constructor for objects of class Tree * @param myType type of the tree * @param myHeight height of the tree */ public Tree(String myType, double myHeight) { height = myHeight; type = myType; } /** * Set the type of the tree * @param myType type of the tree */ public void setType(String myType) { type = myType; } /** * Set the height of the tree * @param myHeight height of the tree */ public void setHeight(double myHeight) { height = myHeight; } /** * Gets the type the tree * @return the type of the tree */ public String getType() { return type; } /** * Gets the height the tree * @return the height of the tree */ public double getHeight() { return height; } }
Testers:
import java.util.Collections; import java.util.ArrayList; /** * */ public class TreeRunner { public static void main(String[] args) { ArrayListtrees = new ArrayList<>(); trees.add(new Tree("avocado", 35.0)); trees.add(new Tree("dwarf maple tree", 4.0)); trees.add(new Tree("lemon",12.5 )); trees.add(new Tree("apple", 20.0)); trees.add(new Tree("cherry", 12.5)); trees.add(new Tree("apricot", 17)); Collections.sort(trees); //They will still be in original order for the draft - not sorted //since campareTo is a stub for(Tree t : trees) { System.out.println(t.getType() + " " + t.getHeight()); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
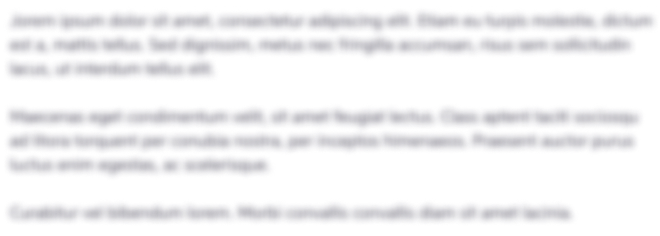
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started