Question
modify this code to meet the requirements PROGRAM TO DEMONSTRATE MULTITHREADING ECHO SERVER import java.io.*; import java.net.*; import java.util.*; public class MultiEchoServer { private static
modify this code to meet the requirements
PROGRAM TO DEMONSTRATE MULTITHREADING ECHO SERVER
import java.io.*;
import java.net.*;
import java.util.*;
public class MultiEchoServer
{
private static ServerSocket servsoc;
private static final int PORT = 1234;
public static void main(String[] args) throws IOException
{
try
{
servsoc = new ServerSocket(PORT);
}
catch (IOException ioEx)
{
System.out.println(" Unable to set up port!");
System.exit(1);
}
System.out.println(" Waiting for Client. ");
do
{
//Wait for client
Socket client = servsoc.accept();
System.out.println(" New client accepted. ");
//Create a thread to handle communication with this client and pass the constructor for //this thread a reference to the relevant socket
ClientHandler handler = new ClientHandler(client);
handler.start();
}while (true);
}
}
class ClientHandler extends Thread
{
private Socket client;
private Scanner input;
private PrintWriter output;
public ClientHandler(Socket soc)
{
//Set up reference to associated socket
client = soc;
try
{
input = new Scanner(client.getInputStream());
output = new PrintWriter(client.getOutputStream(),true);
}
catch(IOException ioEx)
{
ioEx.printStackTrace();
}
}
public void run()
{
String received;
do
{
//Accept message from client on the socket's input stream
received = input.nextLine();
//Echo message back to client on the socket's output stream
output.println("ECHO: " + received);
//Repeat above until 'QUIT' sent by client
}while (!received.equals("QUIT"));
try
{
if (client!=null)
{
System.out.println("client disconnected");
client.close();
}
}
catch(IOException ioEx)
{
System.out.println("Unable to disconnect!");
}
}
}
----------
PROGRAM TO DEMONSTRATE MULTITHREADING ECHO CLIENT
import java.io.*;
import java.net.*;
import java.util.*;
public class MultiEchoClient
{
private static InetAddress host;
private static final int PORT = 1234;
public static void main(String[] args)
{
try
{
host = InetAddress.getLocalHost();
}
catch(UnknownHostException uhEx)
{
System.out.println(" Host ID not found! ");
System.exit(1);
}
sendMessages();
}
private static void sendMessages()
{
Socket soc = null;
try
{
soc = new Socket(host,PORT);
Scanner networkInput =
new Scanner(soc.getInputStream());
PrintWriter networkOutput = new PrintWriter(soc.getOutputStream(),true);
//Set up stream for keyboard entry
Scanner userEntry = new Scanner(System.in);
String message, response;
do
{
System.out.print(
"Enter message ('QUIT' to exit): ");
message = userEntry.nextLine();
//Send message to server on the socket's output stream
//Accept response from server on the socket's intput stream
networkOutput.println(message);
response = networkInput.nextLine();
//Display server's response to user
System.out.println(" SERVER> " + response);
}while (!message.equals("QUIT"));
}
catch(IOException ioEx)
{
ioEx.printStackTrace();
}
finally
{
try
{
System.out.println(" Closing connection");
soc.close();
}
catch(IOException ioEx)
{
System.out.println("Unable to disconnect!");
System.exit(1);
}
}
}
}
-------------
this is the question
Write a Multithread Echo Client Server Program to display the following output.
Network Programming - JAVA
NOTE: the output will be in CMD ( Command Prompt )
Server Program Output:
i) When you run the server program, the following text must be displayed.
Student-ID started Multithread Echo Server on port number : xxxx |
ii) If the client closes the connection by typing end, then the following text must be displayed at
the server.
Student-ID the Client Closed the Connection |
iii) When you run the server program and if you did not enter the port number at run time,
the following text must be displayed.
You forgot to enter the port number-Student-ID |
Client Program Output:
i) When you run the client program, the following text must be displayed.
Student-ID started Multithread Echo Client. Type end to close the Client |
ii) When the client wants to send a message to the server, the following text must be displayed at
client.
Student-ID Please Enter Message for Server : xxxxxxx |
iii) When the server echoes the client message, the following text must be displayed at client.
Server Echoed this Message Student-ID : xxxxxx |
iv) When the client types end, the server echo message must not be displayed instead the
following text must be displayed.
Student-ID Client wants to close |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
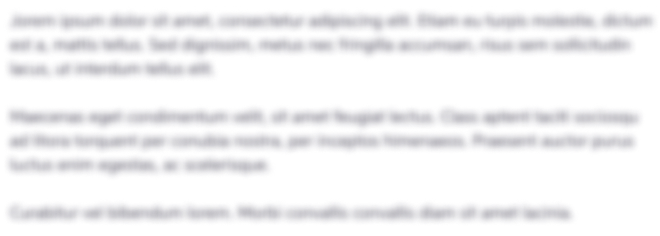
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started