Question
Modify this java code below so it takes in the string instead of the number. public class Question1 { private String text; private String answer;
Modify this java code below so it takes in the string instead of the number.
public class Question1
{
private String text;
private String answer;
public Question1()
{
text = "";
answer = "";
}
public void setText(String questionText)
{
text = questionText;
}
public void setAnswer(String correctResponse)
{
answer = correctResponse;
}
public boolean checkAnswer(String response)
{
return response.equals(answer);
}
public void display()
{
System.out.println(text);
}
}
import java.util.ArrayList;
public class ChoiceQuestion1 extends Question1
{
private ArrayList
public ChoiceQuestion1()
{
choices = new ArrayList
}
public void addChoice(String choice, boolean correct)
{
choices.add(choice);
if (correct)
{
// Convert choices.size() to string
String choiceString = "" + choices.size();
setAnswer(choiceString);
}
}
public void display()
{
// Display the question text
super.display();
// Display the answer choices
for (int i = 0; i < choices.size(); i++)
{
int choiceNumber = i + 1;
System.out.println(choiceNumber + ": " + choices.get(i));
}
}
}
import java.util.Scanner;
public class QuestionDemo2
{
public static void main(String[] args)
{
ChoiceQuestion1 first = new ChoiceQuestion1();
first.setText("What was the original name of the Java language?");
first.addChoice("*7", false);
first.addChoice("Duke", false);
first.addChoice("Oak", true);
first.addChoice("Gosling", false);
ChoiceQuestion1 second = new ChoiceQuestion1();
second.setText("In which country was the inventor of Java born?");
second.addChoice("Australia", false);
second.addChoice("Canada", true);
second.addChoice("Denmark", false);
second.addChoice("United States", false);
presentQuestion(first);
presentQuestion(second);
}
public static void presentQuestion(ChoiceQuestion1 q)
{
q.display();
System.out.print("Your answer: ");
Scanner in = new Scanner(System.in);
String response = in.nextLine();
System.out.println(q.checkAnswer(response));
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
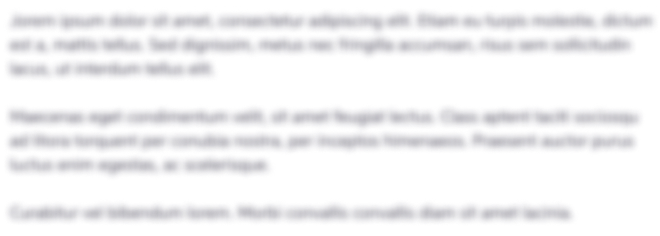
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started