Question
Module 6 - Assignment Using/Writing Classes Using/Writing Classes Assignment To fully understand all of the requirements for the program you will write for this assignment
Module 6 - Assignment Using/Writing Classes
Using/Writing Classes Assignment
To fully understand all of the requirements for the program you will write for this assignment read this entire page, but also study each of the four execution logs linked below. Reading all of these documents will enable you to see what will be required in each of the three classes you will write and submit:
EyesHaveIt.java, PairOfDice.java, and Die.java.
Before you begin writing your Java code, design your algorithms for the various parts. This is a way to save time both in implementation and in debugging. Before doing any coding be sure to review the REMEMBER section at the end of this document, as well as all coding conventions, especially those pertaining to writing classes.
Overview of the Game
In this assignment you will write a dice game called Eyes Have It. You must write a class named, EyesHaveIt.java, that will contain the logic to play the game with two players: the computer and a human player. The computer begins by taking its turn, followed by the human player taking his/her turn. When the game is done, both players will have had the same number of turns.
A turn consists of rolling a pair of six-sided dice at least once, but no more than five times. The computer will automatically stop rolling if its turn total reaches 20 points (the sum of the dice rolls in that turn). The human will be prompted as to whether another roll is desired and may answer Y or y four times in a turn to get a maximum of five turns. For each player the turn score or turn total is sum of both dice on all rolls taken in that turn. A player's game score will be the sum of all the turn totals for all turns taken in the game.
If double sixes (box cars) are rolled, then that player will lose the current turn and ALL points are lost (both current turn points and all game points accumulated so far).
If double ones (snake eyes) are rolled once, 2 points are added to the turn points and all turn points are then doubled at the end of the current turn. If a second snake eyes is rolled in the same turn, 2 more points are added to the turn total and then all turn points are multiplied by 4 at the end of the current turn. This logic continues, so that if snakes eyes were rolled on each of a players five rolls in one turn, the turn total would be 10, but this would be multiplied by 32 (2 to the 5th power) to yield a turn total of 320! LogGame 4 attached to this assignment shows this event actually happening!
If any other double is rolled (besides 6's and 1's), that player will lose the turn AND all points accumulated during this turn. No game points are lost in this case.
The first player to 150 after both players have had the same number of turns is the winner. If both players are over 150, the one with the higher total wins. If both players have more than 150 points and the same number of points, another turn must be played for each player.
The Classes You Must Write
You are given the application class, PlayGame.java - available for download here. This application class will be executed by the JVM and will use the three classes you will write: EyesHaveIt class, which will use the PairOfDice class, which will use the Die class. Each of these is described below.
EyesHaveIt.java - As you can see from the code in PlayGame.java, this class has a method named, init, which accepts a String, and another method named, playGame. Both methods are void. The design of these methods and the other methods that these may invoke is your assignment. You may add other methods as you expand your design to play the game according to the rules in this document. Recall that you are doing an object-oriented design. This implies that the only variables that will be defined at the class level will be attributes of the game object. Methods within this class should communicate by passing parameters and returning values, not by using variables at the class level for this purpose. Examples of the EyesHaveIt object's attributes are the game scores for each player, the name of the human player, and the PairOfDice object used throughout the game by both players. Your design should NOT cause any other variables to be defined at the class level in this class. Note that this class uses one PairOfDice object and does NOT directly use the Die class.
PairOfDice.java - This class will be an abstraction of a physical pair of standard six-sided dice. Only one object of this class will be created by the EyesHaveIt object and used throughout the game by both players. You should consider this object to be a pair of dice. When a player rolls during a turn, that player is rolling the PairOfDice object. Players never roll a Die object directly. The client of the PairOfDice class will roll the PairOfDice object, get the values of each die in the pair, etc. This class should have exactly two attributes: two objects of the class, Die. This is the only class that uses the Die class.
Die.java - This class is an abstraction of one standard six-sided die. A class similar to this is given in Chapter 6 of the text and you may base your design on that example. HOWEVER, it is not a good idea to create the Random object inside the roll method as is done in the book. An application will experience more randomness by creating the Random object less often. Besides that, there really is no need to recreate this object every time the dice are rolled. Therefore, the best design is to create a static Random object at the class level in the Die class. This way only one Random object is created per game and, by making this object static, both Die objects will use the same Random object to generate its rolls. So, create your Random object at the class level (inside the class but outside of or before the main method) in the Die class as follows:
public static Random generator = new Random();
Of course, you can name the object whatever you choose. Remember to import java.util.Random.
When you submit this assignment, you should submit three Java files named exactly as follows:
EyesHaveIt.java
PairOfDice.java
Die.java
Example Game Logs to Help You Understand the Game
Four separate logs are available for you to review to help you fully understand how the game works. Log1 has a few annotations to help you understand the game flow shown in all f the logs. Email your instructor if you have any questions.
Log1 Log2
Log3
Log4
REMEMBER:
All coding conventions! Each Java file should have its own prologue. Each method in a Java class must have a method prologue describing the purpose of the method, including a description of the method's formal parameters and its return value. Do not use ANY Java features NOT covered in the first six chapters of the text. Do not use any JOptionPane elements.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
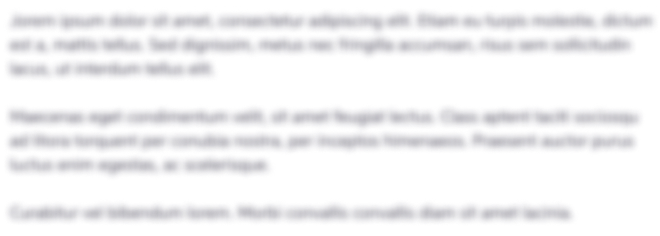
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started