Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Module to check violations for a flight lesson. import utils import pilots import os . path from datetime import datetime, timezone, timedelta
Module to check violations for a flight lesson.
import utils
import pilots
import ospath
from datetime import datetime, timezone, timedelta
import pytz
# WEATHER FUNCTIONS
def badvisibilityvisibilityminimum:
Returns True if the visibility measurement violates the minimum, False otherwise
if visibility "unavailable":
return True
# Check if visibility is a dictionary with 'prevailing' and 'units' keys
if
isinstancevisibility dict
and "prevailing" in visibility
and "units" in visibility
:
prevailingvisibility visibilityprevailing
units visibilityunits
# Convert visibility to statute miles if the units are in FTfeet
if units FT:
prevailingvisibility
prevailingvisibility
# statute mile feet
# Check if 'minimum' exists in the dictionary, if not, use 'prevailing' as minimum
if "minimum" in visibility:
minimumvisibility visibilityminimum
if units FT:
minimumvisibility minimumvisibility
return minimumvisibility minimum
else:
return prevailingvisibility minimum
return False # Invalid visibility format
pass # Implement this function
def badwindswindsmaxwind,maxcross:
Returns True if the wind measurement violates the maximums, False otherwise
if winds "unavailable":
return True
if winds "calm":
return False
if typewinds is not dict: # Not what we were expecting, missing weather data
return True
# Only 'speed' and 'units' are required
if "speed" not in winds or "units" not in winds:
return True
# Declare speed variable
speed windsspeed
if "gusts" in winds:
speed maxspeed windsgusts
# Declare crosswind variable
crosswind None
if "crosswind" in winds:
crosswind windscrosswind
# If units are different
if windsunitsMPS:
if crosswind None:
crosswind crosswind # convert to KT
speed speed
# This function returns True if speed is more than the maximum
if speed maxwind:
return True
# This function should compare ths winds 'crosswind' if it exists
if crosswind None and crosswind maxcross:
return True
return False
pass # Implement this function
def badceilingceilingminimum:
if ceiling 'clear':
return False
if ceiling 'unavailable':
return True
for x in ceiling:
if xtype 'broken':
if xheight minimum:
return True
else:
return False
if xtype 'overcast':
if xheight minimum:
return True
else:
return False
if xtype 'indefinite ceiling':
if xheight minimum:
return True
else:
return False
return False
pass # Implement this function
def getweatherreporttakeoffweather:
isotakeoff takeoff.isoformat
if isotakeoff in weather.keys:
return weatherstrisotakeoff
for k in weather.keys :
stringweatherk
temptimeutils.strtotime stringweathertakeoff.tzinfo
if temptime takeoff:
return weatherk
return None
pass
def getweatherviolationweatherminimums:
Returns a string representing the type of weather violation empty string if flight is ok
if weather is None:
return 'Unknown'
currentvisibility weather.getvisibilitygetminimum
currentwindspeed weather.getwindgetspeed
currentheight weather.getskygetheight
problems
if currentvisibility is not None and badvisibilitycurrentvisibility, minimums:
problems.appendVisibility
if currentwindspeed is not None and badwindsweathergetwind minimums minimums:
problems.appendWinds
if currentheight is not None and badceilingcurrentheight, minimums:
problems.appendCeiling
if lenproblems:
return 'Weather'
elif lenproblems:
return problems
else:
return # if there is only one problemImplement getweatherviolation
violations.badwind passed all tests
violations.badceiling passed all tests
violations.getweatherreport passed all tests
violations.getweatherviolation passed all tests
Again, you will notice that it will take several seconds to complete the test of
getweatherreport. This is a minor inconvenience for the rest
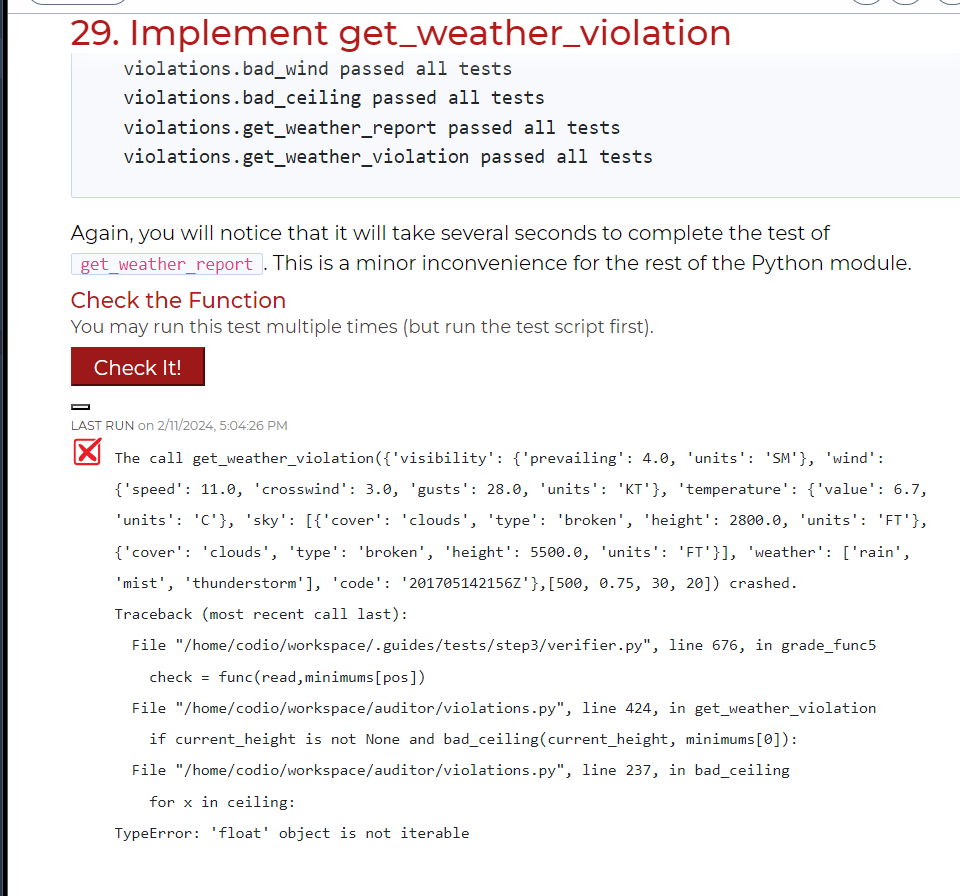
Step by Step Solution
There are 3 Steps involved in it
Step: 1
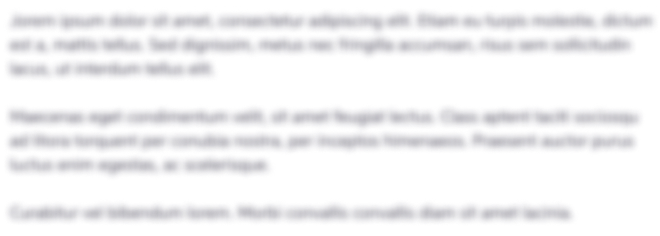
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started