Question
mplement a Max Heap class. Complete client.cpp, maxHeap.h, and maxHeap.cpp. //client.cpp #include #include maxHeap.h using namespace std; int main() { MaxHeap h(11); h.insert(3); h.insert(2); h.insert(15);
mplement a Max Heap class. Complete client.cpp, maxHeap.h, and maxHeap.cpp.
//client.cpp
#include
using namespace std;
int main() { MaxHeap h(11); h.insert(3); h.insert(2); h.insert(15); h.insert(5); h.insert(4); h.insert(45); h.removeAt(2); cout << h.extractMax() << " "; cout << h.getMax() << endl; int a[7] = {2, 3, 4, 5, 6, 7, 1}; MaxHeap h2(7); h2.heapify(a, 7); cout << h2.extractMax() << " "; cout << h2.getMax() << " " << endl; return 0; }
----------------------------------------------------------------------------------------------------------------------
//maxHeap.h
#ifndef HEAP_H #define HEAP_H class MaxHeap { int *arr; // pointer to array of elements in heap int capacity; // maximum possible size of min heap int size; // Current number of elements in min heap int parent(int i); int left(int i); int right(int i); bool isLeaf(int i); void siftup(int i); void siftdown(int i); public: class Overflow{}; class Underflow{}; MaxHeap(int capacity); int getSize(); int getMax(); void insert(int k); int extractMax(); int removeAt(int i); void heapify(int *array, int len); }; #endif
----------------------------------------------------------------------------------------------------------------
//maxHeap.cpp
#include "maxHeap.h"
/*MaxHeap::MaxHeap(int cap) { ;; }*/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
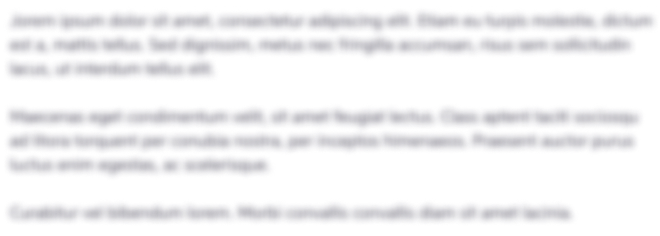
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started