Question
multiply and adding two polynomials using single linked list in java I have figured it out adding two polynomials using single linked list, the codes
multiply and adding two polynomials using single linked list in java
I have figured it out adding two polynomials using single linked list, the codes below is complied and executable for adding. please use same interface as i used in adding part to creating multiply part.
import java.util.*;
import java.text.*;
public class Polynomial1
{
private Monomial1 head1;
private double TOLERANCE1 = 0.00000001;
private class Monomial1
{
private DecimalFormat precision1 = new DecimalFormat("#.####");
private int exp1; //exp1onent for coeff1icient is initialised
private double coeff1;
private Monomial1 next1;
public Monomial1(int exp1, double coeff1, Monomial1 next1)
{
this.exp1 = exp1;
this.coeff1 = coeff1;
this.next1 = next1;
}
public String toString()
{
String form = precision1.format(Math.abs(coeff1));
if(exp1 == 0) return form ;
else
if(exp1 == 1) return form + "*x";
else
return form +"*x^" + exp1;
}
public boolean equals(Monomial1 mono)
{
return exp1 == mono.exp1 && coeff1 == mono.coeff1;
}
}
public Polynomial1()
{
head1 = null;
}
public void addTerm1(int exp1, double coeff1)//addition can be calculated
{
if( Math.abs(coeff1) < TOLERANCE1 ) return;
if( head1 == null || exp1 < head1.exp1 )
{
head1 = new Monomial1(exp1, coeff1, head1);
return;
}
Monomial1 cur1 = head1;
Monomial1 prev1 = null;
while( cur1 != null && exp1 > cur1.exp1)
{
prev1 = cur1;
cur1 = cur1.next1;
}
if( cur1 == null || exp1 != cur1.exp1 )
prev1.next1 = new Monomial1(exp1, coeff1, cur1);
else
{
cur1.coeff1 += coeff1;
if( Math.abs(cur1.coeff1) < TOLERANCE1 )
if(prev1 != null)
prev1.next1 = cur1.next1;
else
head1 = head1.next1;
}
}
public String toString()//to string method
{
//instance for string buffer
StringBuffer sb1 = new StringBuffer();
for(Monomial1 tmp1 = head1; tmp1 != null; tmp1 = tmp1.next1)
if(tmp1.coeff1 < 0 )
sb1.append(" - " + tmp1.toString());
else
sb1.append(" + " + tmp1.toString());
return sb1.toString();
}
public Polynomial1 add1(Polynomial1 poly)
{
Polynomial1 res1 = clone();
for(Monomial1 tmp1 = poly.head1; tmp1 != null; tmp1 = tmp1.next1)
res1.addTerm1(tmp1.exp1, tmp1.coeff1);
return res1;
}
public Polynomial1 clone()
{
Polynomial1 res1 = new Polynomial1();
for(Monomial1 tmp1 = head1; tmp1 != null; tmp1 = tmp1.next1)
res1.addTerm1(tmp1.exp1, tmp1.coeff1);
return res1;
}
public static void main(String[] args)//main method
{
Polynomial1 first1 = new Polynomial1();//first instance for the class
Polynomial1 second1 = new Polynomial1();//second instance for the class
System.out.println( "FIRST" );
first1.addTerm1(5, 1);//passing values
first1.addTerm1(2, 2);
first1.addTerm1(3, 3);
first1.addTerm1(4, 4);
System.out.println( first1 );
System.out.println( );
System.out.println( "SECOND" );
second1.addTerm1(2, 2);
second1.addTerm1(1, 4);
System.out.println( second1 );
System.out.println( );
System.out.println( "ADD FIRST AND SECOND" );
Polynomial1 third1 = first1.add1(second1);//third instance for the class
System.out.println( third1 );
System.out.println( );
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
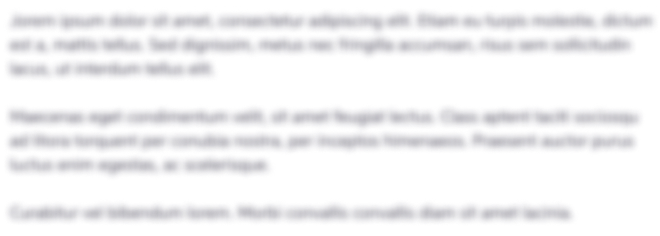
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started