Question
Music.h Main.c __________________________________________________________________________________ #include #include #include music.h /* FUNCTION PROTOTYPES */ void sys_init(void); void delayby1ms(unsigned int); /* FUNCTION DEFINITIONS */ void main(void) { unsigned char
Music.h
Main.c
__________________________________________________________________________________
#include
#include
#include "music.h"
/* FUNCTION PROTOTYPES */
void sys_init(void);
void delayby1ms(unsigned int);
/* FUNCTION DEFINITIONS */
void main(void)
{
unsigned char newkey = 0; /* P7 key state variables */
unsigned char oldkey = 0; /* " */
unsigned char note_h = ZH; /* initial values for note frequency/duration */
unsigned char note_l = ZL; /* " */
sys_init(); /* configure hardware and peripherals */
SFRPAGE = UART0_PAGE; /* select UART SFR page; print welcome */
printf(" *** NEW PLAYER PIANO SESSION *** ");
/* MAIN LOOP */
while (1) {
SFRPAGE = 0x0F; /* select P7 SFR page */
newkey = P7; /* get state of P7 keys (P7.0 - P7.7) */
if (newkey != oldkey) /* key pressed/released? */
{
oldkey = newkey; /* if so, save new key code */
// INSERT YOUR CODE HERE
/* LOAD AND PRINT NEW NOTE VALUES */
SFRPAGE = UART0_PAGE; /* switch to UART SFR page for console output */
switch ( newkey ) { /* select new note value */
case 0x80:
printf("C4 ");
note_h = C4H; /* C4 */
note_l = C4L; /* " */
break;
// INSERT YOUR CODE HERE
default:
printf("| ");
note_h = ZH; /* silence */
note_l = ZL; /* " */
} /* end switch() */
SFRPAGE = TMR2_PAGE; /* switch to T2 SFR page for timer/buzzer */
/* BEGIN PLAYING NEW NOTE */
// INSERT YOUR CODE HERE
} /* end if() */
} /* end main loop */
} /* end main() */
void delayby1ms(unsigned int k)
{
unsigned char p;
unsigned int i;
p = SFRPAGE; /* save previous SFR page */
SFRPAGE = 0; /* switch to T0 page */
for (i = 0; i
TH0 = 0xEB; /* place 59410 in TH0:TL0 so T0 overflows in 1 ms */
TL0 = 0x12;
TF0 = 0;
TR0 = 1; /* start Timer 0 */
while(!TF0); /* wait for 1 ms */
TR0 = 0; /* stop Timer 0 */
}
SFRPAGE = p; /* restore previous SFR page */
}
void sys_init(void)
{
SFRPAGE = 0x0F; /* switch to SFR configuration page (F) */
WDTCN = 0xDE; /* disable watchdog timer */
WDTCN = 0xAD; /* " */
CLKSEL = 0; /* select internal oscillator as SYSCLK */
OSCICN = 0x83; /* " */
XBR0 = 0xF7; /* assign all peripheral signals to port pins */
XBR1 = 0xFF; /* " */
XBR2 = 0x5D; /* " */
XBR3 = 0x8F; /* " */
P2MDOUT = 0x80; /* enable T2 pin output */
P7MDOUT = 0xFF; /* enable P7 as push-pull output */
P6MDOUT = 0xFF; /* enable P6 as push-pull output */
P7 = 0;
P6 = 0;
SFRPAGE = TMR4_PAGE; /* Configure Timer 4 for UART */
RCAP4H = 0xFF; /* set UART0 baud rate to 19200 */
RCAP4L = 0xB2; /* " */
TMR4H = 0xFF; /* " */
TMR4L = 0xB2; /* " */
TMR4CF = 0x08; /* select SYSCLK as TMR4's clock source */
TMR4CN = 0x04; /* select Timer 4 reload mode */
SFRPAGE = TMR2_PAGE; /* Configure Timer 2 */
TMR2CF = 0x0A; /* select SYSCLK as timer 2 clock, enable T2 output */
TMR2CN = 0;
SFRPAGE = UART0_PAGE; /* switch to SFR page 0 */
SCON0 = 0x50; /* UART0 in mode 1, enable reception */
SSTA0 = 0x0F; /* use TMR4 to generate UART0 baud rate */
TI0 = 1; /* get ready to transceive */
RI0 = 0; /* get ready to receive */
TMOD = 0x11; /* configure Timer 0 and 1 to mode 1 */
CKCON = 0x01; /* Timer 0 use system clock divided by 4 as clock source */
SPI0CN = 0x01; /* enable SPI in 3-wire mode */
}
This needs to be done in c language.
This lab assignment explores the use of the C8051F040 microcontroller's built-in timers and counters, and the use of polling to receive input through the I/O ports. In this first part of Lab #3, you will be designing a simple "electronic piano" which plays the notes of the C-major scale when the user presses the buttons corresponding to Port 7 (the top row of buttons in the BIG8051 Development System's button array). In Part Two of this assignment, to be posted next week, you will be expanding this program into a "player piano" which is able to record and play back the music entered by the user. To begin this assignment, download the attached archive, "Lab03.zip". Extract the archive into a new folder, then create a new Silicon Labs IDE project in the same folder and add the header and program files from the archive to the project and to the build. Open the file "main.c", and you will find a short program which contains a partial implementation of this assignment. Refer to the attached instructions for the necessary background information to complete this assignment (which is also discussed in the lecture notes), as well as setup instructions for enabling the piezo buzzer on the BIG8051. Begin by opening and studying the main.c file. It is an incomplete implementation of the "electronic piano program. Notice that it begins by declaring four variables: newkey and oldkey, which will hold the current and previously-entered button presses, and note_1 and note_h, which will hold the high and low bytes of the next note (or rest) to be played. Next, it initializes the hardware and prepares Timer 2 for use, and then switches to SFR Page Ox0F so it can read the buttons from the register P7. Inside the main loop, it reads the input code from P7 into newkey. This code represents the state of the top row of buttons in the button array: if no button is pressed, newkey will have a value of zero, and if one or more buttons are pressed, their corresponding bits in newkey will be set to one. For example, if the leftmost key in the top row is being pressed, newkey will have a value of 0x80 (or 10000000 in binary). After getting the current input code, the program compares it to the previous input code to determine if the state of the buttons has changed (this would indicate that the user has pressed or released a button since the last time they were read). If a change has occurred, the old input code is replaced with the new one. At this point, you will notice the first of several lines containing the comment INSERT YOUR CODE HERE. These comments indicate where you will need to write the code which will complete the program. Your code will need to perform the following steps (note: some of the steps outlined here are already included in the main.c" program file): 1. Switch back to SFR Page 0x0F, so that with each repetition of the main loop, the program can read the state of P7 again. 2. Switch to SFR Page TMR2_PAGE (to provide access to the timer registers). 3. Disable Timer 2 (assign a value of zero to the register TR2, as shown in the sample code). 4. Compare the value of newkey to each of the eight possible bit patterns that can be created by pressing the eight buttons in the top row. For each pattern, copy the low and high bits of the corresponding note value into the variables note_1 and note_h. Use the definitions given in the music.h header. The sequence of notes that you should assign to each button correspond to the notes of the C major scale: C4, D4, E4, F4, G4, A4, B4, and C5 (commonly sung as do, re, mi, fa, so, la, ti, do), in that order. (For example, if the user presses the leftmost button on the keypad, the value of newkey will be 0x80, and your program should copy the value of C4L into note_1 and the value of C4H into note_h. Or, if the user presses the rightmost button, the value of newkey will be 0x01, and your program should copy the value of C5L into note_1 and the value of C5H into note_h.) I recommend that you use a switch statement for this step. Your switch statement should include a default case which copies ZL to note_1 and ZH to note_h (Z is an inaudible high-frequency value which will silence the buzzer). This way, if the user releases a button, or presses more than one button at the same time, the buzzer will be silenced. 5. Load the new sound values into the TMR2 and RCAP2 register pairs. (In other words, copy the value of note_1 into TMR2L and RCAP2L, and the value of note_h into TMR2H and RCAP2H.) 6. Re-enable Timer 2 (assign a value of one to the register TR2, as shown in the sample code). 1 G /* G3 */ 2 G3L B3H 3 /* B3 */ 4 B3L C4H /* C4 (do) */ 6 Lo 000 7 /* C4-sharp */ /* D4 (re) */ 10 11 /* E4 (mi) */ 12 13 /* F4 (fa) */ 14 15 /* F4-sharp */ 16 Judefine #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define C4L C4SH C4SL D4H D4L E4H E4L F4H F4L F4SH F4SL G4H G4L A4H A4L B4FH B4FL B4H B4L C5H C5L D5H 17 18 19 20 21 /* G4 (so) */ OXOB OxDC 0x Ox39 0x49 Ox1A Ox53 Ox5D Ox5D OxD Ox6E OxD5 Ox76 OxFB 0x7E OXAB 0x85 OxEE Ox93 0x3F Ox99 Ox59 Ox9F 0x1C OxA4 0x8D OXAE 0x87 OxB7 Ox6B OxBB Ox7D OxFF OXFA /* A4 (la) */ /* B4-flat */ 22 23 24 /* B4 (ti) */ 25 /* C5 (do) */ 26 /* D5 */ 27 28 D5L E5H /* E5 */ 29 30 31 32 /* F5 * E5L F5H F5L ZH 33 /* ZERO (an inaudible high-frequenc 34 ZL This lab assignment explores the use of the C8051F040 microcontroller's built-in timers and counters, and the use of polling to receive input through the I/O ports. In this first part of Lab #3, you will be designing a simple "electronic piano" which plays the notes of the C-major scale when the user presses the buttons corresponding to Port 7 (the top row of buttons in the BIG8051 Development System's button array). In Part Two of this assignment, to be posted next week, you will be expanding this program into a "player piano" which is able to record and play back the music entered by the user. To begin this assignment, download the attached archive, "Lab03.zip". Extract the archive into a new folder, then create a new Silicon Labs IDE project in the same folder and add the header and program files from the archive to the project and to the build. Open the file "main.c", and you will find a short program which contains a partial implementation of this assignment. Refer to the attached instructions for the necessary background information to complete this assignment (which is also discussed in the lecture notes), as well as setup instructions for enabling the piezo buzzer on the BIG8051. Begin by opening and studying the main.c file. It is an incomplete implementation of the "electronic piano program. Notice that it begins by declaring four variables: newkey and oldkey, which will hold the current and previously-entered button presses, and note_1 and note_h, which will hold the high and low bytes of the next note (or rest) to be played. Next, it initializes the hardware and prepares Timer 2 for use, and then switches to SFR Page Ox0F so it can read the buttons from the register P7. Inside the main loop, it reads the input code from P7 into newkey. This code represents the state of the top row of buttons in the button array: if no button is pressed, newkey will have a value of zero, and if one or more buttons are pressed, their corresponding bits in newkey will be set to one. For example, if the leftmost key in the top row is being pressed, newkey will have a value of 0x80 (or 10000000 in binary). After getting the current input code, the program compares it to the previous input code to determine if the state of the buttons has changed (this would indicate that the user has pressed or released a button since the last time they were read). If a change has occurred, the old input code is replaced with the new one. At this point, you will notice the first of several lines containing the comment INSERT YOUR CODE HERE. These comments indicate where you will need to write the code which will complete the program. Your code will need to perform the following steps (note: some of the steps outlined here are already included in the main.c" program file): 1. Switch back to SFR Page 0x0F, so that with each repetition of the main loop, the program can read the state of P7 again. 2. Switch to SFR Page TMR2_PAGE (to provide access to the timer registers). 3. Disable Timer 2 (assign a value of zero to the register TR2, as shown in the sample code). 4. Compare the value of newkey to each of the eight possible bit patterns that can be created by pressing the eight buttons in the top row. For each pattern, copy the low and high bits of the corresponding note value into the variables note_1 and note_h. Use the definitions given in the music.h header. The sequence of notes that you should assign to each button correspond to the notes of the C major scale: C4, D4, E4, F4, G4, A4, B4, and C5 (commonly sung as do, re, mi, fa, so, la, ti, do), in that order. (For example, if the user presses the leftmost button on the keypad, the value of newkey will be 0x80, and your program should copy the value of C4L into note_1 and the value of C4H into note_h. Or, if the user presses the rightmost button, the value of newkey will be 0x01, and your program should copy the value of C5L into note_1 and the value of C5H into note_h.) I recommend that you use a switch statement for this step. Your switch statement should include a default case which copies ZL to note_1 and ZH to note_h (Z is an inaudible high-frequency value which will silence the buzzer). This way, if the user releases a button, or presses more than one button at the same time, the buzzer will be silenced. 5. Load the new sound values into the TMR2 and RCAP2 register pairs. (In other words, copy the value of note_1 into TMR2L and RCAP2L, and the value of note_h into TMR2H and RCAP2H.) 6. Re-enable Timer 2 (assign a value of one to the register TR2, as shown in the sample code). 1 G /* G3 */ 2 G3L B3H 3 /* B3 */ 4 B3L C4H /* C4 (do) */ 6 Lo 000 7 /* C4-sharp */ /* D4 (re) */ 10 11 /* E4 (mi) */ 12 13 /* F4 (fa) */ 14 15 /* F4-sharp */ 16 Judefine #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define #define C4L C4SH C4SL D4H D4L E4H E4L F4H F4L F4SH F4SL G4H G4L A4H A4L B4FH B4FL B4H B4L C5H C5L D5H 17 18 19 20 21 /* G4 (so) */ OXOB OxDC 0x Ox39 0x49 Ox1A Ox53 Ox5D Ox5D OxD Ox6E OxD5 Ox76 OxFB 0x7E OXAB 0x85 OxEE Ox93 0x3F Ox99 Ox59 Ox9F 0x1C OxA4 0x8D OXAE 0x87 OxB7 Ox6B OxBB Ox7D OxFF OXFA /* A4 (la) */ /* B4-flat */ 22 23 24 /* B4 (ti) */ 25 /* C5 (do) */ 26 /* D5 */ 27 28 D5L E5H /* E5 */ 29 30 31 32 /* F5 * E5L F5H F5L ZH 33 /* ZERO (an inaudible high-frequenc 34 ZLStep by Step Solution
There are 3 Steps involved in it
Step: 1
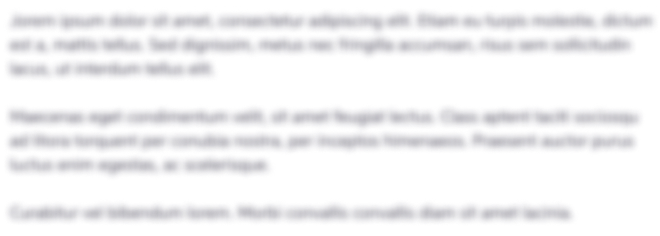
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started