Answered step by step
Verified Expert Solution
Question
1 Approved Answer
MUST BE A . h AND A . cpp FILE The purpose of this assignment is to give you some practice with creating your own
MUST BE A h AND A cpp FILE The purpose of this assignment is to give you some practice with creating your own classes. This program serves as the basis for all the other programming assignments in this class and your future Computer Science classes.
Reminders:
Do not put all your code into a single file. It will cost you points.
Each Class you create requires two files. The first one is h and the second one is cpp If each Class is not in this format, it will cost you points.
o Do a Google search on Ch vs cpp to get a good idea of what type of code goes in each file. Putting the wrong code in one of the files can cost you points.
Each class has one or more constructors. Many students make a mistake with their Class constructors you will be learning about them and use code that looks like this: std::string k : setNamek
o The code in bold and yellow belongs between the not in the declaration line of the constructor. There is a loophole in C that allows you to put code after the constructor declaration, but in CSIS always put the code between the or it will cost you points. Use the example below for the correct format. std::string k setNamek;
o You will find examples of code after the constructor declaration and a colon. The only purpose we put code after the constructor declaration in this class is when we get into Inheritance. We will not use Inheritance until the last few weeks of the course.
Unless instructed to do so there is no user input or output done inside a class.
Remember to review the checklist at the end of the instructions.
INSTRUCTIONS
Class:
Name: Circle
Purpose: To accept and store the data for a circle and to provide functions that will manipulate the data.
Constructor:
Zeroargument constructor that initializes the data members to
Data Members:
radius int Must be or greater.
colorFill string Cannot be blank.
Functions:
calcDiameter double Calculates and returns the diameter of the circle. The diameter is a double.
calcArea double Calculates and returns the area of the circle. The area is a double.
calcCircumference double Calculates and returns the circumference of the circle. The circumference is a double.
setRadius void Accepts a radius value and updates the radius data member.
getRadius int Returns the value stored in the radius data member.
setColorFill void Accepts the fill color value and updates the colorFill data member.
getColorFill string Returns the value stored in the colorFill data member.
Note: Setters and Getters are required even if they are not used.
NonClass Functions:
displayCircleInfo void Creates and displays the formatted output of the book shipment information. Do a Google search for C setw left, right for how to use these functionscommands to create easy to read formatted output.
Formulas:
Note: The calc functions are returning a double, and the radius is an integer. The simplest way to convert an int to a double is to multiply the radius by Example: double myDiameter; myDiameter getRadius ;
Area Pi radius squared For Pi use the value of so your results will match mine. You can make this a const using the following line of code in the Circle.cpp file above the constructor const double PI ;
For radius squared, use the pow function instead of getRadius getRadius Example: pow is squared where is the value of the radius, and is the power to raise the radius to in this case, squared. Use the getRadius function to get the radius value.
Circumference PI radius
Diameter radius
The class should use appropriate protection levels for the member data and functions. Data members must be in the private: section. It must also follow principles of minimalization: that is no data member should be part of a class unless most functions of the object need it A general rule of thumb is that if you can easily calculate it dont store it
Program Flow:
Start a loop that goes until the user enters for the radius.
Create the Circle object using the zero argument constructor.
Prompt the user for the radius. If the user enters exit the loop, otherwise, update the Circle object with the value entered.
Prompt the user for the fill color and update the Circle object with the value entered.
Display the Circle information using a separate function. This function will call the Circle object functions defined in the class.
Pause the display so the user can read the displayed information.
Your program should allow the user to enter new circle radius until the user enters for the radius. Be sure to include appropriate error checking. Does it make sense to enter abc as the radius of a circle? No Ther
Step by Step Solution
There are 3 Steps involved in it
Step: 1
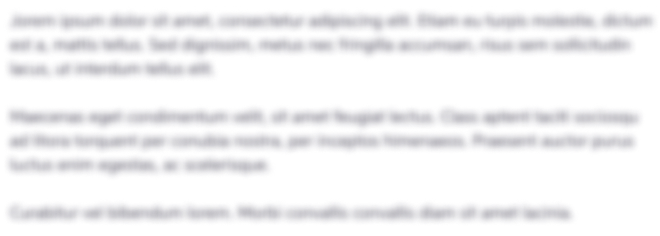
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started