Question
Must be C++ Objectives below 1. Write a function called factorialIterative(). This function should take a single BIG integer (bigint) as its parameter n, and
Must be C++ Objectives below
1. Write a function called factorialIterative(). This function should take a single BIG integer (bigint) as its parameter n, and it will compute the factorial n! of the value and return it as its result (as a bigint). Write this functions using a loop (do not use recursion).
2. Write the factorial function again, but using recursion. Call this function factorialRecursive(). The function takes the same parameters and returns the same result as described in 1.
3. Write a function called countCombinationsDirectly(). This function will compute the number of combinations that result from choosing i elements from set of n items. The function should take two bigint values as its parameters n and i, which will be integers >= 0. The function should use the equation 3 above to directly compute the number of combinations. You should use your factorialRecursive() function to compute the factorials in this function.
4. Write a function called countCombinationsRecursive(). This function will also count the number of combinations of choosing i elements from a set of n items. However, you need to implement this calculation as a recursive function, using the general and base cases described above for counting combinations in equation 4 (general case) and equation 5 (base cases). Your function will take the same two bigint parameters as input n and i with values >= 0, and will return a bigint result. 3 You will again be given 3 starting template les like before, an assg-04.cpp le of tests of your code, and a BinomialFunctions.hpp and BinomialFunctions.cpp header and implementation le. As before, you should practice incremental development, and uncomment the tests in the assg-04.cpp le one at a time, and implement the functions in the order shown. If you implement your code correctly and uncomment all of the tests, you should get the following correct output:
Main Assg.cpp
#include #include #include // measure elapsed time of functions using high resolution clock #include "BinomialFunctions.hpp"
using namespace std;
int main(int argc, char** argv) { // test iterative version of factorial cout
res = factorialIterative(1); cout
res = factorialIterative(-1); cout
res = factorialIterative(10); cout
res = factorialIterative(20); cout
// timing test for factorialIterative, do it 10000 times and see how much time // elapses // https://www.pluralsight.com/blog/software-development/how-to-measure-execution-time-intervals-in-c-- const int NUM_TIMING_LOOPS = 10000; auto start = chrono::high_resolution_clock::now(); for (int testnum = 0; testnum
// test recursive version of factorial cout
res = factorialRecursive(0); cout
res = factorialRecursive(1); cout
res = factorialRecursive(-1); cout
res = factorialRecursive(10); cout
res = factorialRecursive(12); cout
res = factorialRecursive(20); cout
cout
// timing test for factorialRecursive, do it 10000 times and see how much time // elapses // https://www.pluralsight.com/blog/software-development/how-to-measure-execution-time-intervals-in-c-- start = chrono::high_resolution_clock::now(); for (int testnum = 0; testnum
// test direct calculation version of counting combinations cout
int i; int n;
n=5; i=0; res = countCombinationsDirectly(n, i); cout
n=4; i=2; res = countCombinationsDirectly(n, i); cout
max factorial using bigint n=20; i=10; res = countCombinationsDirectly(n, i); cout
// test recursive calculation version of counting combinations cout
n=5; i=0; res = countCombinationsRecursive(n, i); cout
n=4; i=2; res = countCombinationsRecursive(n, i); cout
max factorial using bigint n=20; i=10; res = countCombinationsRecursive(n, i); cout
// timing test for countCobminationsRecursive, do it 10000 times and see how much time // elapses // https://www.pluralsight.com/blog/software-development/how-to-measure-execution-time-intervals-in-c-- start = chrono::high_resolution_clock::now(); for (int testnum = 0; testnum
return 0 to indicate successful completion return 0; }
Bimomial Functions.cpp below this
#include "BinomialFunctions.hpp"
using namespace std;
The hpp below this
#ifndef _BINOMIALFUNCTIONS_H_ #define _BINOMIALFUNCTIONS_H_
#include
using namespace std;
// global definitions typedef long long int bigint; // give long int an alias name of bigint
// Function prototypes for our BinomialFunctions library go here
#endif // _BINOMIALFUNCTIONS_H_
Code must follow this
1. All indentation must be correct according to class style guidelines (2 spaces, no tabs, all code blocks correctly indented by level).
2. All functions (and member functions of classes) must have a function header documentation that gives a short description, documents all input parameters using @param tags, and documents the return value if the function returns a value, using @returns tag. You do not have to document class setter/getter methods, but all other class methods should probably be documented
Step by Step Solution
There are 3 Steps involved in it
Step: 1
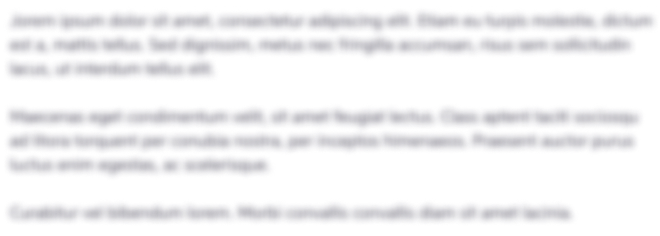
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started