Question
Must be done in Scala! package test.poker object Poker extends App { /* * Given a set of 5 playing card identifiers such as 2H,
Must be done in Scala!
package test.poker
object Poker extends App {
/* * Given a set of 5 playing card identifiers such as 2H, 7C, QS, 10D, 2D; * determine if this hand is better than some other hand, according to the rules of poker. * * Hands will be a string with 5 cards comma separated, * each card will have 1-2 digits or JQKA and a suit indicator C,D,S,H (i.e. 10C, KH) * * Possible Hand Types Below: * Straight flush * Four of a kind * Full house * Flush * Straight * Three of a kind * Two pair * One pair * * The goal of this is to compare between the hand types. * Comparing 2 of the same type (i.e. 2 straights) to determine a winner is outside the scope * and will not be tested. * * Implement hand1WinsOverHand2 method and return whether or not the first hand wins over the second hand. */ def hand1WinsOverHand2(hand1Str: String, hand2Str: String): Boolean = ???
val faceValues = Array("A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A") implicit class CompareTwoPokerHands(hand1: String) { def winsOver(hand2: String): Unit = { val result = if (hand1WinsOverHand2(hand1, hand2)) "Correct" else "Incorrect" println(s"$result, hand [$hand1] wins over [$hand2]") } def hand1WinsOverHand2(hand1: String, hand2: String): Boolean = { if (getResultOfHand(hand1) > getResultOfHand(hand2)) return true else return false } def getResultOfHand(hand: String): Int = { val suits = hand.split(",").map(_.toString().takeRight(1)) val values = hand.split(",").map(_.dropRight(1)) // Check for flush, then check for straight flush if (suits.distinct.length == 1) { if (checkStraight(values)) return 8 //Straight Flush else return 6 //Flush } if (checkFour(values)) return 7 //4 of a kind if (checkThree(values)) if(checkTwo(values) == 1) return 5 //Full House else return 3 //Three of a kind if (checkStraight(values)){ return 4 // Straight } return checkTwo(values) } def checkStraight(values: Array[String]): Boolean = { val stringCompare = faceValues.mkString("") val cardFaceValues = values.mkString("") if (stringCompare.contains(cardFaceValues)) return true else return false } def checkFour(values: Array[String]):Boolean = { for (a <- faceValues) { if (values.count(_ == a) == 4) return true; } return false; } def checkThree(values: Array[String]):Boolean = { for (a <- faceValues) { if (values.count(_ == a) == 3) return true } return false } def checkTwo(values: Array[String]):Integer = { var count = 0 for (a <- faceValues) { if (values.count(_ == a) == 2) count += 1 } return count } }
println("Poker Hand comparison") "8C,9C,10C,JC,QC" winsOver "6S,7H,8D,9H,10D" // straight flush "4H,4D,4C,4S,JS" winsOver "6C,6S,KH,AS,AD" // four of a kind "5C,3C,10C,KC,7C" winsOver "6C,6D,6H,9C,KD" // flush "4H,4D,4C,KC,KD" winsOver "9D,6S,KH,AS,AD" // full house "2C,3C,4S,5S,6S" winsOver "6C,6D,6H,9C,KD" // straight "7C,7D,7S,3H,4D" winsOver "9S,6S,10D,AS,AD" // three of a kind "8C,8H,10S,KH,KS" winsOver "2S,2D,JH,7S,AC" // two pair "AC,AH,3C,QH,10C" winsOver "3S,2D,KH,JS,AD" // one pair System.exit(0) }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
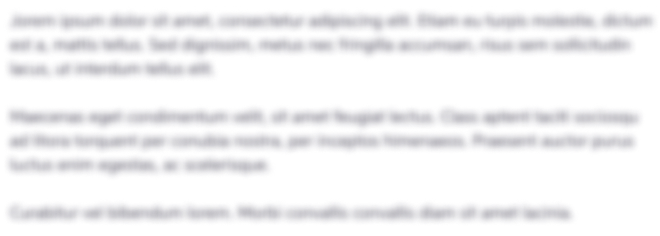
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started