Question
Must be in Python 3, thank you! Your best friend is planning a family, and would like to have a program that will help keep
Must be in Python 3, thank you!
Your best friend is planning a family, and would like to have a program that will help keep track of baby names. You program will allow the user to store baby names, modify the collection of names and report on the stored names.
Your program is to use a DICTIONARY to store baby names. A dictionary stores [key:item] pairs. Each key in this dictionary is a name (string). Associated with each key is a string (girl, boy, or both).
Eg.) this dictionary might at one point store: { Bob:boy, Alicia:girl, Sue:girl, Morgan:both }
You are to code the following functions, to be stored in a module named dictfunc.py. Your program will use these functions to complete its tasks.
is_there(thedict, key)
This function accepts two parameters, a dictionary and a key to be sought for. If the key is in this dictionary, the function returns a 1, otherwise a 0 is returned.
print_all_keys(thedict)
This function prints out all the keys in this dictionary.
For example, if the above dictionary is passed to this function, the function would print out:
Bob
Alicia
Sue
Morgan
print_some_keys(thedict, item)
This function prints out all the keys in the dictionary whose associated value is item.
For example, if the above dictionary is passed to this function, with the item girls, the function would print out:
Alicia
Sue
remove_name(the_dict, key)
This function accepts two parameters, a dictionary and the key of the element to be removed. If the key is not in the dictionary, and a message is printed. Otherwise the element with the matching key is deleted from the dictionary.
For example, ifthe above dictionary is passed to this function, with the key Alicia, the
dictionary would be updated to: { Bob:boy, Sue:girl, Morgan:both }
THE MAIN PROCESS:
The program is use a dictionary to store names. The program will begin with an empty dictionary. The user will be offered the following options until he/she wishes to exit:
* add a name to the collection. The user is to be prompted for the name. If the name is
already in the dictionary, report this to the user. If the name is not stored, ask the user if
name is intended for a boy, girl or both, and add an element to the dictionary.
Regardless of how names are entered, they should be stored as all upper case. That is, if a name is entered
Fred, fred, or fRed, it will be stored as FRED. Keep this in mind when searching for names
* find out if a name is stored. The user will input a name. The program will report if the
name is currently stored or not. The dictionaryr will not be updated by this option.
* display the number of names currently stored in the dictionary
* print out all boy only names currently stored in the dictionary
* print out all girl only names currently stored in the dictionary
* print out all both gender names currently stored in the dictionary
* print out all names and associated genders that are currently stored in the dictionary
* remove a name from the dictionary. The user will provide the name. If the name is not
currently stored, the user will be informed.
***Please make sure that all the functions have a comment indicating what they do, and that the main process contains commented so that it reads clearly.***
Step by Step Solution
There are 3 Steps involved in it
Step: 1
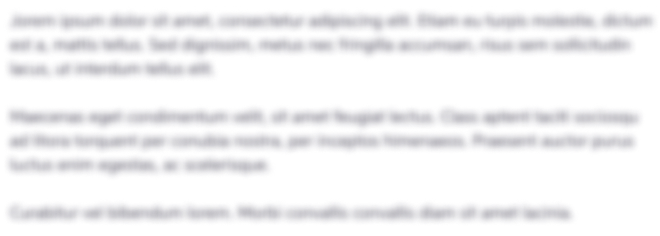
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started