Question
must use code blocks c++ This assignment demonstrates array of STRUCT (structures), two dimensional arrays and the use of index sorting routine. The problem: A
must use code blocks c++
This assignment demonstrates array of STRUCT (structures), two dimensional arrays and the use of index sorting routine.
The problem:
A trucking company charges the customers a fixed amount per pound, based on the following (Zone-Area) table.
Area code Area code Area code
Zone 1 2 3
code
1 .40 .55 .68
2 .60 .70 .85
3 .75 .79 .96
4 .90 1.05 1.10
5 .99 1.08 1.15
Write a program which will read in the above table into a two dimensional array. The array will be used to calculate the shipping cost. Your program will read in the name of the customer, the zone-area code, and the weight of the item shipped. The zone-area code consists of two digits, the first one indicates the zone, and the second indicates the area.
Assume there are less than 20 records for one truckload.
Print all customers information and their shipping charges in a report form. The end of the report must contain the total of all of the customers weight and a total of all the shipping charges.
Reproduce the above report in alphabetical order by customers name
Reproduce the above report sorted by weight.
Produce a report of all the companies in area code number two.
Your test data will be similar to the following:
3
Chevrolet 23 750
Zapata Co. 53 385
Schlumberger 51 1104
The first line of the data file will contain a header record indicating how many customer records are to follow.
The actual test data will be stored under DATA7.txt
The Zone-Area table will be stored under TABLE7.txt
Submit your source code in blackboard and demonstrate your program in lab.
Documentation will be 20% of your grade.
Your source code must contain the following documentation.
Header information: (Source Code and Output)
Your Name, course & section number, assignment number and due date.
A brief description of your assignment.
Variable dictionary: A brief description of every variable used in your program.
Comments related to input and output of data and all formulas used in your code.
data7.txt
10 Chevrolet.... 23 750 Zapata.Co.... 53 375 Schlumberger. 51 1204 FMC.......... 13 675 Buick........ 22 475 Action.Inc... 41 1712 IBM.......... 32 979 Apple.Comp... 42 790 Zapata.Co.... 52 443 Univ..Mich... 12 1960
table7.txt
1.45 1.65 1.75 1.60 1.75 1.85 1.75 1.85 1.90 2.10 2.15 2.35 2.19 3.19 4.19
shell
#include #include #include #include using namespace std; struct company { char name[14]; int zonearea; int weight; float cost; }; void readtable( ifstream &f2, float table[][4]); void readdata(ifstream &f1, company co_array[], int & header); int main() { company co_array[30]; float table [6] [4] = {0}; int header = 0; // initialize the array of structure for(int i = 0; i < 30; i++) { strcpy ( co_array[i].name, " "); co_array[i].zonearea = 0; co_array[i].weight = 0; co_array[i].cost = 0.0; } ifstream f1 ("h:\\data7.txt", ios::in); ifstream f2 ("h:\\table7.txt",ios::in); readtable(f2,table); readdata(f1, co_array, header); f1.close(); f2.close(); return 0; } // main void readtable( ifstream &f2, float table[][4]) { cout<<" table7.txt file "; for(int row =1; row < 6; row ++) { for (int col = 1; col < 4; col++) { f2>> table[row] [col]; cout<< table [row] [col]<< " "; } cout<< endl; }// end row }// end function readtable void readdata(ifstream &f1, company co_array[], int & header) { // read header record f1>>header; cout<<" header record for data7.txt = "<< header<<" "; for( int i = 0; i < header; i++) { f1>> co_array[i].name >> co_array[i].zonearea >> co_array[i].weight; cout<< co_array[i].name <<" " << co_array[i].zonearea<<" " <
Step by Step Solution
There are 3 Steps involved in it
Step: 1
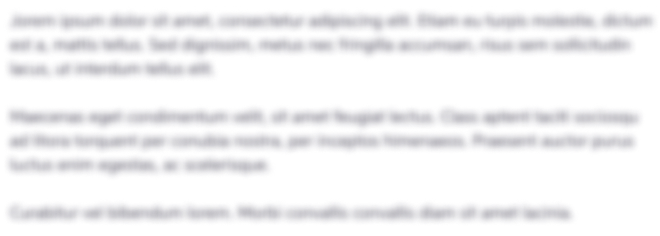
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started