Question
MUST USE GETLINE IF YOU`RE USING IF YOU NEED TO GET SOMETHING. First I have written a header file for you for a class called
MUST USE GETLINE IF YOU`RE USING IF YOU NEED TO GET SOMETHING.
First I have written a header file for you for a class called Friend. (Note that we can only call this class Friend if we use capitalization, since the word friend is reserved.) This class has have two private variables, one for the friends name and the other for their birthday, the later being of type Date, another class that I have given you. You are to write the implementation of this class, including overloaded insertion and extraction operators as well operators for = = and !=. Two friends are equal only if they have the same name and the same birthday. (Doctors offices do this because of the low probability that two people will have both the same name and the same birthday.)
Test this class by writing a main of your own that declares a two friends, lets you put the information into both, outputs them to the screen and compares them for equality.
Now, in the main that I have given you, you will find that the application allows:
The addition of a new friend to the beginning of the list
The viewing of all the friends in the list
The ability to walk through the list, viewing one friend at a time
The ability to remove a friend from the list (de-friending someone)
The ability to insert a new friend at some spot in the middle of the list, which includes at the back end
The ability to sort all the friends by their birthdays
The ability to find a friend using their name
There is also a file backup mechanism. In this case it operates using the persons username for the name of the file. {OVER}
I have given you the header file for the container class that makes all of this possible, fbfriends.h. You are to write the implementation of this as well. The private variables for this class consist of a pointer of type Friend and variables for capacity, used, and current_index. The constructor will begin by allocating a dynamic array of Friends capable of holding only five friends. (This will save on memory space for those users with few friends.) When the action of adding an additional friend to the list happens you should check if used == capacity, and if it does do a resize operation, that increases the size of the array by five.
This container also has an internal iterator, as the author illustrated in section 3.2 of the text, which will require that you write the functions
start
advance
is_item
current
remove_current
insert
attach
and you will find that these are implemented in the same way as they are in the text. You will also need to implement show_all used mostly for testing purposes, bday_sort, find_friend, and is_friend.
Because this is a dynamic array you will need to write a resize function, and the Big 3 (destructor, copy constructor, and assignment operator). And because were providing file backup we will also have functions for load and save.
date.h*****************************************
#include #include #ifndef DATE #define DATE // an exception if the date entered is invalid for the month class bad_day{ public: bad_day(){monthnum=daynum=0;} bad_day(int m, int d) {monthnum = m, daynum=d;} void msg(){std::cerr<
#include "date.h" using namespace std; // default constructor Date::Date(){ day=month=year=1; // permissable days for each month are loaded into an array daysallowed[0] = 0; daysallowed[1] = 31; daysallowed[2] = 29; daysallowed[3] = 31; daysallowed[4] = 30; daysallowed[5] = 31; daysallowed[6] = 30; daysallowed[7] = 31; daysallowed[8] = 31; daysallowed[9] = 30; daysallowed[10] = 31; daysallowed[11] = 30; daysallowed[12] = 31;} // constructor that takes three arguments Date::Date(int d,int m, int y):day(d),month(m),year(y) { // permissable days for each month are loaded into an array daysallowed[0] = 0; daysallowed[1] = 31; daysallowed[2] = 29; daysallowed[3] = 31; daysallowed[4] = 30; daysallowed[5] = 31; daysallowed[6] = 30; daysallowed[7] = 31; daysallowed[8] = 31; daysallowed[9] = 30; daysallowed[10] = 31; daysallowed[11] = 30; daysallowed[12] = 31;} // output operator, overloaded as a friend function ostream& operator <<(ostream& outs, Date d){ outs<
friend.h**************************************************
#include #include #include "date.h" #ifndef FRIEND_H #define FRIEND_H class Friend{ public: Friend(); std::string get_name()const; Date get_bday()const; bool operator == (const Friend& other)const; bool operator != (const Friend& other)const; void input(std::istream& ins); void output(std::ostream& outs)const; private: std::string name; Date bday; }; std::istream& operator >>(std::istream& ins,Friend& f); std::ostream& operator <<(std::ostream& outs,const Friend& f); #endif
fbfriends.h***********************************************
#include #include #include #include "friend.h" #ifndef FBFRIENDS_H #define FBFRIENDS_H class FBFriends{ public: FBFriends(); //The functions known as the Big 3 ~FBFriends(); FBFriends(const FBFriends& other); void operator = (const FBFriends& other); // Functions for the internal iterator void start(); void advance(); bool is_item(); Friend current(); void remove_current(); void insert(const Friend& f); void attach(const Friend& f); void show_all(std::ostream& outs)const; void bday_sort(); Friend find_friend(const std::string& name)const; bool is_friend(const Friend& f) const; void load(std::istream& ins); void save(std::ostream& outs); private: void resize(); // increases the capacity of the container by 5 Friend *data; int used; int capacity; int current_index; }; #endif
main.cc***********************************************************
#include #include #include #include #include "friend.h" #include "fbfriends.h" using namespace std; int menu(); int menu2(); int main(){ Friend myfriend; FBFriends myfb; string friendname; int choice,choice2; string username, filename; ifstream fin; ofstream fout; bool cutout; cout<<"Welcome to Friends Management. "; cout<<"Begin by entering your username: "; getline(cin,username); filename = username + ".txt"; fin.open(filename.c_str()); if(!fin.fail()) myfb.load(fin); fin.close(); choice = 0; choice2 = 0; cutout = false; FBFriends original(myfb); while(choice != 9){ choice = menu(); switch(choice){ case 1: cin>>myfriend; myfb.start(); myfb.insert(myfriend); break; case 2: myfb.show_all(cout); break; case 3: {myfb.start(); choice2 = 0; while(myfb.is_item()&& choice2 <= 5){ cout<>myfriend; if(myfb.is_friend(myfriend)) cout<<"Already in list. "; else myfb.insert(myfriend); cutout = false; } else if(choice2 == 3){ if(!cutout) cin >> myfriend; if(myfb.is_friend(myfriend)) cout<<"Already in list. "; else myfb.attach(myfriend); cutout = false; } else if (choice2 == 4){ myfriend = myfb.current(); myfb.remove_current(); cutout = true; } else if(choice2 == 5){ myfb.advance(); } else cout<<"Going back to main menu. "; } break; } case 4: myfb.bday_sort(); break; case 5:{ cout<<"Enter the name of your friend: "; if(cin.peek() == ' ') cin.ignore(); getline(cin, friendname); myfriend = myfb.find_friend(friendname); cout<>ans; return ans; } int menu2(){ int ans; cout<<"What would like to do with this friend? "; cout<<"1 - Remove from the list. "; cout<<"2 - Insert a new friend or cut-out friend before this friend. "; cout<<"3 - Put a new friend or cut-out friend after this friend. "; cout<<"4 - Cut this friend from the list to be inserted elsewhere. "; cout<<" If you want the friend earlier in the list you will need to start a new walk-through. "; cout<<"5 - Advance to the next friend. "; cout<<"6 - Return to main menu. "; cin>>ans; return ans; }
rplan.txt*************************************
Billy Gibbons 12/16/1949 Jimmy Page 1/9/1944 Jeff Beck 6/24/1944 George Harrison 2/25/1943 Eric Clapton 3/30/1945 Lindsey Buckingham 10/3/1949
Step by Step Solution
There are 3 Steps involved in it
Step: 1
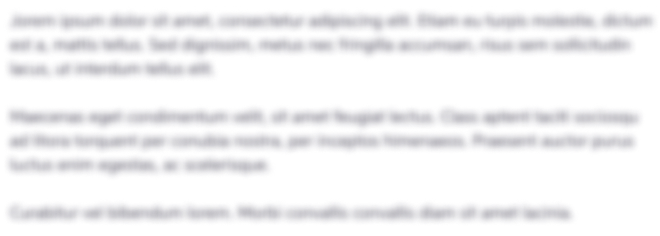
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started