Question
My assignemnt is this 1. Define the class Date which has the data attributes (fields) month, day, and year, and the methods constructor Date( String
My assignemnt is this
1. Define the class Date which has the data attributes (fields) month, day, and year, and the methods constructor Date( String m, int d, int y), toString() and validate(). The toString converts an object of type Date into a string of the following format July, 16, 2018. The validate() is called by the constructor to validate the date before initializing the month, day, and year( you may use the enum type for the months See chapter 8. The year should be five digits <2050 and the day must be between 1 31 or 30 28 29 depending on month ). If the date is invalid, the constructor prints an error message and asks you to renter the date.
2. Define the class Employee which the data attributes: name, ssn, position, dateOfBirth, and pay. The first three attributes of type string, dataofBirth of type Date, and pay of type float. The class Employee has the following methods:
The method toString(): returns the employee info as a string of the following format:
Name:
SSN:
Position:
Date of Birth:
Pay:
The method raise ( float per) which increases the pay by the given percentage, per.
The methods set and get for each attributes including the dateOfBirth.
3. Define the class PTEmployee as an extended class of Employee and include two additional attributes: nHours and wages. The constructor of the extended class is defined by calling the constructor of the superclass Employee (assuming the pay =0)and then initializing the nHours , wages , and pay. The pay is computed as nHours*wages. The method raise( float amount) for this subclass increases the wages by the given amount( it is not percentage) and re compute the pay . Also, this class has get and set methods for nHours and wages. The method toString for the subclass PTEmployee uses the toString of the super class Employee and adds to it nHours and wages.
4. Write the program EmployeeDemo which inputs employee info from the keyboard and creates two objects E of type Employee and the second one PE of type PTEmployee. Test your program for the following:
Entering invalid date;
Call every method above for each of E and PE and print the return value( if it returns a value);
Declare two variables EV of type Employee and PEV of type PTEmployee and the following four assignments:
EV=E
Call every one of the following methods: EV.setPay, EV.getPay, EV.setWages EV.getWages,
EV.raise( 4.0), getDateOfBirth() and then print the info of EV.
EV=PE
Call every one of the following methods: EV.setPay, EV.getPay, EV.setWages EV.getWages,
EV.raise( 4.0), getDateOfBirth() and then print the info of EV.
PEV=E
Call every one of the following methods: EV.setPay, EV.getPay, EV.setWages EV.getWages, EV.raise( 4.0), getDateOfBirth() and then print the info of PEV.
PEV=E
Call every one of the following methods: EV.setPay, EV.getPay, EV.setWages EV.getWages, EV.raise( 4.0), getDateOfBirth() and then print the info of PEV.
You may get error messages when you run some of the above methods. Explain the reason and comment out those methods, one at time, and rerun the program.
THIS IS WHAT I HAVE SO FAR BUT I GET THESE ERROR CODES PLEASE HELP FIX
EmployeeDemo.java:80: error: incompatible types: Employee cannot be converted to PTEmployee PEV = E; ^ EmployeeDemo.java:83: error: cannot find symbol PEV.setWage(84); ^ symbol: method setWage(int) location: variable PEV of type PTEmployee 2 errors
import java.util.Scanner; public class EmployeeDemo { public static void main(String[] args) { Scanner kb = new Scanner(System.in); int day, year, payAsInt, hours, day2, year2, payAsInt2, wagesAsInt; String month, name, ssn,pos, ssn2, pos2, month2; float pay, pay2, wages; System.out.println("Please enter the name"); name = kb.nextLine(); System.out.println("Please enter the ssn"); ssn = kb.nextLine(); System.out.println("Please enter the position"); pos = kb.nextLine(); System.out.println("Please enter the DOB beginning with the month"); month = kb.nextLine(); System.out.println("Next, please enter the day"); day = kb.nextInt(); System.out.println("Lastly, please enter the year"); year = kb.nextInt(); System.out.println("Please enter the pay"); payAsInt = kb.nextInt(); pay = payAsInt; Date dob = new Date(month,day,year); System.out.println(dob.toString()); System.out.println("The next following instructions will be for the next employee"); System.out.println("Please enter the name"); String name2 = kb.nextLine(); System.out.println("Please enter the ssn"); ssn2 = kb.nextLine(); System.out.println("Please enter the position"); pos2 = kb.nextLine(); System.out.println("Please enter the DOB beginning with the month"); month2 = kb.nextLine(); System.out.println("Next, please enter the day"); day2 = kb.nextInt(); System.out.println("Lastly, please enter the year"); year2 = kb.nextInt(); System.out.println("Please enter the pay"); payAsInt2 = kb.nextInt(); pay2 = payAsInt2; System.out.println("Please enter the hours"); hours = kb.nextInt(); System.out.println("Please enter the wage"); wagesAsInt = kb.nextInt(); wages = wagesAsInt; Date dob1 = new Date(month2,day2,year2); System.out.println(dob1.toString()); Employee E = new Employee(name,ssn,pos,dob,pay); PTEmployee PE = new PTEmployee(name2,ssn2,pos2,dob1,pay2,hours,wages); System.out.println(" Employee Details:"); System.out.println(E.toString()); System.out.println(" PTEmployee Details:"); System.out.println(PE.toString()); Employee EV = new Employee(); PTEmployee PEV = new PTEmployee(); //p1 EV=E; EV.setPay(1200); System.out.println(EV.getPay()); EV.raise(4.0f); System.out.println(EV.getDateOfBirth()); System.out.println(EV.toString()); // raise method needs to be a float //ev cant use setwage or getwage since it is not extended to PTE //p2 EV=PE; EV.setPay(900); //ev cant use wage since it is not extended System.out.println(EV.getPay()); EV.raise(4.0f); System.out.println(EV.getDateOfBirth()); System.out.println(EV.toString()); EV.setPay(123); EV.getPay(); EV.raise(4.0F); System.out.println(EV.getDateOfBirth()); //p3 PEV = E PEV = E; PEV.setPay(123); System.out.println("PEV.getPay()"); PEV.setWage(84); System.out.println("Pev.getWage()"); PEV.raise(4.0F); System.out.println(EV.getDateOfBirth()); } }
public class Employee { String name, ssn, position; Date dateOfBirth; float pay;
public Employee(){} public Employee(String name, String ssn, String position, Date dateOfBirth, float pay) { super(); this.name = name; this.ssn = ssn; this.position = position; this.dateOfBirth = dateOfBirth; this.pay = pay; } public String toString() { return "Name:"+name+" SSN:"+ssn+" Position:"+position+" " +"Date of Birth:" +dateOfBirth+" Pay:"+pay; } public void raise(float per) { pay = pay + ((per/100)*pay); } public void setName(String name) { this.name = name; } public String getName() { return name; } public void setSsn(String ssn) { this.ssn = ssn; } public String getSsn() { return ssn; } public void setPosition(String position) { this.position = position; } public String getPosition() { return position; } public void setDateOfBirth(Date dateOfBirth) { this.dateOfBirth = dateOfBirth; } public Date getDateOfBirth() { return dateOfBirth; } public void setPay(float pay) { this.pay = pay; } public float getPay() { return pay; } }
public class PTEmployee extends Employee { private int nHours; private float wages; public PTEmployee(String name,String ssn,String position,Date dateOfBirth,float pay,int nHours, float wages) { super(name,ssn,position,dateOfBirth,pay); this.nHours = nHours; this.wages = wages; } public PTEmployee(){ //constructor stub } public void raise(float amount) { wages=wages+amount; //calling computePay() method after wage raised by given amount this.computePay(); } public void computePay() { pay = nHours*wages; } public int getnHours() { return nHours; } public void setnHours(int nHours) { this.nHours = nHours; } public void setWages(float wages) { this.wages = wages; } public String toString() { return super.toString()+" nHours:"+nHours+" wages:"+wages+" "; //used toString emth from super clas } }
import java.util.LinkedHashMap; import java.util.Map; public class Date { private int day, year; private String month; Map month_Days=new LinkedHashMap();
Date(String m,int d, int y) { if(validate(m,d,y)) { month = m; day = d; y = year; } else System.out.println("Date inputed is wrong"); } private boolean validate(String m, int d, int y) { boolean monthValid = false; boolean dayValid=false; boolean yearValid=false; m = m.toUpperCase(); //adding the d/m here month_Days.put("JANUARY",31); //for leap year, 29days if(y%4==0) month_Days.put("FEBRUARY",29); else month_Days.put("FEBRUARY",28); month_Days.put("MARCH",31); month_Days.put("APRIL",30); month_Days.put("MAY",31); month_Days.put("JUNE",30); month_Days.put("JULY",31); month_Days.put("AUGUST",31); month_Days.put("SEPTEMBER",30); month_Days.put("OCTOBER",31); month_Days.put("NOVEMBER",30); month_Days.put("DECEMBER",31); //if map contains month,then month is valid, else invalid if(month_Days.containsKey(m)) monthValid=true; else monthValid=false; if(y<2050) { yearValid = true; if(y%4 == 0) { if(month_Days.get("FEBRUARY")>1 && month_Days.get("FEBRUARY")<=29) { dayValid=true; } else dayValid = false; } else //4 non leap { //num of days in feb 1-28 if(month_Days.get("FEBRUARY")>1 && month_Days.get("FEBRUARY")<=28) { dayValid = true; } else { dayValid = false; } } } else yearValid = false; if(monthValid & dayValid & yearValid) return true; else return false; } public String toString() { return month+","+day+","+year; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
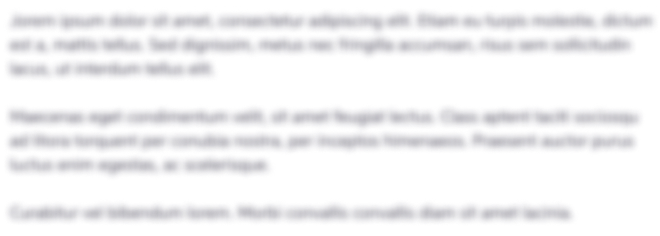
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started