Question
My codes import java.util.Random; import java.util.Scanner; public class TwentyFortyEight { //This instance variable represents the board. Use this to make changes. private int[][] board; //This
My codes
import java.util.Random; import java.util.Scanner; public class TwentyFortyEight { //This instance variable represents the board. Use this to make changes. private int[][] board; //This variable keeps track of the current score. private int score; private int boardWidth; //Constructor public TwentyFortyEight(int boardWidth){ this.boardWidth = boardWidth; this.score = score; board = new int[boardWidth][boardWidth]; placeRandom(); // TODO } //This function resets the board to its initial state public void reset() {for(int i = 0;iboardWidth || fromCol>boardWidth||toCol>boardWidth||toRow>boardWidth){ return false; }else if(board[fromRow][fromCol]==0){ return false; }else if(board[toRow][toCol]==0){ board[toRow][toCol]+=board[fromRow][fromCol]; board[fromRow][fromCol]=0; return true; }else if(board[toRow][toCol]==board[fromRow][fromCol]){ board[fromRow][fromCol]=0; board[toRow][toCol]=board[toRow][toCol] * 2; return true; }else return false; // TODO } //The following four functions move the board in a single direction. public boolean moveUp(){ boolean b; boolean c=true; for(int i = 0; i 0;i--){ for(int j = boardWidth;j>0 ;j--){ b = moveTo(i,j,i-1,j); c = b||moveTo(i,j,i-1,j);} }return c; // TODO } public boolean moveRight() { boolean b; boolean c=true; for(int i = 0; i 0;i--){ for(int j = boardWidth;j>0;j--){ b = moveTo(i,j,i,j-1); c = b||moveTo(i,j,i,j-1);} }return c; // TODO }//
how to fix moveUp etc. partsWhen I use it the number doesn't move at all.
Part 4 - Moving Pieces
Problem
Shifting pieces around and combining them is what makes the game so fun! The moveTo method receives four parameters, which are two sets of coordinates. The first pair are coordinates of the cell from which we are trying to move, while the second pair are the coordinates of the cell to which we are trying to move. Since moveTo is a helper function for later overall board functions, moveTo will only move or merge a piece at most one space in any direction (i.e. the from piece and the to piece should be adjacent).
2048 has the following rules when it comes to shifting adjacent pieces:
If the to piece or the from piece is out of bounds, you cannot shift, so do nothing.
If the to piece and the from piece are not adjacent, do nothing.
If the from piece is a 0, do nothing (nothing to add/move)
If the from piece and the to piece have the same value, shift the from piece into the to space, by doubling the to space's value and reducing from to zero.
If the to piece is zero, simply move the from piece into the to space.
Preparation
Think about what values are valid for each of the four parameters to have.
Procedure
Implement the moveTo method. This method returns true if a piece was moved, otherwise it returns false.
Check the bounds of all the variables (both for adjacency and for the bounds of the board itself)
If the cell we are moving from is 0, return false
If the to cell is 0, shift the value in the from cell to the to cell and erase the from cell.
If the values in from and to are the same, add them, place the added value in to, and clear the from value.
If the cell values are not equal and greater than zero, return false.
Part 5 - Move (up|right|down|left)
Problem
If you play 2048, you can see that single pieces do not just move to the right when you send the move right command, but rather the entire board. Now that we can move pieces, the next step is to write functions that move an entire board in one direction (merging when necessary). The moveUp, moveDown, moveLeft, and moveRight functions will do this operation on the entire board, using the moveTo helper function to make moving cells more manageable.
Preparation
Think about how the board is represented in your program.
Think about how moveTo() can help you accomplish this task.
Procedure
Implement moveUp, moveDown, moveLeft, and moveRight. The procedure to move an entire board in direction X is as follows:
For each cell C in a row or column (depending on move direction), beginning with the cell furthest in the direction X, move or merge that cell one space in direction X unless either:
C cannot be moved due to either its value being zero or it being adjacent to a cell with a different value.
C cannot be moved because it has reached the board boundary.
After all moves are completed, determine if a cell was created with a higher value than the current score. If so, update the score variable with the highest value on the board.
If at least one move was made, return true. Otherwise, return false.
Example
Note: moveX() only moves all pieces on the board at most 1 space in that direction. A single call to moveRight(), for example, will cause the following change to occur in the below example (note the order in which the merge happens, starting with the cell furthest to the right in the row, since this is a moveRight() call):
------------------------- | 4| 4| 2| 2| ------------------------- | | | | 4| ------------------------- | | | | | ------------------------- | | | | | ------------------------- moveRight() -> ------------------------- | | 4| 4| 4| ------------------------- | | | | 4| ------------------------- | | | | | ------------------------- | | | | | -------------------------
urrent score: 4 | Best score: 4 ------------------------- | 4| 4| 2| 2| ------------------------- | | | | 4| ------------------------- | | | | | ------------------------- | | | | | ------------------------- Enter up, down, left or right to move in that direction. Enter reset to reset the game or quit to exit. right Current score: 8 | Best score: 8 ------------------------- | | | 4| 8| ------------------------- | | | | 4| ------------------------- | | 2| | | ------------------------- | | | | | ------------------------- Enter up, down, left or right to move in that direction. Enter reset to reset the game or quit to exit.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
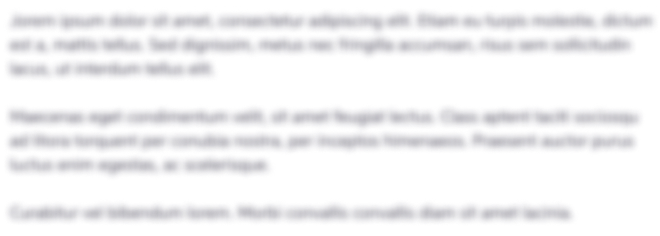
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started