Question
My Contact Manager v3 In the 1D Arrays Unit, you created a command-line Contact Manager v1. It is time to upgrade the software to include
My Contact Manager v3
In the 1D Arrays Unit, you created a command-line Contact Manager v1. It is time to upgrade the software to include a graphic user interface.
Your contact manager must:
Have a menu bar that provides access to the following functionality (it is up to you to organize): Quit the application Save the Contacts Load the Contacts Add a Contact Delete a Contact Edit a Contact View all entries in a table Sort the Contacts by a selected field Store the contacts in a 1D Contact array with at least the following fields: First Name Last Name Address Phone Number E-Mail Use methods to incorporate modular design. Use proper internal documentation as per the Java Coding Conventions. Include the executable JAR of your program Exceed the Expectations:
Add some style to the GUI using BorderLayout, Labels, and Font!
In Java Programming code.
Create it From My contact Manager v1:
///////////////////////////// JAVA program language , using NetBeans
/// Contact.java
public class Contact { private String name;// store name private String phNumber;// store phone number private String address;// store address private String emailId;// store email address // constructor public Contact(){ this.name=""; this.phNumber=""; this.address=""; this.emailId=""; } // setter function public void setName(String a){ this.name=a; } public void setPhoneNumber(String a){ this.phNumber=a; } public void setAddress(String a){ this.address=a; } public void setEmailID(String a){ this.emailId=a; } // getter function public String getName(){ return(this.name); } public String getPhoneNumber(){ return(this.phNumber); } public String getAddress(){ return(this.address); } public String getEmailID(){ return(this.emailId); } // to string method for display public String toString(){ String ret=""; ret+="Name : "+this.name; ret+=" Phone Number : "+this.phNumber; ret+=" Address : "+this.address; ret+=" Email ID : "+this.emailId; return(ret); } }
///// ContactProgram.java
import java.io.BufferedReader; import java.io.File; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.io.PrintWriter; import java.util.Scanner;
public class ContactProgram {
// seraching by name public static int searchByName(String s,Contact [] lst){ int ret=-1; for(int i=0;i // if name found if(s.equals(lst[i].getName())){ ret=i; break; } } return(ret); } // seraching by phone number public static int searchByPhone(String s,Contact [] lst){ int ret=-1; for(int i=0;i // if name found if(s.equals(lst[i].getPhoneNumber())){ ret=i; break; } } return(ret); } public static void main(String[] args) throws FileNotFoundException, IOException { // contact array of 100 contact object Contact [] cList= new Contact[100]; // intialise objects for(int i=0;i<100;i++){ cList[i]=new Contact(); } // index for entry store int index=-1; Scanner sc=new Scanner(System.in); while(true){
// menu System.out.println("1) Add Entry"); System.out.println("2) Modify Entry"); System.out.println("3) Delete Entry"); System.out.println("4) Load book"); System.out.println("5) Save book"); System.out.println("6) View all entry"); System.out.println("7) Exit"); System.out.print("Choose between 1 and 7 : ");
int input=sc.nextInt();
// for correct input if(input>0 && input<8){
// for entry if(input==1){ System.out.print("Enter Name : "); sc.nextLine();// ignore enter String name=sc.nextLine(); System.out.print("Enter Phone Number : "); String phnumber=sc.next(); System.out.print("Enter Address : "); sc.nextLine(); String address=sc.nextLine(); System.out.print("Enter Email ID : "); String email=sc.next();
index++;
// add contact in list cList[index].setName(name); cList[index].setAddress(address); cList[index].setPhoneNumber(phnumber); cList[index].setEmailID(email); }
// modify entry if(input==2){ // menu for modifying System.out.println("1) Modify by Name"); System.out.println("2) Modify by Phone Number"); System.out.print("Choose between 1 or 2 : "); input=sc.nextInt();
if(input>0 && input<3){ // modify ny name if(input==1){ System.out.print("Enter Name : "); sc.nextLine();// ignore enter String n=sc.nextLine();
//search name , if not found retun -1 int i_r=searchByName(n,cList); if(i_r!=-1){ // if write - , previous data does not change System.out.print("Enter Name [for no update , type - ] : "); String n_=sc.nextLine(); System.out.print("Enter Phone Number [for no update , type - ] : "); String p_=sc.next(); System.out.print("Enter Address [for no update , type - ] : "); sc.nextLine();// ignore enter String a_=sc.nextLine(); System.out.print("Enter Email ID [for no update , type - ] : "); String e_=sc.next();
// update data if(n_.equals("-")==false){cList[i_r].setName(n_);} if(p_.equals("-")==false){cList[i_r].setPhoneNumber(p_);} if(a_.equals("-")==false){cList[i_r].setAddress(a_);} if(e_.equals("-")==false){cList[i_r].setEmailID(e_);}
System.out.println("Data Modified."); } else{ System.out.println("No data Found in List."); }
} // modify by phone number if(input==2){ System.out.print("Enter Phone Number : "); sc.nextLine();// ignore enter String ph=sc.next();
// serach by phone number , if not found return -1 int i_r=searchByPhone(ph,cList); if(i_r!=-1){ System.out.print("Enter Name [for no update , type - ] : "); String n_=sc.nextLine(); System.out.print("Enter Phone Number [for no update , type - ] : "); String p_=sc.next(); System.out.print("Enter Address [for no update , type - ] : "); sc.nextLine();// ignore enter String a_=sc.nextLine(); System.out.print("Enter Email ID [for no update , type - ] : "); String e_=sc.next();
// update data if(n_.equals("-")==false){cList[i_r].setName(n_);} if(p_.equals("-")==false){cList[i_r].setPhoneNumber(p_);} if(a_.equals("-")==false){cList[i_r].setAddress(a_);} if(e_.equals("-")==false){cList[i_r].setEmailID(e_);}
System.out.println("Data Modified."); } else{ System.out.println("No data Found in List."); }
} } else{ System.out.println("Sorry , you typed wrong choice"); }
}
// delete entry if(input==3){ System.out.println("1) Delete by Name"); System.out.println("2) Delete by Phone Number"); System.out.print("Choose between 1 or 2 : "); input=sc.nextInt();
if(input>0 && input<3){ if(input==1){ System.out.print("Enter Name : "); sc.nextLine();// ignore enter String n=sc.nextLine();
// serach by name , if not found return -1 int i_r=searchByName(n,cList); if(i_r!=-1){ // delete contact // delete entry for(int c=i_r;c cList[c].setName(cList[c+1].getName()); cList[c].setPhoneNumber(cList[c+1].getPhoneNumber()); cList[c].setAddress(cList[c+1].getAddress()); cList[c].setEmailID(cList[c+1].getEmailID()); }
index--;
System.out.println("Data Deleted."); } else{ System.out.println("No data Found in List."); }
} if(input==2){ System.out.print("Enter Phone Number : "); String ph=sc.next();
// serach by phone number , if not found return -1 int i_r=searchByPhone(ph,cList); if(i_r!=-1){
// delete entry for(int c=i_r;c cList[c].setName(cList[c+1].getName()); cList[c].setPhoneNumber(cList[c+1].getPhoneNumber()); cList[c].setAddress(cList[c+1].getAddress()); cList[c].setEmailID(cList[c+1].getEmailID()); }
index--;
System.out.println("Data Deleted."); } else{ System.out.println("No data Found in List."); }
} } else{ System.out.println("Sorry , you typed wrong choice"); }
}
// load from file to array if(input==4){ System.out.print(" Enter the file name : "); String fName=sc.next(); BufferedReader br=new BufferedReader(new FileReader(fName)); String line=""; index=-1;
while((line=br.readLine())!=null){ String [] spt=line.split("#"); index++;
cList[index].setName(spt[0]); cList[index].setPhoneNumber(spt[1]); cList[index].setAddress(spt[2]); cList[index].setEmailID(spt[3]); }
br.close(); System.out.println("Data from file loaded in Array."); }
// load array data to file if(input==5){ System.out.print(" Enter the file name : "); String fName=sc.next();
PrintWriter writer =new PrintWriter(new File(fName));
for(int i=0;i<=index;i++){ writer.print(cList[i].getName()); writer.print("#"); writer.print(cList[i].getPhoneNumber()); writer.print("#"); writer.print(cList[i].getAddress()); writer.print("#"); writer.print(cList[i].getEmailID());
if(i writer.println(); } }
writer.close();
System.out.println("Data from ARRAY loaded to FILE."); } // display all contact if(input==6){ for(int i=0;i<=index;i++){ System.out.println(" Entry "+(i+1)+" ----"); System.out.println(cList[i]); } }
// exit loop if(input==7){ break; } } else{ System.out.println("Please enter choice between 1-7 ");; } } System.out.println("---- Program End ----"); } }
/////////// output and file
Step by Step Solution
There are 3 Steps involved in it
Step: 1
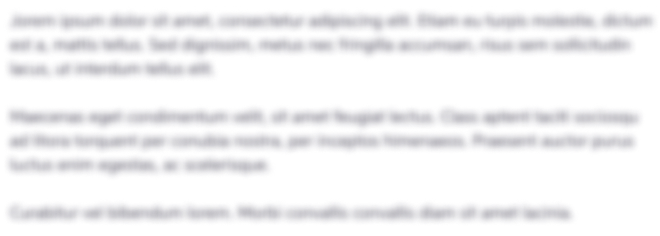
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started