Question
My homework assignment is to make a console based rock paper scissors game. Here are the instructions: You have to be able to play multiple
My homework assignment is to make a console based rock paper scissors game. Here are the instructions:
- You have to be able to play multiple rounds against the computer; the first side (player or computer) to win two more rounds than their opponent is considered the victor! (Think tennis)
-
E.g. If the computer has won 5 rounds, the user needs to win 7 times to win the game.
-
- After a winner is decided, user is asked if they want to play again, if not the program ends
We also get extra credit if we add Lizard and Spock as options in the game. My question is how do I get it to display the results of the game? I need the game to end after either the user or the computer has won by 2. I used an if statement to say if the user has won or lost, but that part is not printing. I have bolded the part of the code that I am having trouble with. Thanks!
import java.util.Scanner;
/**
* @author
*
*/
public class RockPaperScissors {
public static void main(final String[] args) {
final Scanner input = new Scanner(System.in);
//define variables
double playerWins = 0;
double computerWins = 0;
int playAgain;
System.out.println("Let's play rock, paper, scissors, with a twist!");
//start the loop to end until one has two more games won than the other
while (true) {
//prompt the used to enter their hand
System.out.println("Enter rock, paper, scissors, Lizard, or Spock.");
//store the user's input in the string
final String player = input.nextLine().toLowerCase();
//generate random number for the computer to play
double randomNumber = (int) (Math.random() * 4 + 1);
//if-else statements for user input = rock
if (player.equals("rock")) {
if (randomNumber == 0) {
System.out.println("Rock versus rock. It's a tie!");
} else if (randomNumber == 1) {
System.out.println("Rock versus paper. Paper covers rock. You lose!");
computerWins++;
} else if (randomNumber == 2) {
System.out.println("Rock versus scissors. Rock smashes scissors. You win!");
playerWins++;
} else if (randomNumber == 3) {
System.out.println("Rock versus Lizard. Rock smashes Lizard. You win!");
playerWins++;
} else
System.out.println("Rock versus Spock. Spock vaporizes rock. You lose!");
computerWins++;
}
//if-else statements for user input = paper
if (player.equals("paper")) {
if (randomNumber == 0) {
System.out.println("Paper versus rock. Paper covers rock. You win!");
playerWins++;
} else if (randomNumber == 1) {
System.out.println("Paper versus paper. It's a tie!");
} else if (randomNumber == 2) {
System.out.println("Paper versus scissors. Scissors cuts paper. You lose!");
computerWins++;
} else if (randomNumber == 3) {
System.out.println("Paper versus Lizard. Lizard eats paper. You lose!");
computerWins++;
} else
System.out.println("Paper versus Spock. Paper disproves Spock. You win!");
playerWins++;
}
//if-else statements for user input = scissors
if (player.equals("scissors")) {
if (randomNumber == 0) {
System.out.println("Scissors versus rock. Rock smashes scissors. You lose!");
computerWins++;
} else if (randomNumber == 1) {
System.out.println("Scissors versus paper. Scissors cuts paper! You win!");
playerWins++;
} else if (randomNumber == 2) {
System.out.println("Scissors versus scissors. It's a tie!");
} else if (randomNumber == 3) {
System.out.println("Scissors versus Lizard. Scissors decapitates Lizard. You win!");
playerWins++;
} else
System.out.println("Scissors versus Spock. Spock smashes scissors. You lose!");
computerWins++;
}
//if-else statements for user input = Lizard
if (player.equals("lizard")) {
if (randomNumber == 0) {
System.out.println("Lizard versus rock. Rock crushes Lizard. You lose!");
computerWins++;
} else if (randomNumber == 1) {
System.out.println("Lizard versus paper. Lizard eats paper. You win!");
playerWins++;
} else if (randomNumber == 2) {
System.out.println("Lizard versus scissors. Scissors decapitates Lizard. You lose!");
computerWins++;
} else if (randomNumber == 3) {
System.out.println("Lizard versus Lizard. It's a tie!");
} else
System.out.println("Lizard versus Spock. Lizard poisons Spock. You win!");
playerWins++;
}
//if-else statements for user input = Spock
if (player.equals("spock")) {
if (randomNumber == 0) {
System.out.println("Spock versus rock. Spock vaporizes rock. You win!");
playerWins++;
} else if (randomNumber == 1) {
System.out.println("Spock versus paper. Paper disproves Spock. You lose!");
computerWins++;
} else if (randomNumber == 2) {
System.out.println("Spock versus scissors. Spock smashes scissors. You win!");
playerWins++;
} else if (randomNumber == 3) {
System.out.println("Spock versus Lizard. Lizard poisons Spock. You lose!");
computerWins++;
} else
System.out.println("Spock versus Spock. It's a tie!");
}
This is the part I am having trouble with. This bolded part will not execute for some reason.
//Display the results of the game using another if-else statement
if (playerWins + 2 == computerWins) {
System.out.println("You have lost! Nice try!");
}
if (computerWins + 2 == playerWins) {
System.out.println("You have won! Congrats!");
}
//ask the player if they would like to play again
System.out.println("Would you like to play again? Enter 1 for yes and 2 for no.");
playAgain = input.nextInt();
if (playAgain == 1) {
System.out.println("Let's play again!");
continue;
}
else {
System.out.println("Thanks for playing! Goodbye!");
break;
}
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
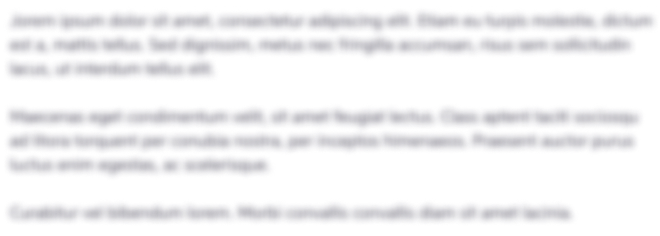
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started