Question
My previous tasks below : Task 2 : Complete the following function template: function PermuteVector(row, p) if p = 0 then return row end if
My previous tasks below :
Task 2 : Complete the following function template: function PermuteVector(row, p) if p = 0 then return row end if new Queue q ... end function This function should take a four-element vector called row as an input parameter and return that vector but with its values cyclically permuted by p elements to the left: p should be a number between 0 and 3 (inclusive). To be able to get full marks you need to use the queue abstract data structure appropriately as outlined above. [5 marks]
Answer:
function PermuteVector(row, p)
if p = 0 then
return row
end if
new Queue q
p = p mod 4
For each element x in row
Enqueue(q, x)
End for
For i from 1 to p
x = Dequeue(q)
Enqueue(q,x)
End for
For i from 0 to 3
x = Dequeue(q)
row[i] = x
End for
Return row
Task 3 : Complete the following function template: function PermuteRows(puzzle, x, y, z) ... end function This function should take a four-element vector called puzzle, which will be of the form of the output of MakeVector as an input parameter, as well as three integers x, y and z. The function will return puzzle but with elements puzzle[1], puzzle[2] and puzzle[3] cyclically permuted by x, y and z elements respectively to the left: x, y and z should all be numbers between 0 and 3 (inclusive). To be able to get full marks you should call the function PermuteVector appropriately. HINT: You do not need to loop over integers x, y and z. [3 marks]
Answer:
function PERMUTEROW(puzzle,x,y,z)
deque dq
//deque
for 0
dq.pushBack(puzzle[i])
end for
for 0
temp=dq.back()
dq.popBack()
dq.pushFront(temp)
end for
for 0
puzzle[i]=dq.front()
dq.popFront()
end for
return puzzle
end function
Task 4 : Complete the following function: function SearchStack(stack, item) ... end function This function will take a stack and a value (called item) as input parameters, and return FALSE if item is not stored in the stack, otherwise return the stack without the element storing item. [6 marks]
Answer:
//function that search an item in a stack and returns
//true if element is found else false
bool SearchStack(stack
{
//initializing the flag variable as false
bool flag = false;
//searching in stack until it not empty
while (!stack.empty())
{
//if the element is equal to the given itme
//then flag becomes true and then return the flag
if (stack.top() == item){
flag = true;
return flag;
}
//removing the top element to get next element
stack.pop();
}
return flag;
}
//utility function to display stack elements
void display(stack
{
while (!stack.empty())
{
cout
stack.pop();
}
cout
}
int main()
{
//creating a stack numbers of integer
stack
int value;
//inserting elements in stack
numbers.push(1);
numbers.push(8);
numbers.push(10);
numbers.push(2);
numbers.push(5);
numbers.push(4);
cout
display(numbers);
//taking user input for value
cout
cin>>value;
//if function return true then element is found
if(SearchStack(numbers, value)) {
cout
}
//else element is not ffound
else{
cout
}
return 0;
}
Task 5 : Complete the following function: function CheckColumn(puzzle, j) ... end function This function will take the vector puzzle (as produced by MakeVector) as an input parameter and check that column j contains all numbers from 1 to 4: if it does contain all numbers from 1 to 4, it should return TRUE, otherwise it should return FALSE. The procedure you should use is the one outlined above. To get full marks you need to call SearchStack(stack, item). [4 marks]
Answer:
Make a vector puzzle of length 4
Use for circle from 0 to 4
Add a column vector inside every component of the vector puzzle
For I := 0 to 4
puzzle.push_back(row)
End For
Return puzzle vector
MAKEVECTOR(row)
new Vector puzzle(4)
For I := 0 to 4
puzzle.push_back(row)
End For
bring puzzle back
Task 6 : Complete the following function: function CheckGrids(puzzle) ... end function This function will take the vector puzzle (as produced by MakeVector) as an input parameter and check that all sub-grids contain all numbers from 1 to 4: if every sub-grid does contain all numbers from 1 to 4, it should returnTRUE, otherwise it should return FALSE. For each sub-grid you should create a stack with numbers from 1 to 4, and then repeatedly search the stack to see if the values in the sub-grid are stored there. To get full marks you need to call SearchStack(stack, item). [6 marks]
Answer:
function CHECKGRIDS(puzzle):
for 0
stack
for 0
if SEARCHSTACK(st,puzzle[i][j])==FALSE:
return FALSE
end if
end for
end for
return TRUE
end function
Task 7 : Consider the following puzzle vector: Design and explain a concrete data structure that implements this puzzle vector. The data structure must only consist of elements that can store an integer or a pointer to another element or null- elements can be indexed if they are contiguous in memory as with an array. You can draw the data structure and explain how the allowed operations of vectors are implemented on this concrete data structure- additional pointers can be created to traverse lists. One approach could be to use arrays, or linked lists, or another approach completely. [6 marks]
Answer:
#include
using namespace std;int main()
{
ios::sync_with_stdio(false);
int row, col;
cin>>row>>col;vector
v.resize(col,vector
for(int i=0; i { for(int j=0; j { cin>>v[i][j]; } }for(int i=0;i { for(int j=0;j { cout } } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
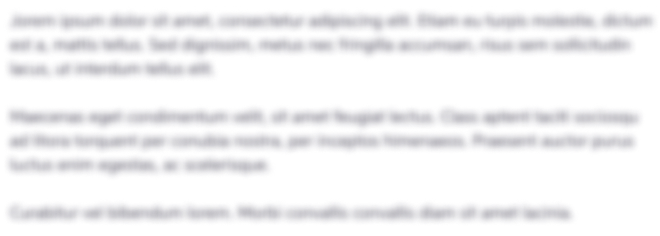
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started