Question
My program is having issues and will fail tests once run. Please help me debug the guessme.java class. Thanks. I will supply a text format
My program is having issues and will fail tests once run. Please help me debug the guessme.java class. Thanks. I will supply a text format of all three java files but can only do so in the comments since it is too long of a question to post.
GuessMe.java:
import java.util.Random; /* Remember to import java.util.Random, otherwise you will get errors! */
public class GuessMe {
/* Here you will be creating your instance variables. When making instance variables, we think * about what information we need to store between method calls. The info we will need to store * is: * * 1) the word the user is trying to guess. We will need a String instance variable called 'word' for that. * * 2) the number of guesses the user has left. We will need an int called 'remainingGuesses' for that. * 3) we need to know if the word has been found. We will need a boolean called 'wordFound' for that. * 4) we need a random number generator. We will need a Random object called 'rand' for that. * * Be sure to use the suggested variable names exactly, or you won't pass many of the tests. */ private String word; private int remainingGuesses; private boolean wordFound; private static Random rand;
//Instance variables here:
/* This is the first of two constructors. This one is used in the main method. We initialize each * of our instance variables here. The player will start off with 10 guesses, the word obviously * has not been found yet. You will need to initialize 'rand' before you can generate the word, and * you will want to use the generateWord() helper method at the bottom of this file to initialize * 'word' that way you don't have to write the same code a ton of times. */ public GuessMe() {
remainingGuesses = 10; wordFound = false; rand = new Random(); word = generateWord(); }
/* This is our second constructor. There is only one real difference: in this one we take in an * int which will act as the seed for our Random object. This is used for the tests. */ public GuessMe(int seed) { remainingGuesses = 10; wordFound = false; rand = new Random(seed); word = generateWord(); }
/* This is our play method. It takes in a String called 'str' and compares it to the word the * player is trying to guess 'word'. It returns an int representing the number of letters which * are in the exact same place in both words. For example, if the word to find were "ABCD" play * would return: * * 1 on "AAAA" * 2 on "ABAA" * 2 on "AAAD" * 4 on "ABCD" * 4 on "abcd" * * NOTE these values are ONLY for the example word "ABCD" and will not be the same for your random * word. Looping and using charAt() on the strings would be very useful here. Remember we do not * care about upper/lower case here, so be sure to account for that. * * We also want to decrement the user's remaining guesses, and in the event they guess the word * we will also update wordFound to true before returning 4. */ public int play(String str) {
int matches = 0; matches += word.charAt(0) == str.charAt(0) ? 1 : 0; matches += word.charAt(1) == str.charAt(1) ? 1 : 0; matches += word.charAt(2) == str.charAt(2) ? 1 : 0; matches += word.charAt(3) == str.charAt(3) ? 1 : 0; remainingGuesses -= 1; if(matches==4){ wordFound=true; } return matches; }
/* isOver simply returns true if the game is over, which is either when the player runs * out of guesses or when they have found the correct word. It returns false otherwise. */ public boolean isOver() { return remainingGuesses == 0 || wordFound; }
/* isWin returns true if the player has correctly guessed the word and false otherwise. */ public boolean isWin() { return wordFound == true;
}
/* getRemainingGuesses returns the number of remaining guesses the player has. */ public int getRemainingGuesses() { return remainingGuesses; }
/* reset returns nothing but resets the game. 'remainingGuesses' and 'wordFound' go back to * their default values and a new word is generated with generateWord(). */ public void reset() { word=generateWord(); remainingGuesses = 10; wordFound = false; }
/* getWord simply returns the word the user is attempting to guess. It is not tested, * and is only used in the main method. */ public String getWord() {
return word;
}
/**********************************/ /* Helper Methods */
/**********************************/
/* A helper method is a private method which cannot be accessed outside of the file. Its * sole purpose is to be used in other constructors/methods and simply 'helps' them do their * job better. In our case, we need to generate a random word occassionally, so we make * a helper method so that we don't have to write the same code repeatedly. * * We will go about writing this by using our Random object. We will call nextInt(4) on our * Random object a total of four times to generate four different ints. Specifying 4 in the * method call forces it to generate an int between 0 and 4, including 0 but not including 4. * The ints correspond to a letter to add to our word as such: * * 0: 'A' * 1: 'B' * 2: 'C' * 3: 'D' * * We can build our String by starting with local variable which is an empty String ("") which we can * call retStr. Using String concatination (+=), we can add letters to our String based on the int * generated. Once we have done this 4 times, we can return the String. We do not use any instance * variables in this method. It may be helpful to use a loop here. */ private String generateWord() { String aRandomWord = ""; for (int i = 0; i
1 Lmport java.util. 3 public class GuessMeMain 5 public static void main(String args)t /Here we declare and initialize our scanner Scanner scan-new Scanner(System.in) //Here we declare and initialize our play again" boolean boolean againtrue; Systen.out-println( "Welcone to Guess Me!") Syster out printin A random word will be generated. It will be four letters long andinconsist of the letters A'. B GuessMe gane-ne Guesse) 13 C and D Your goal is to guess the word within the Blloted gues ses Good luck ! " ; 16 while(again)t while(lgame. 1soverO) Systen.out.println(You have"+ game.getRemainingGuesses (uesses left. System.out.printin( Enter your guess:") String guess - while(true)t 19 guess scan.nextline); if(guess.length)-4) 25 systen . out-Print1n("Invalid guess, please enter a 4 letter word consisting of 'A', B , and .D':"); 27 int score- gane-play (Ruess); Systen.out.println( There are score correct letter placements.") if(gane.isin))( Systen.out.println( Congrats!") Systen.out.println( You 1ose! The word wasgane.getiord)) 35 36 Systen . out . print1n(-would you like to play again? y"); f (Character . toLowerCase(scan . nextLine().charAt(0)) .. 'n'){ 37 38 again false; gane.reset 41 43 Systen.out.printlnGoodbyel) 1 import java.util.*; 2 import org.junit.Assert; 3 import static org.junit.Assert.* 4 import org.junit.Before; 5 import org.junit.Test; 6 import java.lang.reflect.* 8 9 public class GuessMeTest f 10 11 private GuessMe game; 12 13* Fixture initialization (common initialization for all tests) / 15 @Before public void setUp) gamenew GuessMe (1234) 18 19* Ensures word variable exists */L 0 @Test public void instanceExistsTest1) String word tryt /Note that you do not need to understand how this test works. This is some *fancy stuff to get around the fact that these variables are private. You should not try to do this outside of testing, as it defeats the purpose of things being private/ Field wordField GuessMe.class.getDeclaredField( "word" wordField.setAccessible(true) word (String)wordField.get (game); Assert.assertEquals("word instance variable exists", 1, 1); 26 28 29 catch (Exception e) Assert.fail( "word instance variable doesn't exist"); 34 35 36 *Ensures remainingGuesses variable exists */ 37 @Test public void instanceExistsTest2) 38 39 int remainingGuesses1; tryt /Note that you do not need to understand how this test works. This is some *fancy stuff to get around the fact that these variables are private. You 41 42 43 14 45 46 should not try to do this outside of testing, as it defeats the purpose of *things being private*/ Field intField -GuessMe.class.getDeclaredField("remainingGuesses"); intField.setAccessible(true); remainingGuesses(Integer)intField.get (game); Assert.assertEquals("remainingGuesses instance variable exists", 1, 1); catch (Exception e) 48 49 50 Assert.fail("remainingGuesses instance variable does not exist"); Ensures wordFound variable exists 53 Ensures wordFound variable exists 54 @Test public void instanceExistsTest3() t boolean wordFound true; tryt 56 57 58 59 60 /Note that you do not need to understand how this test works. This is some *fancy stuff to get around the fact that these variables are private. You should not try to do this outside of testing, as it defeats the purpose of 62 63 64 65 *things being private*/ Field booleanField - GuessMe.class.getDeclaredField ("wordFound"); booleanField.setAccessible(true); wordFound(Boolean)booleanField.get(game); Assert.assertEquals("wordFound instance variable exists", 1, 1); catch (Exception e)t Assert.fail("wordFound instance variable does not exist"); 68 69 70 * Ensures rand variable exists*/ 71 @Test public void instanceExistsTest4() 72 73 74 75 76 Random rand null; tryt /Note that you do not need to understand how this test works. This is some fancy stuff to get around the fact that these variables are private. You *should not try to do this outside of testing, as it defeats the purpose of *things being private*/ Field randFieldGuessMe.class.getDeclaredField("rand"); randField.setAccessible(true); rand -(Random)randField.get (game); Assert.assertEquals("rand instance variable exists", 1, 1); 80 catch (Exception e)1t 82 83 84 85 86 87 Ensures constuctor initializes word correctly 88 @Test public void constructorTest1){ 89 90 91 92 93 94 95 96 Assert.fail("rand instance variable does not exist"); String word tryt /Note that you do not need to understand how this test works. This is some *fancy stuff to get around the fact that these variables are private. You should not try to do this outside of testing, as it defeats the purpose of things being private/ Field wordFieldGuessMe.class.getDeclaredField("word"); wordField.setAccessible(true); word(String)wordField.get (game) catch (Exception e)1 Assert.assertEquals("constructor initializes word correctly", "CBDA", word) 98 100 101 102Ensures constuctor initializes remainingGuesses correctly 103 @Test public void constructorTest2)f 104 105 int remainingGuesses- -1; tryt 102 *Ensures constuctor initializes remainingGuesses correctly/ 103 @Test public void constructorTest2) 104 105 106 107 108 109 110 int remainingGuesses- -1; tryt /Note that you do not need to understand how this test works. This is some *fancy stuff to get around the fact that these variables are private. You *should not try to do this outside of testing, as it defeats the purpose of *things being private*/ Field intField GuessMe.class.getDeclaredField("remainingGuesses") intField.setAccessible(true); remainingGuesses(Integer)intField.get (game); 112 113 114 115 116 117 Ensures constuctor initializes wordFound correctly**/ 118@Test public void constructorTest3) 119 catch (Exception e)fh Assert.assertEquals("constructor initializes word correctly", 10, remainingGuesses); boolean wordFound true; tryt 121 122 /Note that you do not need to understand how this test works. This is some fancy stuff to get around the fact that these variables are private. You *should not try to do this outside of testing, as it defeats the purpose of *things being private*/ Field booleanField- GuessMe.class.getDeclaredField("wordFound"); booleanField.setAccessible(true); wordFound(Boolean)booleanField.get(game); 126 catch (Exception e)fh Assert.assertEquals("constructor initializes word correctly", false, wordFound) 130 131 132 Ensures constuctor initializes rand correctly/ 133@Test public void constructorTest4) 134 135 136 137 138 Random rand null; tryt /Note that you do not need to understand how this test works. This is some fancy stuff to get around the fact that these variables are private. You should not try to do this outside of testing, as it defeats the purpose of *things being private*/ Field randFieldGuessMe.class.getDeclaredField("rand"); randField.setAccessible(true); rand(Random)randField.get (game) 140 141 142 143 144 145 146 147 148 149 Ensures constuctor initializes rand correctly/ 150 @Test public void constructorTest5) 151 catch (Exception e)f Assert.assertNotNull("Constructor initializes rand", rand); int i- rand.nextInt ); Assert.assertEquals("Constructor initializes rand correctly", -611652875, i); gamenew GuessMe) Random rand null; tryt 153 154 /Note that you do not need to understand how this test works. This is some Field randField-GuessMe.class.getDeclaredField("rand"); randField.setAccessible(true); rand(Random)randField.get (game); 158 159 160 161 162 163 164 165 166 *Ensures reset resets word correctly 167@Test public void resetTest1) 168 169 170 catch (Exception e) Assert.assertNotNull( "Constructor initializes rand", rand); int i- rand.nextInt (2); game.reset) String word tryt /Note that you do not need to understand how this test works. This is some 172 *fancy stuff to get around the fact that these variables are private. You *should not try to do this outside of testing, as it defeats the purpose of 174 175 176 *things being private/ Field wordField-GuessMe.class.getDeclaredField( "word"); wordField.setAccessible(true); word(String)wordField.get (game) catch (Exception e)th Assert.assertEquals("reset resets word correctly", "DBBC", word); 178 179 180 181 182 Ensures reset resets remainingGuesses correctly/ 183 @Test public void resetTest2) 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 *Ensures reset resets wordFound correctly*/ 199@Test public void resetTest3) 200 201 202 203 204 205 206 207 208 209 210 game.reset) int remainingGuesses1; tryt /Note that you do not need to understand how this test works. This is some *fancy stuff to get around the fact that these variables are private. You *should not try to do this outside of testing, as it defeats the purpose of *things being private/ Field intField GuessMe.class.getDeclaredField("remainingGuesses"); intField.setAccessible(true); remainingGuesses(Integer)intField.get (game); catch (Exception e)to Assert.assertEquals("reset resets remainingGuesses correctly", 10, remainingGuesses); boolean wordFound true; tryt /Note that you do not need to understand how this test works. This is some fancy stuff to get around the fact that these variables are private. You *should not try to do this outside of testing, as it defeats the purpose of things being private/ Field booleanField- GuessMe.class.getDeclaredField("wordFound"); booleanField.setAccessible(true); booleanField.set (game, true); game.reset ) wordFound(Boolean)booleanField.get (game); game.reset () wordFound(Boolean)booleanField.get (game); 209 210 211 212 213 214 215 /tests isOver on remainingGuesses**/ 216 @Test public void sOverTest1(){ 217 218 219 220 221 catch (Exception e){ Assert.assertEquals("reset resets wordFound correctly", false, wordFound); for(int = 0; i but was:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
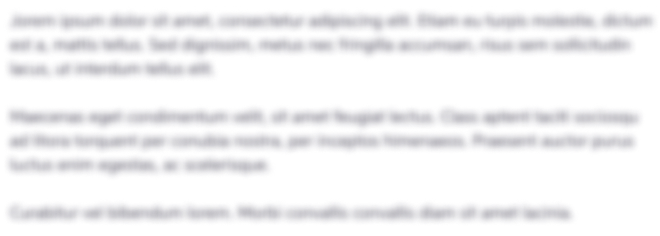
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started