Question
my python code is not working not sure why # Library Project import datetime import uuid class LibrarySystem: def __init__(self, list_of_books, library_name):
my python code is not working not sure why
# Library Project
import datetime
import uuid
class LibrarySystem:
def __init__(self, list_of_books, library_name):
self.list_of_books = list_of_books
self.list_of_books = "list_of_books.txt"
self.library_name = library_name
self.books_dict = {}
with open(self.list_of_books) as bk:
content = bk.readlines()
for line in content:
books_title, author, category = line.strip().split(",")
book_id = str(uuid.uuid4()) # generate a unique ID
self.books_dict.update({book_id: {"book_title": books_title, "author": author, "category": category,
"quantity": 5, "availability": "yes"}})
def display_books(self):
print("-----------------------------------List Of Books----------------------------")
print("Book ID", "t", "Title", "ttt", "Author", "tt", "Category", "tt", "Quantity", "t",
"Availability")
print("----------------------------------------------------------------------------")
for key, value in self.books_dict.items():
print(key, "tt", value.get("book_title"), "tt", value.get("author"), "t", value.get("category"),
"tt", value.get("quantity"), "tt", value.get("availability"))
def rent_books(self):
rented_books = []
while True:
search_criteria = input("Enter book ID, title, author, or category to search for a book (q to quit): ")
if search_criteria == 'q':
break
found_books = []
for book_id, book_info in self.books_dict.items():
if search_criteria.lower() in book_info['book_title'].lower() or search_criteria.lower() in book_info[
'author'].lower() or search_criteria.lower() in
book_info['category'].lower() or search_criteria == book_id:
found_books.append((book_id, book_info))
if not found_books:
print(f"No books found for '{search_criteria}'")
else:
print("Found books:")
print("Book ID", "t", "Title", "ttt", "Author", "tt", "Category", "tt", "Quantity", "t",
"Availability")
print("----------------------------------------------------------------------------")
for book_id, book_info in found_books:
print(book_id, "tt", book_info['book_title'], "tt", book_info['author'], "t",
book_info['category'], "tt", book_info['quantity'], "tt", book_info['availability'])
book_id = input("Enter the book ID to rent (q to quit): ")
if book_id == 'q':
break
elif book_id not in self.books_dict.keys():
print("Invalid book ID. Please enter a valid book ID.")
elif self.books_dict[book_id]['availability'] == 'no':
print("This book is currently not available. Please choose another book or try again later.")
elif int(self.books_dict[book_id]['quantity']) == 0:
print(
"Sorry, all copies of this book have been rented out."
" Please choose another book or try again later.")
else:
lender_name = input("Enter your name: ")
issue_date = datetime.date.today()
self.books_dict[book_id]['lender_name'] = lender_name
self.books_dict[book_id]['issue_date'] = issue_date
self.books_dict[book_id]['quantity'] = str(int(self.books_dict[book_id]['quantity']) - 1)
if int(self.books_dict[book_id]['quantity']) == 0:
self.books_dict[book_id]['availability'] = 'no'
rented_books.append(self.books_dict[book_id]['book_title'])
print(
f"Congratulations! You have rented the book '"
f"{self.books_dict[book_id]['book_title']}' successfully !!!")
another_rental = input("Do you want to rent another book? (y/n) : ")
if another_rental.lower() == 'n':
self.print_receipt(rented_books)
break
def add_books(self):
books_title = input("Enter Book Title : ")
author = input("Enter Author Name : ")
category = input("Enter Book Category : ")
quantity = input("Enter Book Quantity : ")
if books_title == "" or author == "" or category == "" or quantity == "":
print("Please enter valid input for book title, author, category and quantity!!!")
return self.add_books()
else:
with open(self.list_of_books, "a") as b:
b.writelines(f"{books_title},{author},{category}")
book_id = str(uuid.uuid4()) # generate a unique ID
self.books_dict.update({book_id: {"book_title": books_title, "author": author,
"category": category, "quantity": quantity, "availability": "yes"}})
print(f"The book '{books_title}' has been added successfully !!!")
def return_books(self):
while True:
book_id = input("Enter the book ID to return (6 to quit): ")
if book_id == '6':
break
elif book_id not in self.books_dict.keys():
print("Invalid book ID. Please enter a valid book ID.")
elif self.books_dict[book_id]['availability'] == 'yes':
print("This book is not currently rented. Please enter a valid book ID.")
else:
lender_name = self.books_dict[book_id]['lender_name']
issue_date = self.books_dict[book_id]['issue_date']
due_date = issue_date + datetime.timedelta(days=30)
return_date = datetime.date.today()
days_late = (return_date - due_date).days if return_date > due_date else 0
late_fee = days_late * 0.10
self.books_dict[book_id]['lender_name'] = ''
self.books_dict[book_id]['issue_date'] = ''
self.books_dict[book_id]['quantity'] = str(int(self.books_dict[book_id]['quantity']) + 1)
self.books_dict[book_id]['availability'] = 'yes'
print(f"Thank you for returning the book '{self.books_dict[book_id]['book_title']}'")
print("Lender Name: ", lender_name)
print("Issue Date: ", issue_date.strftime("%Y-%m-%d"))
print("Due Date: ", due_date.strftime("%Y-%m-%d"))
print("Return Date: ", return_date.strftime("%Y-%m-%d"))
print("Days Late: ", days_late)
print("Late Fee: $", late_fee)
return_option = input("Do you want to return another book? (y/n): ")
if return_option.lower() == 'n':
break
def search_books(self):
while True:
search_criteria = input("Enter a book title, author, or category to search for a book (q to quit): ")
if search_criteria == 'q':
break
found_books = []
for book_id, book_info in self.books_dict.items():
if search_criteria.lower() in book_info['book_title'].lower() or search_criteria.lower() in
book_info['author'].lower() or search_criteria.lower() in book_info['category'].lower():
found_books.append((book_id, book_info))
if not found_books:
print(f"No books found for '{search_criteria}'")
else:
print("Found books :")
print(" Book ID", "t", "Title", "ttt", "Author", "tt", "Category", "tt", "Quantity", "t",
"Availability")
print("----------------------------------------------------------------------------")
for book_id, book_info in found_books:
print(book_id, "tt", book_info['book_title'], "tt", book_info['author'], "t",
book_info['category'], "tt", book_info['quantity'], "tt", book_info['availability'])
rent_option = input("Do you want to rent a book? (y/n): ")
if rent_option.lower() == 'y':
book_id = input("Enter the book ID to rent: ")
if book_id in self.books_dict.keys() and self.books_dict[book_id]['availability'] == 'yes':
lender_name = input("Please Enter your name: ")
issue_date = datetime.date.today()
self.books_dict[book_id]['lender_name'] = lender_name
self.books_dict[book_id]['issue_date'] = issue_date
self.books_dict[book_id]['quantity'] = str(int(self.books_dict[book_id]['quantity']) - 1)
if self.books_dict[book_id]['quantity'] == '0':
self.books_dict[book_id]['availability'] = 'no'
print(
f"Congratulations! You have rented the book '{self.books_dict[book_id]['book_title']}' "
f"successfully !!!")
return
else:
print("Invalid book ID or the book is not available for rent. Please enter a valid book ID.")
else:
return
def print_receipt(self, rented_books):
print("--------------------- Receipt ---------------------")
print("Book Title", "ttt", "Return Due Date")
print("---------------------------------------------------")
due_date = datetime.date.today() + datetime.timedelta(days=30)
for book_title in rented_books:
print(book_title, "ttt", due_date.strftime("%Y-%m-%d"))
print("---------------------------------------------------")
if __name__ == "__main__":
try:
mylms = LibrarySystem("list_of_books.txt", "Python's")
press_key_list = {"1": "Display Books", "2": "Rent Books", "3": "Donate Books", "4": "Return Books",
"5": "Search Books", "6": "Exit"}
key_press = False
while not (key_press == "6"):
print(f"-------------Welcome TO {mylms.library_name}'s Library System---------------")
for key, value in press_key_list.items():
print("Press", key, "To", value)
key_press = input("Press Key : ").lower()
if key_press == "1":
print("Current Selection : Display Books")
mylms.display_books()
elif key_press == "2":
print("Current Selection : Rent Books")
mylms.rent_books()
elif key_press == "3":
print("Current Selection : Donate Books")
mylms.add_books()
elif key_press == "4":
print("Current Selection : Return Books")
mylms.rent_books()
elif key_press == "5":
print("Current Selection : Search Books")
mylms.search_books()
elif key_press == "6":
break
else:
continue
except Exception:
print("Something went wrong. Please check again !!!!!")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Answer Corrected code import datetime import uuid class LibrarySystem def initself listofbooks libraryname selflistofbooks listofbooks selflibraryname libraryname selfbooksdict with openselflistofbook...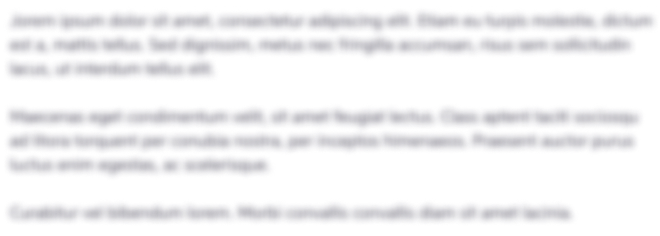
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started