Question
My question is Create a class called Date that includes 3 instance variablesa month (type int), a day (type int), and a year (type int).
My question is Create a class called Date that includes 3 instance variablesa month (type int), a day (type int), and a year (type int). Provide a constructor that initializes the 3 instance variables and assumes the values provided are correct. Provide a set and a get method for each instance variable. Provide a method displayDate that displays the month, day, and year separated by forward slashes(/). Write a test application named DateTest that demonstrates class Dates capabilities.
My code is
public class Date {
int month;
int day;
int year;
public Date(int month, int day, int year) {
this.month = month;
this.day = day;
this.year = year;
}
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
public int getDay() {
return day;
}
public void setDay(int day) {
this.day = day;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public void displayDate() {
System.out.println(month + "/" + day + "/" + year);
}
}
public class DateTest {
public static void main(String[] args) {
Date day = new Date(4,16,2012);
day.displayDate();
System.out.println("Month: " + day.getMonth());
System.out.println("Day: " + day.getDay());
System.out.println("Year: " + day.getYear());
day.setMonth(12);
day.setDay(25);
day.setYear(2011);
day.displayDate();
}
}
This was given when someone previously answered, however this code does not work. Please help
Step by Step Solution
There are 3 Steps involved in it
Step: 1
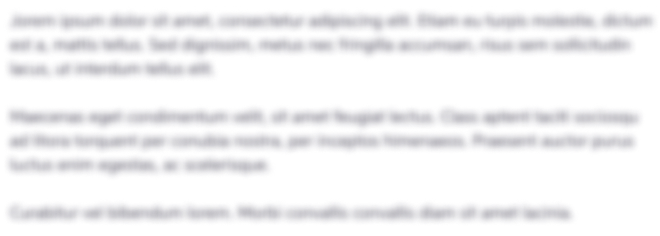
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started