Question
my quicksort algorithm is outputting for out_abc10b: abb abb acd acd b b baa baa c c ca ca il il zr zr zza zza
my quicksort algorithm is outputting for out_abc10b:
abb abb acd acd b b baa baa c c ca ca il il zr zr zza zza zzz zzz and
I want it to output:
abb acd b baa c ca il zr zza zzz
#include
using namespace std;
const int MAX_VAL = 262627;
int converter(string word) { int result = 0; int num; int length = word.length();
transform(word.begin(), word.end(), word.begin(), ::tolower);
for (int i = 0; i < length; i++) { char ch = word[i]; num = (((int)ch) - 96); result = result * 100 + num; }
if (length == 1) { result *= 10000; } else if (length == 2) { result *= 100; }
return result; }
string decoder(int encodedValue) { int num; string result;
while (encodedValue > 0) { num = encodedValue % 100; result = char(num + 96) + result; encodedValue /= 100; }
return result; }
void countingSort(vector
for (int i = 0; i < words.size(); i++) { count[words[i].first]++; }
for (int i = 1; i <= MAX_VAL; i++) { count[i] += count[i - 1]; }
for (int i = words.size() - 1; i >= 0; i--) { result[count[words[i].first] - 1] = words[i]; count[words[i].first]--; }
words = result; }
bool stringCompare(const string& a, const string& b) { return a.length() < b.length() || (a.length() == b.length() && a < b); }
int partition(vector
for (int j = low; j <= high - 1; j++) { if (words[j].first < pivot) { i++; swap(words[i], words[j]); } } swap(words[i + 1], words[high]); return (i + 1); }
void quickSort(vector
int main() { string word, maxValue; vector
inFile.open("in_abc10.txt"); if (!inFile) { cerr << "Unable to open file"; exit(1); }
getline(inFile, maxValue); // exclude the first line from input file
while (inFile >> word) { transform(word.begin(), word.end(), word.begin(), ::tolower); int encodedValue = converter(word); words.push_back(make_pair(encodedValue, word)); }
//Sort using counting sort and write the result to out_abc10a.txt countingSort(words);
outFile1.open("out_abc10a.txt"); if (!outFile1) { cerr << "Unable to open file"; exit(1); }
for (int i = 0; i < words.size(); i++) { outFile1 << words[i].second; if (i < words.size() - 1) { outFile1 << " "; } }
outFile1.close(); //sort using the bucket sort and write result to out_abc10b.txt inFile.clear(); inFile.seekg(0, ios::beg);
inFile2.open("in_abc10.txt"); if (!inFile2) { cerr << "Unable to open file"; exit(1); }
getline(inFile2, maxValue); //exclude the first line from input file
while (inFile2 >> word) { transform(word.begin(), word.end(), word.begin(), ::tolower); int encodedValue = converter(word); words.push_back(make_pair(encodedValue, word)); }
quickSort(words, 0, words.size() - 1);
outFile2.open("out_abc10b.txt"); if (!outFile2) { cerr << "Unable to open file"; exit(1); } for (int i = 0; i < words.size(); i++) { cout << words[i].second << " "; outFile2 << words[i].second; if (i < words.size() - 1) { outFile2 << " "; } }
inFile.close(); outFile1.close(); outFile2.close(); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
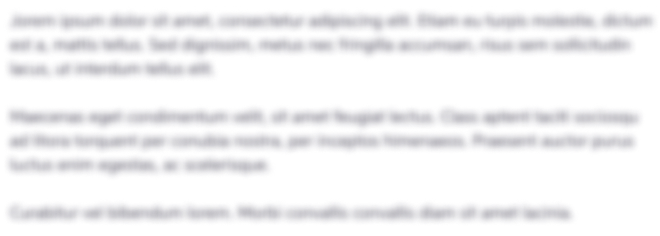
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started