Answered step by step
Verified Expert Solution
Question
1 Approved Answer
need an explanation, or a report where I can simply understand how this code works. I am learning Java and have some programming knowledge. My
need an explanation, or a report where I can simply understand how this code works. I am learning Java and have some programming knowledge. My professors told us to evaluate this code and discover how every line interacts in between. So I would be glad if someone could explain me in detail how this code works using JavaFX Graphics
App.java
/* Imports */ import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.canvas.Canvas; import javafx.scene.canvas.GraphicsContext; import javafx.scene.layout.StackPane; import javafx.stage.Stage; /* Driver class */ public class App extends Application { // Sets boundaries of stage final int WIDTH = 1000; final int HEIGHT = 600; @Override public void start(Stage primaryStage) { try { // Sets up primary stage, canvas, and graphics context primaryStage.setTitle("Assignment 1"); Canvas canvas = new Canvas(WIDTH, HEIGHT); GraphicsContext gc = canvas.getGraphicsContext2D(); // Draws alternate ovals and rectangles of random colors double width = 500, height = 300, centerX = WIDTH / 2, centerY = HEIGHT / 2; MyOval oval; MyRectangle rect; rect = new MyRectangle(centerX - width / 2, centerY - height / 2, width, height, MyColor.getRandom()); rect.draw(gc); System.out.println(rect + " "); for (int i = 1; i < 6; ++i) { if (i % 2 != 0) { oval = new MyOval(centerX - width / 2, centerY - height / 2, width, height, MyColor.getRandom()); oval.draw(gc); System.out.println(oval + " "); } else { width /= Math.sqrt(2); height /= Math.sqrt(2); rect = new MyRectangle(centerX - width / 2, centerY - height / 2, width, height, MyColor.getRandom()); rect.draw(gc); System.out.println(rect + " "); } } // Finishing off with desired horizontal line and slightly thick window border MyLine line1 = new MyLine(0, 0, WIDTH, HEIGHT, MyColor.BLACK.getCol()); line1.draw(gc); System.out.println(line1 + " "); gc.setLineWidth(5); gc.strokeRect(0, 0, WIDTH, HEIGHT); // Sets stack pane and scene for image to appear on StackPane root = new StackPane(canvas); Scene scene = new Scene(root, WIDTH, HEIGHT); primaryStage.setScene(scene); primaryStage.show(); } catch (Exception e) { e.printStackTrace(); } } public static void main(String[] args) { launch(args); } }
My Point.java
import javafx.scene.canvas.GraphicsContext; public class MyPoint { private double x; private double y; public MyPoint(double x, double y) { this.x = x; this.y = y; } public double getX() { return x; } public double getY() { return y; } public void setX(double x) { this.x = x; } public void setY(double y) { this.y = y; } @Override public String toString() { return String.format("----- Point Properties ----- %15s (%.2f,%.2f)", "Point:", x, y); } public void draw(GraphicsContext gc) { gc.setFill(MyColor.BLACK.getCol()); gc.fillOval(x, y, 1, 1); } }
MyColor.java
import javafx.scene.paint.Color; public enum MyColor { RED(255, 0, 0, 255), BLUE(0, 0, 255, 255), LIME(0, 255, 0, 255), CYAN(0, 255, 255, 255), GREEN(0, 128, 0, 255), GREY(128, 128, 128, 255), MAGENTA(255, 0, 255, 255), PURPLE(128, 0, 128, 255), VIOLET(148, 0, 211, 255), YELLOW(255, 255, 0, 255), WHITE(255, 255, 255, 255), BLACK(0, 0, 0, 255), HOTPINK(255, 105, 180, 255), ORANGE(255, 165, 0, 255); private int r, g, b, a; // Value for red, green, blue, and opacity /* Random color picker from color library */ public static Color getRandom() { int r, g, b; r = (int) ((Math.random() * (255))); g = (int) ((Math.random() * (255))); b = (int) ((Math.random() * (255))); return Color.rgb(r, g, b, 1); } MyColor(int r, int g, int b, int a) { this.r = r; this.g = g; this.b = b; this.a = a; } public Color getCol() { return Color.rgb(r, g, b, (double) (a / 255)); } }
MyShape.java
import javafx.scene.canvas.GraphicsContext; import javafx.scene.paint.Color; import java.util.ArrayList; import java.util.HashSet; // implements MyShapeInterface public abstract class MyShape implements MyShapeInterface { private MyPoint point; // Point of shape in pixels private Color color; MyShape(double x, double y, Color color) { this.point = new MyPoint(x, y); this.color = color; } public double getX() { return point.getX(); } public double getY() { return point.getY(); } public Color getColor() { return color; } public void setX(int x) { this.point.setX(x); } public void setY(int y) { this.point.setY(y); } public void setColor(Color color) { this.color = color; } @Override public ArrayList overlapMyShapes(MyShape shape) { ArrayList overlap = new ArrayList<>(); HashSet pointStr = new HashSet<>(); for (MyPoint point : shape.getMyArea()) { pointStr.add(point.toString()); } for (MyPoint point : this.getMyArea()) { if (pointStr.contains(point.toString())) { overlap.add(point); } } return overlap; } public abstract String toString(); public abstract void draw(GraphicsContext gc); }
MyLine.java
import javafx.scene.canvas.GraphicsContext; import javafx.scene.paint.Color; import java.util.ArrayList; public class MyLine extends MyShape { private final double x1, y1, x2, y2; // Coordinates of two points of line MyLine(double x1, double y1, double x2, double y2, Color color) { super(0, 0, color); this.x1 = x1; this.y1 = y1; this.x2 = x2; this.y2 = y2; } public double getLength() { return Math.sqrt(Math.pow(x2 - x1, 2) + Math.pow(y2 - y1, 2)); } public static double getLength(double x1, double y1, double x2, double y2) { return Math.sqrt(Math.pow(x2 - x1, 2) + Math.pow(y2 - y1, 2)); } public double get_xAngle() { double angle = Math.toDegrees(Math.atan2(y2 - y1, x2 - x1)); if (angle < 0) { angle += 360; } return angle; } @Override public String toString() { return String.format( "----- Line Properties ----- %15s (%.2f,%.2f) %15s (%.2f,%.2f) %15s %.2f %15s %.2f %15s " + super.getColor(), "Point 1:", x1, y1, "Point 2:", x2, y2, "Line Length:", getLength(), "Angle:", get_xAngle(), "Color:"); } @Override public MyRectangle getMyBoundingRectangle() { double cornerX, cornerY; cornerX = Math.min(x1, x2); cornerY = Math.min(y1, y2); return new MyRectangle(cornerX, cornerY, Math.abs(x2 - x1), Math.abs(y2 - y1), super.getColor()); } @Override public ArrayList getMyArea() { ArrayList set = new ArrayList<>(); double m = (y2 - y1) / (x2 - x1), intersect = y1 - (m * x1); MyRectangle bound = getMyBoundingRectangle(); double len = getLength(), bX = bound.getX(), bY = bound.getY(); for (double x = bX; x <= bX + bound.getWidth(); ++x) for (double y = bY; y <= bY + bound.getHeight(); ++y) if (Math.abs(y - (m * x + intersect)) < 1) set.add(new MyPoint(x, y)); if (Math.abs(get_xAngle() - 90) < 1) { for (int i = 0; i < len; ++i) set.add(new MyPoint(x1, y1 + i)); } else if (Math.abs(get_xAngle() - 270) < 1) { for (int i = 0; i < len; ++i) set.add(new MyPoint(x1, y1 - i)); } return set; } @Override public void draw(GraphicsContext gc) { gc.setStroke(super.getColor()); gc.strokeLine(x1, y1, x2, y2); } }
MyRectangle.java
import javafx.scene.canvas.GraphicsContext; import javafx.scene.paint.Color; import java.util.ArrayList; public class MyRectangle extends MyShape { private double width; private double height; // (x,y) Top Left Corner MyRectangle(double x, double y, double width, double height, Color color) { super(x, y, color); this.width = width; this.height = height; } public double getWidth() { return width; } public double getHeight() { return height; } public double getPerimeter() { return 2 * (width + height); } private double getArea() { return width * height; } public void setWidth(int width) { this.width = width; } public void setHeight(int height) { this.height = height; } @Override public void draw(GraphicsContext gc) { gc.setFill(super.getColor()); gc.setStroke(super.getColor()); gc.strokeRect(super.getX(), super.getY(), width, height); gc.fillRect(super.getX(), super.getY(), width, height); } @Override public MyRectangle getMyBoundingRectangle() { return this; } @Override public ArrayList getMyArea() { ArrayList set = new ArrayList<>(); // Picks up points from every column and row within rectangle, starting from top // left to bottom right for (double x = super.getX(); x <= super.getX() + width; ++x) { for (double y = super.getY(); y <= super.getY() + height; ++y) { set.add(new MyPoint(x, y)); } } return set; } @Override public String toString() { return String.format( "----- Rectangle Properties ----- %15s (%.2f,%.2f) %15s %.2f %15s %.2f %15s %.2f %15s %.2f %15s " + super.getColor(), "Corner Point:", super.getX(), super.getY(), "Area:", getArea(), "Perimeter:", getPerimeter(), "Width:", width, "Height:", height, "Color:"); } }
MyOval.java
import javafx.scene.canvas.GraphicsContext; import javafx.scene.paint.Color; import java.util.ArrayList; public class MyOval extends MyShape { private final MyPoint center; private double width; private double height; MyOval(double x, double y, double width, double height, Color color) { // (x,y) is top left corner super(x, y, color); this.width = width; this.height = height; this.center = new MyPoint(x + width / 2, y + height / 2); } public double getArea() { return Math.PI * width * height * 0.5; } public double getPerimeter() { return 2 * Math.PI * Math.sqrt((width + height) / 2); } public MyPoint getCenter() { return center; } public double getRadius(double angle) { double semiW = width / 2; double semiH = height / 2; double temp = Math.pow(semiW, 2) * Math.pow(Math.sin(Math.toRadians(angle)), 2); temp += Math.pow(semiH, 2) * Math.pow(Math.cos(Math.toRadians(angle)), 2); return (semiH * semiW) / (Math.sqrt(temp)); } public void setAxes(double width, double height) { this.width = width; this.height = height; } public void setCenter(double x, double y) { this.center.setX(x); this.center.setY(y); } @Override public String toString() { return String.format( "----- Oval Properties ----- %15s (%.2f,%.2f) %15s %.2f %15s %.2f %15s %.2f %15s %.2f %15s " + super.getColor(), "Center:", center.getX(), center.getY(), "Area:", getArea(), "Perimeter:", getPerimeter(), "Width:", width, "Height:", height, "Color:"); } @Override public MyRectangle getMyBoundingRectangle() { return new MyRectangle(super.getX(), super.getY(), width, height, super.getColor()); } @Override public ArrayList getMyArea() { ArrayList set = new ArrayList<>(); double horizonLen = width / 2; double verticalLen = height / 2; double dx, dy; for (double x = getX(); x <= getX() + width; ++x) { for (double y = getY(); y <= getY() + height; ++y) { dx = Math.abs(x - center.getX()); dy = Math.abs(y - center.getY()); if (Math.pow((dx) / horizonLen, 2) + Math.pow((dy) / verticalLen, 2) <= 1) { // Accounts for 4 quadrants of oval set.add(new MyPoint(x, y)); set.add(new MyPoint(x - 2 * dx, y - 2 * dy)); set.add(new MyPoint(x - 2 * dx, y)); set.add(new MyPoint(x, y - 2 * dy)); } } } return set; } @Override public void draw(GraphicsContext gc) { gc.setFill(super.getColor()); gc.setStroke(super.getColor()); gc.strokeOval(super.getX(), super.getY(), width, height); gc.fillOval(super.getX(), super.getY(), width, height); } }
Step by Step Solution
★★★★★
3.49 Rating (159 Votes )
There are 3 Steps involved in it
Step: 1
Sure Ill provide an explanation of how the code works focusing on how each class interacts with each other and the main application class App Ill use JavaFX graphics to illustrate the explanation App ...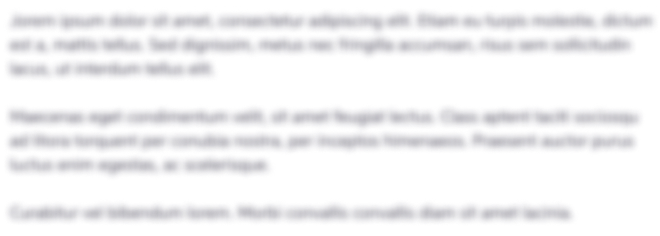
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started