Question
Need C++ code ASAP 50 mins left Create a custom class called WishList that manages a dynamically-allocated collection of gifts. Each gift will be stored
Need C++ code ASAP 50 mins left
Create a custom class called WishList that manages a dynamically-allocated collection of gifts.
Each gift will be stored as an object of type std::string and will have the format SKU##NAME. The SKU is the identifier that the store assigns to an item it sells, and NAME is a human-readable name of the item; the characters ## act as a separator between those two components. A string is considered a valid gift if it has the format described above.
The class should have at least the following members:
- a dynamically allocated array of gifts
- a default constructor
- an overload for += operator that receives as parameter an std::string.
- if the parameter doesn't represent a valid gift, raise an exception to inform the client (see in the sample output how the exception message should look)
- if the parameter is a valid gift, but is already added in the current object, this function does nothing
- if the parameter is a valid gift that doesn't exist in the current object, then add the gift to the array of gifts (and resize the array attribute)
- a query named display that receives as parameter one of the global functions printId or printName (see below).
- This query uses the parameter to print the gifts stored in the current object if the gift array is not empty, otherwise it prints the text "Empty list!".
- If the parameter is not valid, terminate the program with the error code 123.
- a class function called howManyLists that returns the number of objects of type WishList that were created and not destroyed yet.
Your class should allow move operations, and NOT allow copy operations.
Modify the main function provided below:
- to use a smart pointer
to handle any exception that is generated by the class.
Do not change this function for any other purposes.
See the main function and the expected output for hints on how the class is used, prototype for the functions and how to format your output.
Add any special member functions/private members as your design requires. For full marks, your program must be complete, compilable and correct under C++17 standard.
Bonus: (5 points) re-write the printId and printName functions to use members from class std::string instead of loops. This bonus is provided only if both re-written functions are complete and correct.
Provided Code
// Prints the SKU component of the `gift`. // Assumes `gift` represents a valid gift. void printId(const std::string& gift) { for (auto i = 0; gift[i] != '#'; ++i) std::cout << gift[i]; std::cout << std::endl; } // Prints the NAME component of the `gift`. // Assumes `gift` represents a valid gift. void printName(const std::string& gift) { size_t idx{}; for (auto i = 0; gift[i] != '#'; ++i) ++idx; // skip the "##" for (auto i = idx + 2; i < gift.size(); ++i) std::cout << gift[i]; std::cout << std::endl; } int main() { std::string gifts[]{ "ABC123##Scarf", "AA12##iPhone", "invalid_gift", // missing ## "3245HOHOHO##Coffee Maker", "ID#Invalid_Gift", // missing ## "EFG##Gaming Headset", "987654##Some Book" }; WishList* pLst = new WishList(); (*pLst += gifts[0]) += gifts[1]; std::cout << "---- "; std::cout << "1. Objects alive: " << WishList::howManyLists() << " "; std::cout << "---- "; for (const auto& gift : gifts) { *pLst += gift; } { WishList another(std::move(*pLst)); another.display(printId); std::cout << " ---- "; std::cout << "2. Objects alive: " << WishList::howManyLists() << " "; std::cout << "---- "; another.display(printName); } std::cout << " ---- "; std::cout << "3. Objects alive: " << WishList::howManyLists() << " "; std::cout << "---- "; pLst->display(printId); pLst->display(printName); delete pLst; }
Sample Output
---- 1. Objects alive: 1 ---- String [invalid_gift] doesn't represent a valid gift. String [ID#Invalid_Gift] doesn't represent a valid gift. ABC123 AA12 3245HOHOHO EFG 987654 ---- 2. Objects alive: 2 ---- Scarf iPhone Coffee Maker Gaming Headset Some Book ---- 3. Objects alive: 1 ---- Empty list! Empty list!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
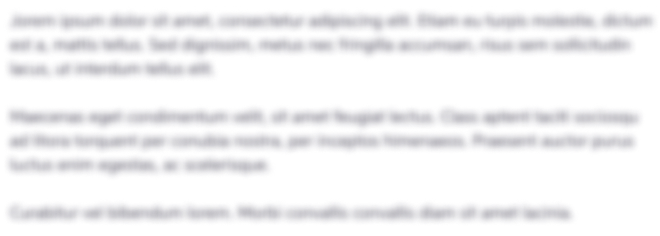
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started