Question
Need code (java) that allows the user to choose the color of the snake (red, green, blue). The two classes that are part of the
Need code (java) that allows the user to choose the color of the snake (red, green, blue). The two classes that are part of the game are listed below. Please highlight any changes made. Thanks!
Class 1:
import java.awt.*; import java.awt.event.*; import java.util.ArrayList; import javax.swing.*;
public class SnakeGame extends JPanel implements KeyListener, ActionListener {
// DECLARE ALL INSTANCE VARIABLES HERE.. private Snake snake; private Rectangle nibble; private int countFrames;// timer private String title = "Blind Snake - " + "CONTROLS - (ARROWS-MOVE)(R TO RESTART)"; private Font font = new Font("Helvetica",Font.BOLD,20);//Font size and type public static final Rectangle border = new Rectangle(0,0,610,610);//size of JPanel private Timer clock;// screen private Image offScreenBuffer;// graphics double buffer private Graphics offScreenGraphics;// graphics double buffer /** * main method needed for initialize the game window * .. THIS METHOD SHOULD NOT BE MODIFIED! .. */ public static void main(String[] args){ JFrame window = new JFrame("Snake Game Clone"); window.setBounds(100, 100, border.width + 7, border.height + 27);//setting boundaries window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); window.setResizable(false);
SnakeGame game = new SnakeGame(); game.setBackground(Color.BLACK); window.getContentPane().add(game); window.setVisible(true); game.init(); window.addKeyListener(game); }
/** * init() is needed to override the init in JApplet. * THIS METHOD SHOULD NOT BE MODIFIED! .. * you should write all necessary initialization code in initRound() */ public void init() { System.out.println(getWidth() + " , " + getHeight()); offScreenBuffer = createImage(getWidth(), getHeight()); //creating screen offScreenGraphics = offScreenBuffer.getGraphics(); initRound(); clock = new Timer(100, this); // 100 millisecond game speed //You can change the game speed by modifying the 100! That would make it too hard or easy though. // ............................and invokes method actionPerformed()
// INITIALIZE ALL SOUNDS AND IMAGE VARIABLES HERE... }
/** * initializes all fields needed for each round of play (i.e. restart) */ public void initRound() { countFrames = 0; // YOUR CODE GOES HERE.. // initialize game Objects like Snake snake = new Snake(); nibble = new Rectangle(20 * ((int)(Math.random()*30)), 20 * ((int)(Math.random()*30)), 20, 20); }
/** * Called automatically after a repaint request * .. THIS METHOD SHOULD NOT BE MODIFIED! .. * you should write all necessary rendering code in method draw() */ public void paint(Graphics g) { draw((Graphics2D) offScreenGraphics); g.drawImage(offScreenBuffer, 0, 0, this); }
/** * renders all objects to Graphics g (i.e. the window) */ public void draw(Graphics2D g) { super.paint(g);// refresh the background g.setFont(font); g.drawString(title, 0, 20);// draw the title in top left g.drawString("TIMER: " + (int)(countFrames/10),0, 70);// under title g.drawString("SCORE: " + snake.getcountSize(),0, 120); //under timer
// YOUR CODE GOES HERE.. // render all game objects here snake.draw(g); g.fill(snake.getHead()); for (Rectangle bodySegment : snake.getBody()) { g.fill(bodySegment); } g.fill(nibble); } public void nibbleRandomizer() { nibble.setLocation(20 * ((int)(Math.random()*30)), 20 * ((int)(Math.random()*30))); }
/** * Called automatically when the timer fires * this is where all the game Objects will be updated */ public void actionPerformed(ActionEvent e) {
snake.move(); // YOUR CODE GOES HERE.. if (snake.isDead()) { clock.stop(); } if (nibble.intersects(snake.getHead())) { snake.grow(); nibbleRandomizer(); } countFrames++;// timer repaint();// needed to refresh screen } public void keyPressed(KeyEvent e)// direction change with arrows { int keyCode = e.getKeyCode(); if (keyCode == KeyEvent.VK_UP) { snake.setDirection(8); } else if (keyCode == KeyEvent.VK_LEFT) { snake.setDirection(4); } else if (keyCode == KeyEvent.VK_DOWN) { snake.setDirection(2); } else if (keyCode == KeyEvent.VK_RIGHT) { snake.setDirection(6); } } /** * handles any key released events and restarts the game if * the Timer is not running and user types 'r' */ public void keyReleased(KeyEvent e) { int keyCode = e.getKeyCode(); if (keyCode == KeyEvent.VK_R && !clock.isRunning()) { clock.start(); initRound(); } } /** * leave empty.. needed for implementing interface KeyListener */ public void keyTyped(KeyEvent e) { }
}//end class SnakeGame
Class 2:
import java.awt.*; import java.awt.event.*; import java.util.ArrayList; import javax.swing.*;
public class Snake { private int size, direction, bodyAdd, countSize; private Rectangle head; private ArrayListbody; private ArrayListdirectionQ; public Snake() { size = 20; direction = 8; bodyAdd = 4; countSize = 4; head = new Rectangle(300, 300, 20, 20); body = new ArrayList(); directionQ = new ArrayList(); } public void draw(Graphics2D g) { g.draw(head); for (Rectangle bodySegment : body) { g.draw(bodySegment); } } public boolean isDead() { if (head.x < 0 || head.x > 580 || head.y < 0 || head.y > 580) { return true; } for (Rectangle segment : body) { if (head.intersects(segment)) { return true; } } return false; } public int getDirection() { return direction; } public int getcountSize() { return countSize; } public ArrayList getBody() { return body; } public Rectangle getHead() { return head; } public int getbodyAdd() { return bodyAdd; } public void grow() { bodyAdd += 1; } public void setDirection(int d) { directionQ.add(d); } public void move() { if (directionQ.isEmpty() == false) { direction = directionQ.get(0); directionQ.remove(0); } body.add(0, new Rectangle(head)); if (direction == 4) { head.x -= size; } else if (direction == 6) { head.x += size; } else if (direction == 8) { head.y -= size; } else if (direction == 2) { head.y += size; } if (bodyAdd >= 0) { bodyAdd--; } else if (body.size() > 0) { body.remove(body.size()-1); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
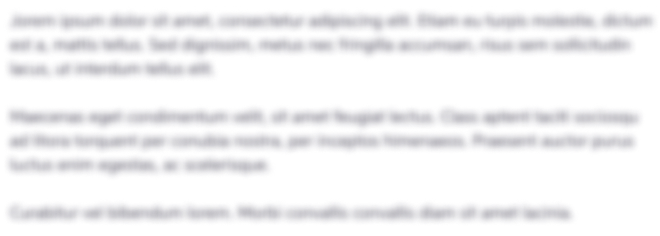
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started