Question
Need Help!! C++ #include #includelinkedlist.h using namespace std; /** * @brief Destructor to destroy all nodes and release memory */ LinkedList::~LinkedList() { //TODO: Add code
Need Help!! C++
#include
using namespace std;
/** * @brief Destructor to destroy all nodes and release memory */ LinkedList::~LinkedList() { //TODO: Add code here. Make sure memory is released properly. }
/** * @brief Purpose: Checks if the list is empty * @return true if the list is empty, false otherwise */ bool LinkedList::isEmpty() const { // TODO: Add code here return count == 0; }
/** * @brief Get the number of nodes in the list * @return int The number of nodes in the list */ int LinkedList::length() const{ //TODO: Add code here }
/** * @brief Convert the list to a string * */ string LinkedList::toString() { string str = "["; Node *ptr = front; if (ptr != nullptr) { // Head node is not preceded by separator str += to_string(ptr->val); ptr = ptr->next; } while (ptr != nullptr) { str += ", " + to_string(ptr->val); ptr = ptr->next; } str += "]"; return str; }
/** * @brief Displays the contents of the list */ void LinkedList::displayAll() { cout << toString() << endl; }
//TODO: Add comments void LinkedList::addRear(T val) { // TODO: Add code here // consider the two cases of whether the list was empty }
//TODO: Add comments void LinkedList::addFront(T val) { // TODO: Add code here // consider the two cases of whether the list was empty }
//TODO: Add comments bool LinkedList::deleteFront(T &OldNum) { // TODO: Add code here // consider if the list was empty and return false if the list is empty // consider the special case of deleting the only node in the list }
//TODO: Add comments bool LinkedList::deleteRear(T &OldNum) { // TODO: Add code here // consider if the list was empty and return false if the list is empty // consider the special case of deleting the only node in the list }
/* --- harder ones for test 2 and 3 -- */
/** * @brief Delete a node at a given position from the list. The * node at position pos is deleted and the value of the deleted node is returned in val. * The valid range of pos is 1 to count. pos = 1 is the first node, and pos = count is the last node. * @param pos: position of the node to be deleted * @param val: it is set to the value of the node to be deleted * @return true: if the node was deleted successfully * @return false: if the node was not deleted successfully because the position was out of range */ bool LinkedList::deleteAt(int pos, T &val) { //TODO: Add code here // check if the pos is valid first, then move the ptr to the rigth positon // consider the special case of deleting the first node and the last node // Do not forget to set value. }
/** * @brief Insert a value at a specified position in the list. The valid pos is in the range of 1 to count+1. * The value will be inserted before the node at the specified position. if pos = 1, the value will be inserted * at the front of the list. if pos = count+1, the value will be inserted at the rear of the list. * @param pos: position to insert the value at. * @param val: value to insert. * @return true: if the value was inserted. * @return false: if the value was not inserted because pos is out of the range. */ bool LinkedList::insertAt(int pos, T val) { //TODO: Add code here // check if the pos is valid first, then move the ptr to the rigth positon // consider the special case of inserting the first node and the last node }
/** * @brief Copy Constructor to allow pass by value and return by value of a LinkedList * @param other LinkedList to be copied */ LinkedList::LinkedList(const LinkedList &other) { // Start with an empty list front = nullptr; rear = nullptr; count = 0; // TODO: Add code here. Interate through the other list and add a new node to this list // for each node in the other list. The new node should have the same value as the other node.
}
/** * @brief Overloading of = (returns a reference to a LinkedList) * @param other LinkedList to be copied * @return reference to a LinkedList */ LinkedList &LinkedList::operator=(const LinkedList &other) { if(this != &other) { // check if the same object // Delete all nodes in this list // TODO: Add code here // Interate through the other list and add a new node to this list // Be sure to set the front and rear pointers to the correct values // Be sure to set the count to the correct value // TODO: Add code here } return *this; }
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
LinkedList.h //INSTRUCTION: //Linkedlist class - header file template //You must complete the TODO parts and then complete LinkedList.cpp. Delete "TODO" after you are done. //You should always comments to each function to describe its PURPOSE and PARAMETERS #pragma once
// ======================================================= // Your name: ??? (TODO: Add your name) // Compiler: g++ // File type: headher file linkedlist.h //======================================================= #include
// Datatype T : element type definition typedef int T; // int for now but can change later
//a list node is defined here as a struct Node for now struct Node { T val; // stored value Node *next; // pointer to the next node
// Constructor Node(T val = 0, Node *next = nullptr) { this->val = val; this->next = next; } }; //---------------------------------------------------------
class LinkedList { private: Node *front; // pointer to the front node Node *rear; // pointer to the rear node int count; // the number of nodes in the list
public: LinkedList() { // constructor to create an empty list front = nullptr; rear = nullptr; count = 0; }
~LinkedList(); // destructor to destroy all nodes and release memory
/** * @brief Copy Constructor to allow pass by value and return by value of a LinkedList * @param other LinkedList to be copied */ LinkedList(const LinkedList &other);
/** * @brief Overloading of = (returns a reference to a LinkedList) * @param other LinkedList to be copied * @return reference to a LinkedList */ LinkedList &operator=(const LinkedList &other);
/** * @brief Purpose: Checks if the list is empty * @return true if the list is empty, false otherwise */ bool isEmpty() const;
/** * @brief Get the number of nodes in the list * @return int The number of nodes in the list */ int length() const;
/** * @brief Convert the contents of the list to a string */ string toString();
/** * @brief Displays the contents of the list * */ void displayAll();
//TODO: Add comments void addFront(T val);
//TODO: Add comments void addRear(T val);
//TODO: Add comments bool deleteFront(T &val);
//TODO: Add comments bool deleteRear(T &val);
/** * @brief Delete a node at a given position from the list. The * node at position pos is deleted and the value of the deleted node is returned in val. * The valid range of pos is 0 to count-1. pos = 0 for the first node, and pos = count-1 for the last node. * @param pos: position of the node to be deleted * @param val: it is set to the value of the node to be deleted * @return true: if the node was deleted successfully * @return false: if the node was not deleted successfully because the position was out of range */ bool deleteAt(int pos, T &val);
/** * @brief Insert a value at a specified position in the list. The valid pos is in the range of 0 to count. * The value will be inserted before the node at the specified position. if pos = 0, the value will be inserted * at the front of the list. if pos = count, the value will be inserted at the rear of the list. * @param pos: position to insert the value at. * @param val: value to insert. * @return true: if the value was inserted. * @return false: if the value was not inserted because pos is out of the range. */ bool insertAt(int pos, T val); };
Step by Step Solution
There are 3 Steps involved in it
Step: 1
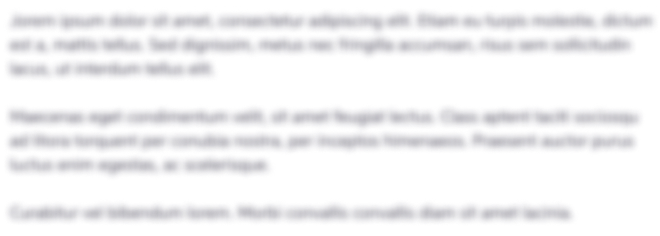
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started