Question
Need help coding this List. Lists are a lot like arrays, but youll be using get, set, add, size and the like instead of the
Need help coding this List. Lists are a lot like arrays, but youll be using get, set, add, size and the like instead of the array index operators [].
package list.exercises; import java.util.List; public class ListExercises {
/**
* Counts the number of characters in total across all strings in the supplied list;
* in other words, the sum of the lengths of the all the strings.
* @param l a non-null list of strings
* @return the number of characters
*/
public static int countCharacters(List
return 0; }
/**
* Splits a string into words and returns a list of the words.
* If the string is empty, split returns a list containing an empty string.
*
* @param s a non-null string of zero or more words
* @return a list of words
*/
public static List
/**
* Returns a copy of the list of strings where each string has been
* uppercased (as by String.toUpperCase).
*
* The original list is unchanged.
*
* @param l a non-null list of strings
* @return a list of uppercased strings
*/
public static List
return null;
}
/**
* Returns true if and only if each string in the supplied list of strings
* starts with an uppercase letter. If the list is empty, returns false.
*
* @param l a non-null list of strings
* @return true iff each string starts with an uppercase letter
*/
public static boolean allCapitalizedWords(List
return false;
}
/**
* Returns a list of strings selected from a supplied list, which contain the character c.
*
* The returned list is in the same order as the original list, but it omits all strings
* that do not contain c.
*
* The original list is unmodified.
*
* @param l a non-null list of strings
* @param c the character to filter on
* @return a list of strings containing the character c, selected from l
*/
public static List
return null;
}
/**
* Inserts a string into a sorted list of strings, maintaining the sorted property of the list.
*
* @param s the string to insert
* @param l a non-null, sorted list of strings
*/
public static void insertInOrder(String s, List
}
}
ListExercisesTest .java
package list.exercises;
import static org.junit.Assert.*; import java.util.ArrayList; import java.util.Arrays; import java.util.List;
import org.junit.Rule; import org.junit.Test; import org.junit.rules.Timeout;
public class ListExercisesTest {
// Uncomment these two lines if you want to catch infinite loops // @Rule // public Timeout globalTimeout = Timeout.seconds(10); // 10 seconds
@Test public void testCountCharactersEmpty() { assertEquals(0, ListExercises.countCharacters(new ArrayList
@Test public void testCountCharactersEmptyStrings() { assertEquals(0, ListExercises.countCharacters(Arrays.asList("", "", ""))); } @Test public void testCountCharactersOneString() { assertEquals(9, ListExercises.countCharacters(Arrays.asList("asdf jkl;"))); }
@Test public void testCountCharactersThreeStrings() { assertEquals(15, ListExercises.countCharacters(Arrays.asList("asdf jkl;", "", "123456"))); } @Test public void testSplitZero() { assertEquals(Arrays.asList(""), ListExercises.split("")); }
@Test public void testSplitOne() { assertEquals(Arrays.asList("banana"), ListExercises.split("banana")); }
@Test public void testSplitThree() { assertEquals(Arrays.asList("Larry", "Moe", "Curly"), ListExercises.split("Larry Moe Curly ")); }
@Test public void testUppercasedEmptyList() { assertEquals(Arrays.asList(), ListExercises.uppercased(Arrays.asList())); } @Test public void testUppercasedEmpty() { assertEquals(Arrays.asList(""), ListExercises.uppercased(Arrays.asList(""))); } @Test public void testUppercasedList() { assertEquals(Arrays.asList("ASDF", "JKL;", "!@#$"), ListExercises.uppercased(Arrays.asList("asdf", "jkl;", "!@#$"))); }
@Test public void testUppercasedParameterUnchanged() { List
@Test public void testAllCapitalizedWordsEmptyString() { assertFalse(ListExercises.allCapitalizedWords(Arrays.asList(""))); }
@Test public void testAllCapitalizedWordsThreeStringsFalse1() { assertFalse(ListExercises.allCapitalizedWords(Arrays.asList("Asdf", "Jkl;", "qwer"))); }
@Test public void testAllCapitalizedWordsThreeStringsFalse2() { assertFalse(ListExercises.allCapitalizedWords(Arrays.asList("Asdf", "Jkl;", "!@#$"))); }
@Test public void testAllCapitalizedWordsThreeStringsFalse3() { assertFalse(ListExercises.allCapitalizedWords(Arrays.asList("Asdf", "", "Jkl;"))); }
@Test public void testAllCapitalizedWordsOneStringTrue() { assertTrue(ListExercises.allCapitalizedWords(Arrays.asList("Asdf"))); }
@Test public void testAllCapitalizedWordsThreeStringsTrue() { assertTrue(ListExercises.allCapitalizedWords(Arrays.asList("Asdf", "Jkl;", "Qwer"))); }
@Test public void testFilterContainingEmptyList() { assertEquals(Arrays.asList(), ListExercises.filterContaining(Arrays.asList(), 'a')); }
@Test public void testFilterContainingEmptyStrings() { assertEquals(Arrays.asList(), ListExercises.filterContaining(Arrays.asList("", ""), 'b')); } @Test public void testFilterContainingEmptyAndMatchingStrings() { assertEquals(Arrays.asList("Marc"), ListExercises.filterContaining(Arrays.asList("Marc", "", "banana"), 'c')); }
@Test public void testFilterContainingMultipleMatchingStrings() { assertEquals(Arrays.asList("David", "Ted", "Bundy"), ListExercises.filterContaining(Arrays.asList("Marc", "David", "Ted", "Bundy"), 'd')); } @Test public void testFilterContainingParameterUnchanged() { List
@Test public void testInsertInOrderFirst() { List
@Test public void testInsertInOrderMiddle() { List
Step by Step Solution
There are 3 Steps involved in it
Step: 1
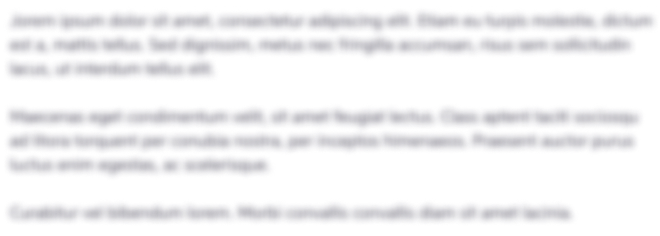
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started