Question
Need help creating a calendar/drawing in java. Here are the instructions: Refer to this page on how to incorporate the DrawingPanel class. Ch 3G DrawingPanel
Need help creating a calendar/drawing in java.
Here are the instructions:
- Refer to this page on how to incorporate the DrawingPanel class. Ch 3G DrawingPanel Java code
- Create a DrawingPanel and Graphics object in main() as shown in the book.
- Comment out the loops & calls to printMonth() but do not delete it. Create a new drawMonth() routine to call instead of printMonth() which takes a year (2018), month (1), and Graphics object.
public static void drawMonth(int iYear, int iMonth, Graphics g)
- Look at examples in the book on how to set the font and colors. Setting the color will affect all future text and graphics until you change it again.
- When you draw the month header, you may wish to use the following code to obtain the width of the string s, Graphics g. You could hard code the width, but it won't look right for other months. Note that you must call this after the font is set.
int width = g.getFontMetrics().stringWidth(s)
- When you draw the days of the week header, the simplest way is to use the same text string you did for printMonth() and adjust the spacing as needed to fit your grid. Play with font sizing to match your grid.
- It may simplify your code if you have variables that points to the upper left xOrigin,yOrigin of your calendar, and variables that hold the widthDay & heightDay. Then you can draw at X=xOrigin + * widthDay, Y=yOrigin + * heightDay.
- You should only need one nested FOR loop to draw the grid. The outer loop should control the 6 weeks (header + 5 weeks). The inner loop should control the 7 days of the week.
- You should only need one simple FOR loop to draw the days of the month similar to printMonth(). However we can no longer just use print() and println() - we need to calculate X/Y. The same expression ((day-1)% 7) works to figure out the column offset. The expression (day / 7) calculates the row offset. But remember that graphics are 0-based so subtract one. For example in April 2018
- Day 1 is drawn at X = + *((1-1)%7) , Y = + *(1/7)
- Day 8 is drawn at X = + *((8-1)%7) , Y = + *(8/7)
- Day 17 is drawn at X = + *((17-1)%7) , Y = + *(17/7)
-
For the top graphics, if you want to incorporate randomness you may want to use the following code which generates a random number from 1 to x. Please put it into its own method and name it intelligently. Consider if you can make other subroutines of repeated graphics functions.
(int)(Math.random()*x)+1
Here is my code: import java.time.*; import java.awt.*; import java.util.*;
public class Calendars {
public static void main(String args[]){ //set drawing panel DrawingPanel calendar = new DrawingPanel(640, 480); //get graphics Graphics g = calendar.getGraphics(); //call drawing drawMonth(480,640, g);
//OLD WORK LINES: (13 - 24) //System.out.println("Enter the # of (month year)"); //prints month of April 2018 //printMonth(2018, 4); //Get month + year //int year, month; //Scanner sc= new Scanner(System.in); //month = sc.nextInt(); //year = sc.nextInt(); //calls year and month //for (int month = 1; month <= 12; month ++) { //printMonth(year, month); } public static void drawMonth(int width, int height, Graphics g) {
//set font g.setFont(new Font("Arial", Font.BOLD, 20)); //string to write month String s = "Month of January 2018"; //string to write days of week String dayhead = " Sun Mon Tue Wed Thu Fri Sat"; //sets width for all font days int w = g.getFontMetrics().stringWidth(s); //draws string g.drawString(s, (width-w)/2, 20); g.drawString(dayhead, (width-w)/2, 20); //sets calendar origin coordinates int xOrigin = 130; int yOrigin = 200; //calculates width and height int dayWidth = (width-20)/7; int dayHeight = (height-20)/7;
//set color g.setColor(Color.BLACK); for (int week = 1; week <= 6; week ++) { for (int day = 1; day <= 7; day++) { g.drawRect(xOrigin+dayWidth*(day-1), yOrigin+dayHeight*(week-1), dayWidth, dayHeight); } }
yOrigin += 200;
for (int day = 1; day <= 30; day++) { // draw day using day/7 for vertical, day % 7 for horizontal g.drawString("" + day, xOrigin + ((day-1) % 7)*dayWidth, yOrigin + (day-1) / 7); } }
/** * Input: month and year * * Output: calendar based on month and year * @param iMonth - month of year */ public static void printMonth(int iYear, int iMonth) { //Print Month of string String monthTitle = "Month of " + Month.of(iMonth).name() + " " + iYear;
//Print month title System.out.println(centerString(monthTitle, " Sun Mon Tue Wed Thu Fri Sat".length())); //Print days of week subtitle System.out.println(" Sun Mon Tue Wed Thu Fri Sat");
//prints blank days until first day int firstDay = firstDayOfMonth(iYear, iMonth); for (int day = 1; day < firstDay; day++) { System.out.print(" ");
} //print day numbers for (int day = 1; day <= daysInMonth(iYear, iMonth); day++) { System.out.print(centerString(padded(day,2),7)); //add start day for correct break if ((day + firstDay - 1) % 7 == 0) System.out.println(); } System.out.println(); }
private static int dayOfWeek(int i, int j, int k) { // TODO Auto-generated method stub return 0; }
/** *Input: space #'s for padding * *Output: padded spaces on both sides of "hello" * Example: * centerString("hello",10) returns " hello " * centerString("hello",11) returns " hello " * * Arguments: * @param s - the string variable in the center * @param n - number of spaces padded * @return padded string output */ public static String centerString(String s, int number) { //insert padding to center string int len = s.length();
for (int spaces = 1; spaces <= (number - len)/2 + (number - len)%2; spaces++) { s = " " + s; } for (int spaces = 1; spaces <= (number - len)/2; spaces++) { s = s + " "; }
return s; }
// DO NOT MODIFY ANYTHING BELOW THIS LINE. PUT ALL YOUR CODE ABOVE IT
/** * Returns the number of days in a month * Example: daysInMonth(2018,1) => 31 daysInMonth(2018,2) => 28
Imports: You must place this line at the top of your .JAVA file: import java.time.*; * @param iYear - day of the week with Sun = 1, Mon = 2... Sat = 7 * @param iMonth - month of the year with 1 = Jan... 12 = Dec * @return the count of the days in the month (28-31) */ public static int daysInMonth(int iYear, int iMonth) { // DO NOT MODIFY THIS CODE GregorianCalendar cal = new GregorianCalendar(iYear, iMonth-1, 1); return (cal.getActualMaximum(Calendar.DAY_OF_MONTH)); }
/** * Returns the start day of a month * Example: firstDayOfMonth(2018,1) => 2 (1/1/18 is a Monday) firstDayOfMonth(2018,2) => 5 (2/1/18 is a Thurs)
* @param iYear - full year in the form 2018 * @param iMonth - month of the year with 1 = Jan... 12 = Dec * @return day of the week with Sun = 1, Mon = 2... Sat = 7 */ public static int firstDayOfMonth(int iYear, int iMonth) { // DO NOT MODIFY THIS CODE Calendar cal = new GregorianCalendar(iYear, iMonth-1, 1); return(cal.get(Calendar.DAY_OF_WEEK)); }
/** * left pads a number with spaces up to a larger size
Example: padded(1,2) => " 1" padded(20,5) => " 20"
* @param n - number to be padded * @param width - the desired width, assumed to be larger than n's string * @return spaces followed by the number up to size n */ public static String padded(int n, int width) { // DO NOT MODIFY THIS CODE String s = "" + n; for (int i = s.length(); i < width; i++) { s = " " + s; } return s; }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
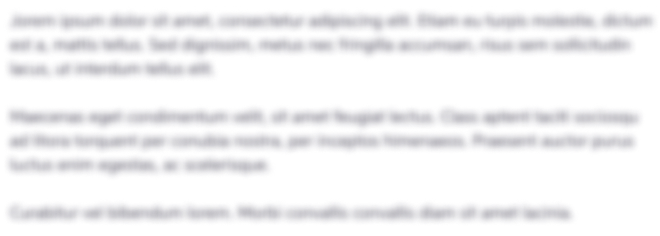
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started