Question
Need help finish coding java 2d array method. There are 4 codes that I need help with, all of the other java code methods has
Need help finish coding java 2d array method. There are 4 codes that I need help with, all of the other java code methods has been completed. Please help code the following 4 codes. I have listed what I already have
-print the row of grades
-determine and print average
-print each assignment average
-print the average of the assignment averages
Basic concept of this JAVA assignment:
Print the headers for the report, a row for each student by student ID (which is just 1 through 4) in which you will list each grade in the gradebook and that student's average. Then you will print a line that contains the average grade for each assignment. You will end that line with the average of the assignment averages, which happens to also be equal to the average of all the student averages.
Code currently displays:
Need Final code to display this:
This is the code I have so far, see below:
import java.util.Scanner;
public class Program00
{
static int actualNumberStudents = 4;
static int actualNumberAssignments = 3;
static int[][] grades = {{90,90,90},
{ 0,90,91},
{57,89,78},
{60,70,80}};
public static double calculateAssignmentAverage(int assignmentIndex)
{
// This method will return the average of all the grades for the given assignmentIndex.
if (actualNumberStudents == 0) return -1.0;
}
private static void printStudentID(int rowIndex)
{
System.out.printf("%2d. ", rowIndex + 1);
}
private static void printRow(int rowIndex)
{
int sumGrades = 0;
int grade = 0;
// print the Student ID fot the row, which is rowIndex + 1
printStudentID(rowIndex);
// print the row of grades
//??Need help with print the row of grades code
// determine and print average
//??Need help with print average code
//Leave the println statement unless you incorporate the end of line into the method elsewhere
System.out.println();
}
private static void printHeader()
{
System.out.println();
// print # Name
System.out.print(" # ");
// print assignment numbers
for (int j = 0; j
{
System.out.printf("%3d ", j + 1);
}
System.out.println(" Avg");
}
private static void printAssignmentAvgRow()
{
// Print the start of the line
System.out.print("Avg ");
// print each assignment average
//??Need help with printing each assignment average code
// print the average of the assignment averages
//??Need help with printing average of the assignment averages code,this is located at the end of the instructions
// print the end of line
System.out.println();
}
private static void printGradebook()
{
printHeader();
for (int i = 0; i
{
printRow(i);
}
printAssignmentAvgRow();
}
public static void main(String[] args)
{
printGradebook();
}
}
Instructions:
1. Call your class Program00, so your filename will be Program000.java. It is essential for grading purposes that everyone have the same class name.
2. Prepare to print each grade belonging to our current student which corresponds to rowIndex. Delete the "//??Need help" line where you see:
// print the row of grades
//??Need help with this code
3. Create code to make a for loop with j as the counter (if we are using counters, we usually use i for the row index which is first and j for the column index which is 2nd) and j
4. Test your program at this point and do not go on until it is working correctly.
5. Now we will get ready to print the student's average with one digit beyond the decimal (to the nearest tenth).
6. To do this, you will need to add a line in your for loop to keep track of the sum of the grades. Use the sumGrades variable for this.
7. After the for loop's closing brace and after the comment:
// determine and print average
//??Need help with this code
8. Check to see whether acutalNumberAssignments is zero. If so, you cannot calculate the student average because you can't divide by zero, so just print 5 dashes. Otherwise, declare the double variable average to be sumGrades / actualNumberAssignments; however, you'll need to cast sumGrades to double first or else you'll be doing integer division and it will throw away everything after the decimal place.
Then print the average. Use the %5.1f formatter (we need 3 because it could be up to 100 which is 3 digits, then one for the period and one for the place after the decimal; that all adds up to 5, and the .1 means one digit after the decimal). Add spaces to the beginning or end of your formatter to make things line up.
9. Test this and make sure it works before proceeding. Make sure that your header and rows line up nicely.
10. We still need to print the assignment averages in the printAssignmentAvgRow method:
Delete the "//??Need help" line after:
// print each assignment average
//??Need help with this code
11. Code a for loop that will print the assignment average under each of the lists of grades for that assignment. Use j as your index since you are doing it for each assignment, which means the column or 2nd index, and j
12. Test this, but remember that you have not finished the method calculateAssignmentAverage yet, so it will return -1.0 everywhere. Don't worry yet if it doesn't line up; the negative sign messes that up for now. Fix it later.
13. In the calculateAssignmentAverage method, notice that we are returning a double and we have an int parameter of the assignmentIndex.
14. Check to see whether actualNumberStudents is zero. If so, just return -1.0 . It is common to return -1 if you can't do a calculation and if -1 is not a valid result.
if (actualNumberStudents == 0)
return -1.0;
15. Now you will need to set an int variable sum to zero. Make a for loop with counter i (since this involves each student) with i
16. Test this and fix any alignment problems.
17. Print average of assignment averages instructions:
18. In the method printAssignmentAvgRow at the end of the line, print the average of the assignment averages. To do this, inside the for loop of this method, you will need to add assignmentAverage to an assignmentAverageSum variable of type double which you will have to declare above the for loop. After the for loop's closing brace and after:
// print the average of the assignment averages
//??Need help with this code
19. Calculate assignmentAverageSum / actualNumberAssignments. You won't have to cast anything because assignmentAverageSum is already double. Print this number using %5.1f formatter plus any spaces needed.
12. Test this. Make sure everything lines up. And you are finished.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
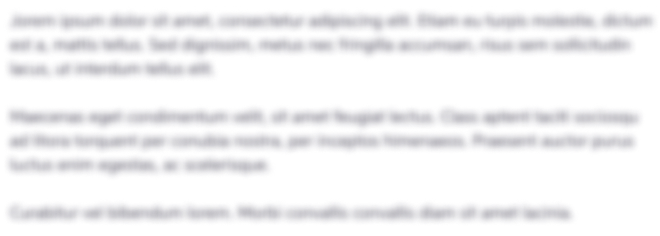
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started