Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Need help implementing a database.cs that will store the info for the code below and another database.cs that will perform operations Create, Read, Update, Delete
Need help implementing a database.cs that will store the info for the code below and another database.cs that will perform operations Create, Read, Update, Delete operations on an SQLite database. public class Category
public string Name get; set;
public Categorystring name
Name name;
public string GetName
return Name;
public class Expense
public int Id get; set;
public double Amount get; set;
public string Description get; set;
public Category Category get; set;
public DateTime Date get; set;
public Expenseint id double amount, string description, Category category, DateTime date
Id id;
Amount amount;
Description description;
Category category;
Date date;
public double GetAmount
return Amount;
public string GetDescription
return Description;
public Category GetCategory
return Category;
public DateTime GetDate
return Date;
using System;
using System.Collections.Generic;
public class ExpenseTracker
public List Expenses get; new List;
public void AddExpenseExpense expense
Expenses.Addexpense;
public void RemoveExpenseExpense expense
Expenses.Removeexpense;
public List GetExpensesByCategoryCategory category
return Expenses.FindAllexpense expense.Category category;
public double GetTotalSpending
double totalSpending ;
foreach var expense in Expenses
totalSpending expense.Amount;
return totalSpending;
public double GetSpendingByCategoryCategory category
double spendingByCategory ;
foreach var expense in Expenses
if expenseCategory category
spendingByCategory expense.Amount;
return spendingByCategory;
using System;
using System.Collections.Generic;
namespace ExpenseTrackerApp
class Program
static void Mainstring args
Create an instance of ExpenseTracker
ExpenseTracker expenseTracker new ExpenseTracker;
Add some sample expenses
expenseTrackerAddExpensenew Expense "Groceries", new CategoryFood DateTime.Now;
expenseTrackerAddExpensenew Expense "Movie tickets", new CategoryEntertainment DateTime.Now.AddDays;
expenseTrackerAddExpensenew Expense "Car repair", new CategoryTransportation DateTime.Now.AddDays;
Print the total spending
ConsoleWriteLineTotal spending: :C expenseTracker.GetTotalSpending;
Print expenses by category
ConsoleWriteLine
Expenses by category:";
foreach var category in expenseTracker.Expenses.Selecte eCategoryDistinct
ConsoleWriteLine$categoryGetName: expenseTrackerGetSpendingByCategorycategory:C;
Demonstrate other methods as needed eg addingremoving expenses, getting expenses by category
ConsoleReadLine; Keep the console open
public class User
public int Id get; set;
public string Name get; set;
public string Email get; set;
public Userint id string name, string email
Id id;
Name name;
Email email;
public int GetId
return Id;
public string GetName
return Name;
public string GetEmail
return Email;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
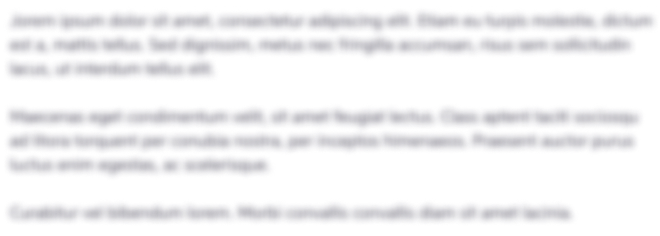
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started