Question
Need help implementing this Java class public class SquareMap extends GraphMap A square lattice where each cell has 4 neighbors set up in a checker
Need help implementing this Java class
public class SquareMap extends GraphMap
A square lattice where each cell has 4 neighbors set up in a checker board pattern.
Every Cell (the colored squares) in the SquareMap has an column and row index into a 2D array
holding the cells. In Fig. 5, the top left cell has the index (column, row) = (0, 0), and its neighbor
to the right has (1, 0), and so on. The neighbors for a cell at index (column, row) shall constitute
the following if they're within the map:
1. The cell at index (col-1, row)
2. The cell at index (col+1, row)
3. The cell at index (col, row-1)
4. The cell at index (col, row+1)
Depending on the cell's index in the 2D array, it can have as few as two neighbors and as many
as four. (Note: here we're describing cell indices using the pairs (horizontal, vertical) as in
mathematical coordinates. Remember when you access elements of the 2D array, you have
to reverse them, since for a 2D array the row index is first.)
In addition to its column and row index, each cell is associated with a location in pixels within a
graphical window. Pixels are measured from the upper left corner. The GraphMap class
includes an attribute distance representing the size in pixels of each Cell. When creating
Polygons for each cell, the coordinates in pixels shall follow the scheme shown in Fig. 5. There
shall be an empty border around the cells with width of distance/2. The createPolygon method
then shall return a square with width distance. The orange dot in Fig. 5 represents the point (0,
0) in pixels. For any point in pixels, the selectClosetIndex method shall return the cell's column
and row if the given (x, y) is within its square. For example, focus on the pink dot in Fig. 5:
Let's say that distance equals 10, and let's say that the pink dot belongs to the point (19,
21) in pixels. Then the closest column and row would be (1, 1). A point on the boundary
between cells is assumed to belong to the square to the right and/or below.
Finally, the width of the GraphMap in pixels shall equal the sum of the widths of the polygons
we need to draw in a row, plus the empty border on either side of the row. The height shall work
similarly.
Constructor Summary
Constructor and Description |
---|
SquareMap() |
Method Summary
Modifier and Type | Method and Description |
---|---|
Cell[] | createNeighbors(int col, int row) Create an array of neighbors for the cell with given column and row. |
java.awt.Polygon | createPolygon(int col, int row) Create a polygon for the cell with the given column and row. |
int | getPixelHeight() Gets the height of the window in pixels for rendering, including the border area. |
int | getPixelWidth() Gets the width of the window in pixels for rendering, including the border area. |
protected java.awt.Point | selectClosestIndex(int x, int y) Get the column and row indices for the cell closest to a given pixel (x, y) coordinate, returned as a Point object in which x is the column and y is the row. |
Methods inherited from class graph.GraphMap
clearAllDistances, createFood, getCells, getDistance, initialize, initialize, iterator,selectClosestCell, setColorGenerator, setCreateFood, setDistance, update, updateMouse
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface java.lang.Iterable
forEach, spliterator
Constructor Detail
SquareMap
public SquareMap()
Method Detail
getPixelWidth
public int getPixelWidth()
Description copied from class: GraphMap
Gets the width of the window in pixels for rendering, including the border area.
Specified by:
getPixelWidth in class GraphMap
Returns:
The width in pixels
getPixelHeight
public int getPixelHeight()
Description copied from class: GraphMap
Gets the height of the window in pixels for rendering, including the border area.
Specified by:
getPixelHeight in class GraphMap
Returns:
The height in pixels
createNeighbors
public Cell[] createNeighbors(int col, int row)
Description copied from class: GraphMap
Create an array of neighbors for the cell with given column and row.
Specified by:
createNeighbors in class GraphMap
Parameters:
col - The column index of a Cell
row - The row index of a Cell
Returns:
An array containing adjacent cells
selectClosestIndex
protected java.awt.Point selectClosestIndex(int x, int y)
Description copied from class: GraphMap
Get the column and row indices for the cell closest to a given pixel (x, y) coordinate, returned as a Point object in which x is the column and y is the row.
Specified by:
selectClosestIndex in class GraphMap
Parameters:
x - The x coordinate in pixels
y - The y coordinate in pixels
Returns:
column and row indices for the cell closest to the given (x, y)
createPolygon
public java.awt.Polygon createPolygon(int col, int row)
Description copied from class: GraphMap
Create a polygon for the cell with the given column and row.
Specified by:
createPolygon in class GraphMap
Parameters:
col - The column index of a Cell
row - The row index of a Cell
Returns:
A polygon with correct pixel coordinates for rendering the cell
Step by Step Solution
There are 3 Steps involved in it
Step: 1
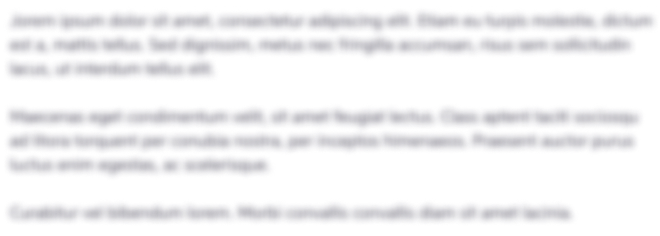
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started